argparse
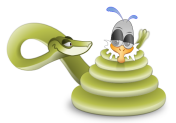
What is argparse?
"The argparse module makes it easy to write user-friendly command-line interfaces. The program defines what arguments it requires, and argparse will figure out how to parse those out of sys.argv. The argparse module also automatically generates help and usage messages and issues errors when users give the program invalid arguments." - from argparse - Parser for command-line options, arguments and sub-commands
The following description is from The argparse module is now part of the Python standard library!
The argparse module provides an easy, declarative interface for creating command line tools, which knows how to:
- parse the arguments and flags from sys.argv
- convert arg strings into objects for your program
- format and print informative help messages
and much more...
The argparse module improves on the standard library optparse module in a number of ways including:
- handling positional arguments
- supporting sub-commands
- allowing alternative option prefixes like + and /
- handling zero-or-more and one-or-more style arguments
- producing more informative usage messages
- providing a much simpler interface for custom types and actions
Let's look at our first sample of using argparse:
# arg.py import argparse import sys def check_arg(args=None): parser = argparse.ArgumentParser(description='Script to learn basic argparse') parser.add_argument('-H', '--host', help='host ip', required='True', default='localhost') parser.add_argument('-p', '--port', help='port of the web server', default='8080') parser.add_argument('-u', '--user', help='user name', default='root') results = parser.parse_args(args) return (results.host, results.port, results.user) if __name__ == '__main__': h, p, u = check_arg(sys.argv[1:]) print 'h =',h print 'p =',p print 'u =',u
If we run it:
$ python arg.py -H 192.17.23.5 h = 192.17.23.5 p = 8080 u = root
Note that the 'host' arg is set as 'required'. So, if we run the code without feeding host ip, we'll get an error like this:
$ python arg.py usage: arg.py [-h] -H HOST [-p PORT] [-u USER] arg.py: error: argument -H/--host is required
Also, we need to look at how the help works:
$ python arg.py -h usage: arg.py [-h] -H HOST [-p PORT] [-u USER] Script to learn basic argparse optional arguments: -h, --help show this help message and exit -H HOST, --host HOST host ip -p PORT, --port PORT port of the web server -u USER, --user USER user name
Notice that we used -H for host-ip mandatory option instead of lower case 'h' because it is reserved for 'help.
Another sample code:
# arg.py import argparse import sys def int_args(args=None): parser = argparse.ArgumentParser(description='Processing integers.') parser.add_argument('integers', metavar='N', type=int, nargs='+', help='integer args') return parser.parse_args() if __name__ == '__main__': print int_args(sys.argv[1:])
Just to see how it works, let's request 'help':
$ python arg.py -h usage: arg.py [-h] N [N ...] Processing integers. positional arguments: N integer args optional arguments: -h, --help show this help message and exit
We need to check the add_argument() method in ArgumentParser.add_argument():
ArgumentParser.add_argument(name or flags...[, action][, nargs][, const][, default][, type][, choices][, required][, help][, metavar][, dest])
- name or flags - Either a name or a list of option strings, e.g. foo or -f, --foo.
- action - The basic type of action to be taken when this argument is encountered at the command line.
- nargs - The number of command-line arguments that should be consumed.
- const - A constant value required by some action and nargs selections.
- default - The value produced if the argument is absent from the command line.
- type - The type to which the command-line argument should be converted.
- choices - A container of the allowable values for the argument.
- required - Whether or not the command-line option may be omitted (optionals only).
- help - A brief description of what the argument does.
- metavar - A name for the argument in usage messages.
- dest - The name of the attribute to be added to the object returned by parse_args().
Now, it's time to run the code:
$ arg.py 1 2 3 4 5 Namespace(integers=[1, 2, 3, 4, 5])
The '+' in nargs='+', just like '*', makes all command-line args present to be gathered into a list.
If we provide a wrong type arg such as a float type, we'll get an error:
$ arg.py 1.9999 2 3 4 5 usage: arg.py [-h] N [N ...] arg.py: error: argument N: invalid int value: '1.9999'
For details, check Einsteinish/GitHub-API
Python code:
import json import requests import time import calendar import argparse import sys import operator DAYS = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'] WEEK_IN_SECOND = 604800 def count_commits(repo, weeks, sort): # Get commit activity via Github REST API v3 with Python's requests module r = requests.get('https://api.github.com/repos/%s/stats/commit_activity' % repo) repos = json.loads(r.content) # sample : [{'days': [0, 0, 0, 0, 0, 0, 0], 'total': 0, 'week': 1511053200}, ... ] # calculate time cut in weeks current_epoctime = calendar.timegm(time.gmtime()) week_cut = current_epoctime-(weeks)*WEEK_IN_SECOND # initialize commits for the days of a week commits = [0]*7 # loop through commits week by week. Cut by input 'weeks' for r in repos: if r['week'] >= week_cut: for i,d in enumerate(r['days']): commits[i] += d # average commits per week commits = [c/weeks for c in commits] # construct dictionary from the two list : zip(DAYS, commits) # then, sort it (default: descending) days_commits = dict(zip(DAYS, commits)) days_commits = sorted(days_commits.items(), key = operator.itemgetter(1), reverse = (sort == 'dsc')) # sample : days_commits = [('Wednesday', 75.95), ('Thursday', 73.7), ... ] print('\n--- Commits (average) ---') for item in days_commits: print('%s %.1f' %(item[0],item[1])) print('\n--- The most commits ---') if sort == 'dsc': index = 0 # top else: index = 6 # bottom print('%s %.1f' %(days_commits[index][0],days_commits[index][1])) # setup args including default values and input error handling def check_arg(args=None): parser = argparse.ArgumentParser(description='Github API - stats of commits') #parser.add_argument('repo', metavar='repository name', type=str, help='user/repo') parser.add_argument('-r', nargs='?', default='kubernetes/kubernetes') parser.add_argument('-w', nargs='?', default='52') parser.add_argument('-s', nargs='?', default='dsc') results = parser.parse_args(args) return (results.r, results.w, results.s) # MAIN if __name__ == '__main__': ''' Count Github commits via 'stats/commit_activity' - using REST API v3 Usage : python ask_ki.py -r kubernetes/kubernetes -w=36 -s=dsc (note) All args are optional: '-r', '-w', and '-s' ''' r, w, s = check_arg(sys.argv[1:]) print('Inputs: repo=%s weeks=%s sort=%s' %(r,w,s)) # call github api count_commits(repo=r, weeks=int(w), sort=s)
Python tutorial
Python Home
Introduction
Running Python Programs (os, sys, import)
Modules and IDLE (Import, Reload, exec)
Object Types - Numbers, Strings, and None
Strings - Escape Sequence, Raw String, and Slicing
Strings - Methods
Formatting Strings - expressions and method calls
Files and os.path
Traversing directories recursively
Subprocess Module
Regular Expressions with Python
Regular Expressions Cheat Sheet
Object Types - Lists
Object Types - Dictionaries and Tuples
Functions def, *args, **kargs
Functions lambda
Built-in Functions
map, filter, and reduce
Decorators
List Comprehension
Sets (union/intersection) and itertools - Jaccard coefficient and shingling to check plagiarism
Hashing (Hash tables and hashlib)
Dictionary Comprehension with zip
The yield keyword
Generator Functions and Expressions
generator.send() method
Iterators
Classes and Instances (__init__, __call__, etc.)
if__name__ == '__main__'
argparse
Exceptions
@static method vs class method
Private attributes and private methods
bits, bytes, bitstring, and constBitStream
json.dump(s) and json.load(s)
Python Object Serialization - pickle and json
Python Object Serialization - yaml and json
Priority queue and heap queue data structure
Graph data structure
Dijkstra's shortest path algorithm
Prim's spanning tree algorithm
Closure
Functional programming in Python
Remote running a local file using ssh
SQLite 3 - A. Connecting to DB, create/drop table, and insert data into a table
SQLite 3 - B. Selecting, updating and deleting data
MongoDB with PyMongo I - Installing MongoDB ...
Python HTTP Web Services - urllib, httplib2
Web scraping with Selenium for checking domain availability
REST API : Http Requests for Humans with Flask
Blog app with Tornado
Multithreading ...
Python Network Programming I - Basic Server / Client : A Basics
Python Network Programming I - Basic Server / Client : B File Transfer
Python Network Programming II - Chat Server / Client
Python Network Programming III - Echo Server using socketserver network framework
Python Network Programming IV - Asynchronous Request Handling : ThreadingMixIn and ForkingMixIn
Python Coding Questions I
Python Coding Questions II
Python Coding Questions III
Python Coding Questions IV
Python Coding Questions V
Python Coding Questions VI
Python Coding Questions VII
Python Coding Questions VIII
Python Coding Questions IX
Python Coding Questions X
Image processing with Python image library Pillow
Python and C++ with SIP
PyDev with Eclipse
Matplotlib
Redis with Python
NumPy array basics A
NumPy Matrix and Linear Algebra
Pandas with NumPy and Matplotlib
Celluar Automata
Batch gradient descent algorithm
Longest Common Substring Algorithm
Python Unit Test - TDD using unittest.TestCase class
Simple tool - Google page ranking by keywords
Google App Hello World
Google App webapp2 and WSGI
Uploading Google App Hello World
Python 2 vs Python 3
virtualenv and virtualenvwrapper
Uploading a big file to AWS S3 using boto module
Scheduled stopping and starting an AWS instance
Cloudera CDH5 - Scheduled stopping and starting services
Removing Cloud Files - Rackspace API with curl and subprocess
Checking if a process is running/hanging and stop/run a scheduled task on Windows
Apache Spark 1.3 with PySpark (Spark Python API) Shell
Apache Spark 1.2 Streaming
bottle 0.12.7 - Fast and simple WSGI-micro framework for small web-applications ...
Flask app with Apache WSGI on Ubuntu14/CentOS7 ...
Fabric - streamlining the use of SSH for application deployment
Ansible Quick Preview - Setting up web servers with Nginx, configure enviroments, and deploy an App
Neural Networks with backpropagation for XOR using one hidden layer
NLP - NLTK (Natural Language Toolkit) ...
RabbitMQ(Message broker server) and Celery(Task queue) ...
OpenCV3 and Matplotlib ...
Simple tool - Concatenating slides using FFmpeg ...
iPython - Signal Processing with NumPy
iPython and Jupyter - Install Jupyter, iPython Notebook, drawing with Matplotlib, and publishing it to Github
iPython and Jupyter Notebook with Embedded D3.js
Downloading YouTube videos using youtube-dl embedded with Python
Machine Learning : scikit-learn ...
Django 1.6/1.8 Web Framework ...
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization