Qt5 Tutorial Main Window and Action - 2020
In this tutorial, we will learn how to setup the action from the menu and toolbar of the Main Window class.
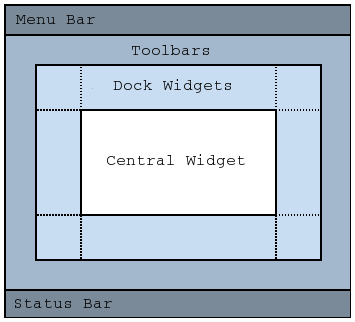
File->New File or Project...
Applications->Qt Gui Application->Choose...
We keep the class as MainWindow as given by default.
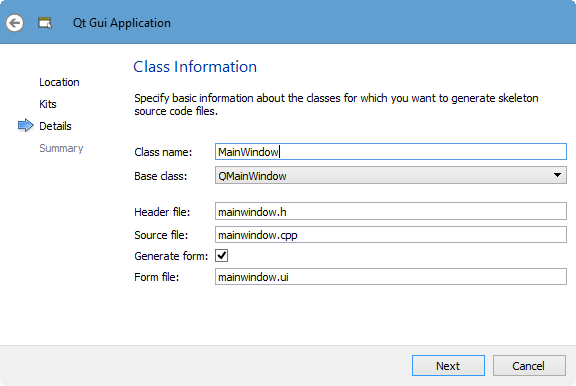
Hit Next.
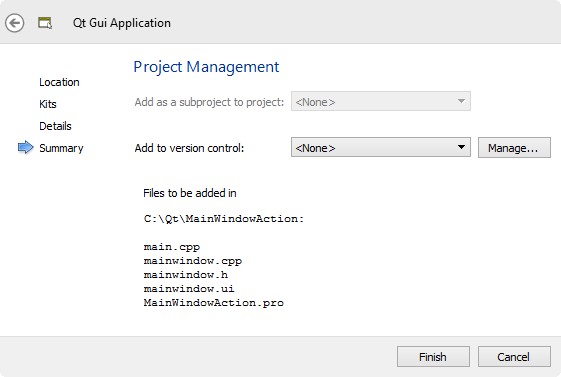
Hit Finish.
Here is the main.cpp:
#include "mainwindow.h" #include <QApplication> int main(int argc, char *argv[]) { QApplication a(argc, argv); MainWindow w; w.show(); return a.exec(); }
From the highlight line, we have an incident of the MainWindow class.
Here is the header file: mainwindow.h:
#ifndef MAINWINDOW_H #define MAINWINDOW_H #include <QMainWindow> namespace Ui { class MainWindow; } class MainWindow : public QMainWindow { Q_OBJECT public: explicit MainWindow(QWidget *parent = 0); ~MainWindow(); private: Ui::MainWindow *ui; }; #endif // MAINWINDOW_H
Note that we have a namespace UI, and our MainWindow is under the UI scope. Also, in the header, we declared a pointer to the UI::MainWindow as a private member, ui.
Let's open up Designer by double-clicking the mainwindow.ui to put menu bar and action. Type in "File" at the top menu, and "New Window..." under the "File" menu. We need to press Enter to make a real change in the menu. Notice that at the bottom, in the Action Editor, a new action called actionNew_Window has been created.
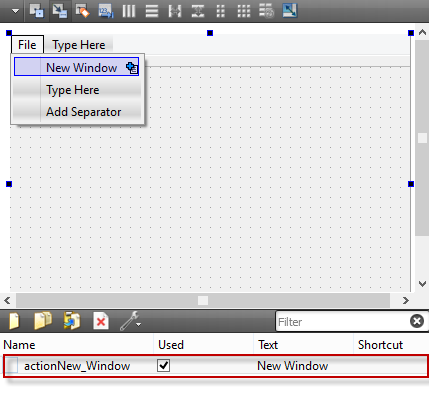
We can also drag the actionNew_Window action menu and drop in the toolbar:
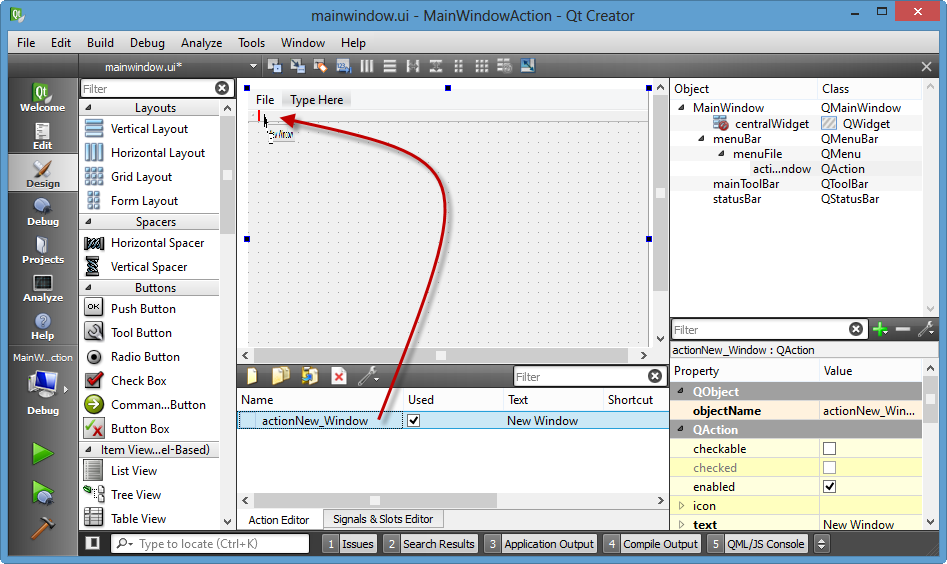
Now, we have two places to trigger actionNew_Window action.
Click the action on the Action Editor, and select "Go to Slot...".
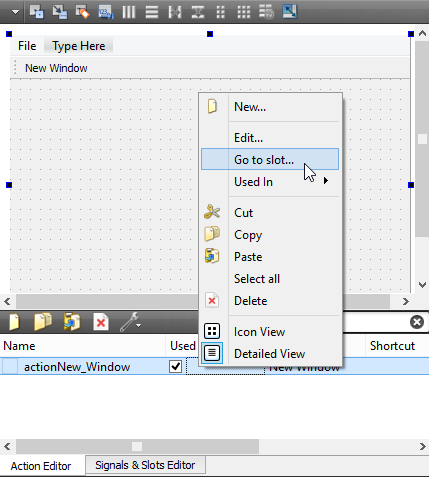
It allows us to trigger an event. Select triggered() and hit OK.
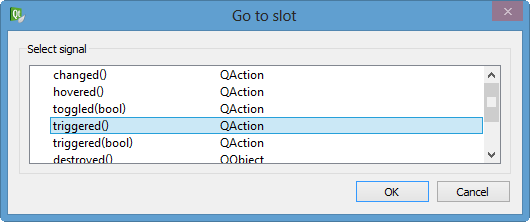
Then, Creator will show the changes in the code (mainwindow.cpp) automatically:
#include "mainwindow.h" #include "ui_mainwindow.h" MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent), ui(new Ui::MainWindow) { ui->setupUi(this); } MainWindow::~MainWindow() { delete ui; } void MainWindow::on_actionNew_Window_triggered() { }
So, whenever we click either toolbar item or menu item, this event will be triggered.
If we run the code, we get:
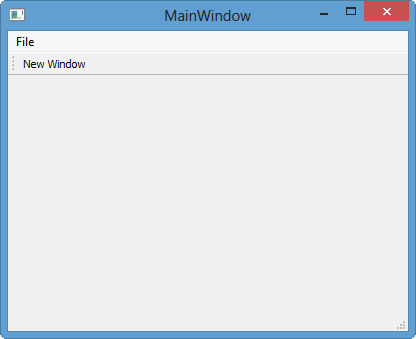
When we click the New Window, nothing happens. This is because we haven't put anything into the MainWindow::on_actionNew_Window_triggered() method.
Right click on MainWindowAction project->Add New->Qt->Qt Designer Form Class->Dialog without Buttons
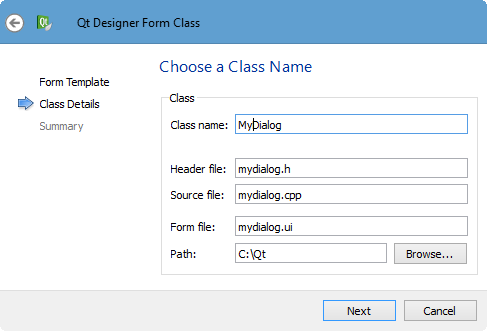
New files related to our new dialog have been created:
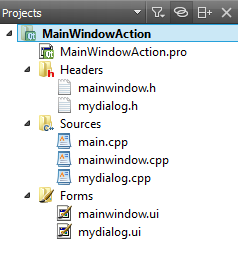
Let's put the proper code for the triggered event.
We'll open up the new dialog in two types: modal and modaless.
#include "mainwindow.h" #include "ui_mainwindow.h" #include "mydialog.h" MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent), ui(new Ui::MainWindow) { ui->setupUi(this); } MainWindow::~MainWindow() { delete ui; } void MainWindow::on_actionNew_Window_triggered() { MyDialog mDialog; mDialog.setModal(true); mDialog.exec(); }
If we run it, we get the modal type dialog:
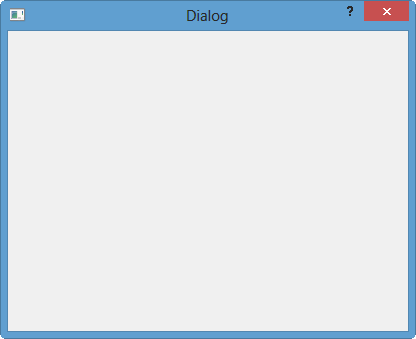
To get modeless, we editted the mainwindow.cpp as below:
void MainWindow::on_actionNew_Window_triggered() { MyDialog mDialog; /* mDialog.setModal(false); mDialog.exec(); */ mDialog.show(); }
But it disappears as soon as it is created. That's because unlike modal type dialog, modaless gives control to the main app, the stack variable goes away when it gets out of on_actionNew_Window_triggered(). So, we need to set a pointer as a member of the MainWindow class:
mainwindow.cpp:
#include "mainwindow.h" #include "ui_mainwindow.h" #include "mydialog.h" MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent), ui(new Ui::MainWindow) { ui->setupUi(this); } MainWindow::~MainWindow() { delete ui; } void MainWindow::on_actionNew_Window_triggered() { mDialog = new MyDialog(this); mDialog->show(); }
mainwindow.h:
#ifndef MAINWINDOW_H #define MAINWINDOW_H #include <QMainWindow> #include "mydialog.h" namespace Ui { class MainWindow; } class MainWindow : public QMainWindow { Q_OBJECT public: explicit MainWindow(QWidget *parent = 0); ~MainWindow(); private slots: void on_actionNew_Window_triggered(); private: Ui::MainWindow *ui; MyDialog *mDialog; }; #endif // MAINWINDOW_H
Now, modeless dialog does not disappear.
Qt 5 Tutorial
- Hello World
- Signals and Slots
- Q_OBJECT Macro
- MainWindow and Action
- MainWindow and ImageViewer using Designer A
- MainWindow and ImageViewer using Designer B
- Layouts
- Layouts without Designer
- Grid Layouts
- Splitter
- QDir
- QFile (Basic)
- Resource Files (.qrc)
- QComboBox
- QListWidget
- QTreeWidget
- QAction and Icon Resources
- QStatusBar
- QMessageBox
- QTimer
- QList
- QListIterator
- QMutableListIterator
- QLinkedList
- QMap
- QHash
- QStringList
- QTextStream
- QMimeType and QMimeDatabase
- QFile (Serialization I)
- QFile (Serialization II - Class)
- Tool Tips in HTML Style and with Resource Images
- QPainter
- QBrush and QRect
- QPainterPath and QPolygon
- QPen and Cap Style
- QBrush and QGradient
- QPainter and Transformations
- QGraphicsView and QGraphicsScene
- Customizing Items by inheriting QGraphicsItem
- QGraphicsView Animation
- FFmpeg Converter using QProcess
- QProgress Dialog - Modal and Modeless
- QVariant and QMetaType
- QtXML - Writing to a file
- QtXML - QtXML DOM Reading
- QThreads - Introduction
- QThreads - Creating Threads
- Creating QThreads using QtConcurrent
- QThreads - Priority
- QThreads - QMutex
- QThreads - GuiThread
- QtConcurrent QProgressDialog with QFutureWatcher
- QSemaphores - Producer and Consumer
- QThreads - wait()
- MVC - ModelView with QListView and QStringListModel
- MVC - ModelView with QTreeView and QDirModel
- MVC - ModelView with QTreeView and QFileSystemModel
- MVC - ModelView with QTableView and QItemDelegate
- QHttp - Downloading Files
- QNetworkAccessManager and QNetworkRequest - Downloading Files
- Qt's Network Download Example - Reconstructed
- QNetworkAccessManager - Downloading Files with UI and QProgressDialog
- QUdpSocket
- QTcpSocket
- QTcpSocket with Signals and Slots
- QTcpServer - Client and Server
- QTcpServer - Loopback Dialog
- QTcpServer - Client and Server using MultiThreading
- QTcpServer - Client and Server using QThreadPool
- Asynchronous QTcpServer - Client and Server using QThreadPool
- Qt Quick2 QML Animation - A
- Qt Quick2 QML Animation - B
- Short note on Ubuntu Install
- OpenGL with QT5
- Qt5 Webkit : Web Browser with QtCreator using QWebView Part A
- Qt5 Webkit : Web Browser with QtCreator using QWebView Part B
- Video Player with HTML5 QWebView and FFmpeg Converter
- Qt5 Add-in and Visual Studio 2012
- Qt5.3 Installation on Ubuntu 14.04
- Qt5.5 Installation on Ubuntu 14.04
- Short note on deploying to Windows
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization