Qt5 Tutorial QStatusBar - 2020
In this tutorial, we will learn about StatusBar.
The QStatusBar class provides a horizontal bar suitable for presenting status information.
Each status indicator falls into one of three categories:
- Temporary - briefly occupies most of the status bar. Used to explain tool tip texts or menu entries.
- Normal - occupies part of the status bar and may be hidden by temporary messages. Used to display the page and line number in a word processor.
- Permanent - is never hidden. Used for important mode indications, for example, some applications put a Caps Lock indicator in the status bar.
Typically, a request for the status bar functionality occurs in relation to a QMainWindow object. QMainWindow provides a main application window, with a menu bar, tool bars, dock widgets and a status bar around a large central widget. The status bar can be retrieved using the QMainWindow::statusBar() function, and replaced using the QMainWindow::setStatusBar() function.
In this example, we'll start from MainWindow class.
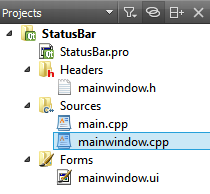
We need to add menu actions.
In this example, we'll add just one menu (MyMenuAction) to trigger action.
void MainWindow::on_actionMyMenuAction_triggered() { // showMessage(const QString & message, int timeout = 0) ui->statusBar->showMessage("Status"); // When the action triggered, set the progress bar at 51% statusProgressBar->setValue(51); }
The void QStatusBar::showMessage(const QString & message, int timeout = 0) hides the normal status indications and displays the given message for the specified number of milli-seconds (timeout). If timeout is 0 (default), the message remains displayed until the clearMessage() slot is called or until the showMessage() slot is called again to change the message.
To make the code above to work, the pointer for the ProgressBar should be set in mainwindow.h:
#ifndef MAINWINDOW_H #define MAINWINDOW_H #include <QMainWindow> #include <QLabel> #include <QProgressBar> namespace Ui { class MainWindow; } class MainWindow : public QMainWindow { Q_OBJECT public: explicit MainWindow(QWidget *parent = 0); ~MainWindow(); private slots: void on_actionMyMenuAction_triggered(); private: Ui::MainWindow *ui; // add references to Label and ProgressBar QLabel *statusLabel; QProgressBar *statusProgressBar; }; #endif // MAINWINDOW_H
Here is the implementation file, mainwindow.cpp:
#include "mainwindow.h" #include "ui_mainwindow.h" MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent), ui(new Ui::MainWindow) { ui->setupUi(this); // create objects for the label and progress bar statusLabel = new QLabel(this); statusProgressBar = new QProgressBar(this); // set text for the label statusLabel->setText("Status Label"); // make progress bar text invisible statusProgressBar->setTextVisible(false); // add the two controls to the status bar ui->statusBar->addPermanentWidget(statusLabel); ui->statusBar->addPermanentWidget(statusProgressBar,1); } MainWindow::~MainWindow() { delete ui; } void MainWindow::on_actionMyMenuAction_triggered() { // showMessage(const QString & message, int timeout = 0) ui->statusBar->showMessage("Status"); statusProgressBar->setValue(51); }
The void QStatusBar::addPermanentWidget(QWidget * widget, int stretch = 0) adds the given widget permanently to this status bar, reparenting the widget if it isn't already a child of this QStatusBar object. The stretch parameter is used to compute a suitable size for the given widget as the status bar grows and shrinks. The default stretch factor is 0, i.e giving the widget a minimum of space.
Permanently means that the widget may not be obscured by temporary messages. It is located at the far right of the status bar.
If we run the code, we get:
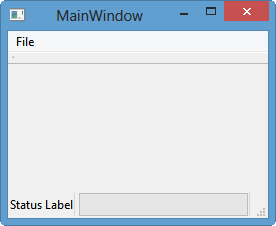
If we trigger the action:
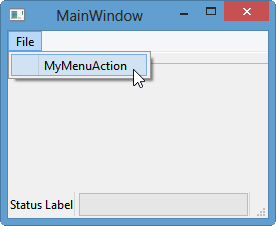
This is the final output:
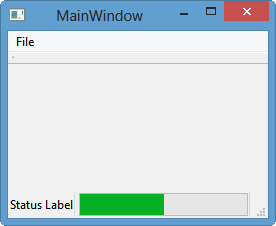
Qt 5 Tutorial
- Hello World
- Signals and Slots
- Q_OBJECT Macro
- MainWindow and Action
- MainWindow and ImageViewer using Designer A
- MainWindow and ImageViewer using Designer B
- Layouts
- Layouts without Designer
- Grid Layouts
- Splitter
- QDir
- QFile (Basic)
- Resource Files (.qrc)
- QComboBox
- QListWidget
- QTreeWidget
- QAction and Icon Resources
- QStatusBar
- QMessageBox
- QTimer
- QList
- QListIterator
- QMutableListIterator
- QLinkedList
- QMap
- QHash
- QStringList
- QTextStream
- QMimeType and QMimeDatabase
- QFile (Serialization I)
- QFile (Serialization II - Class)
- Tool Tips in HTML Style and with Resource Images
- QPainter
- QBrush and QRect
- QPainterPath and QPolygon
- QPen and Cap Style
- QBrush and QGradient
- QPainter and Transformations
- QGraphicsView and QGraphicsScene
- Customizing Items by inheriting QGraphicsItem
- QGraphicsView Animation
- FFmpeg Converter using QProcess
- QProgress Dialog - Modal and Modeless
- QVariant and QMetaType
- QtXML - Writing to a file
- QtXML - QtXML DOM Reading
- QThreads - Introduction
- QThreads - Creating Threads
- Creating QThreads using QtConcurrent
- QThreads - Priority
- QThreads - QMutex
- QThreads - GuiThread
- QtConcurrent QProgressDialog with QFutureWatcher
- QSemaphores - Producer and Consumer
- QThreads - wait()
- MVC - ModelView with QListView and QStringListModel
- MVC - ModelView with QTreeView and QDirModel
- MVC - ModelView with QTreeView and QFileSystemModel
- MVC - ModelView with QTableView and QItemDelegate
- QHttp - Downloading Files
- QNetworkAccessManager and QNetworkRequest - Downloading Files
- Qt's Network Download Example - Reconstructed
- QNetworkAccessManager - Downloading Files with UI and QProgressDialog
- QUdpSocket
- QTcpSocket
- QTcpSocket with Signals and Slots
- QTcpServer - Client and Server
- QTcpServer - Loopback Dialog
- QTcpServer - Client and Server using MultiThreading
- QTcpServer - Client and Server using QThreadPool
- Asynchronous QTcpServer - Client and Server using QThreadPool
- Qt Quick2 QML Animation - A
- Qt Quick2 QML Animation - B
- Short note on Ubuntu Install
- OpenGL with QT5
- Qt5 Webkit : Web Browser with QtCreator using QWebView Part A
- Qt5 Webkit : Web Browser with QtCreator using QWebView Part B
- Video Player with HTML5 QWebView and FFmpeg Converter
- Qt5 Add-in and Visual Studio 2012
- Qt5.3 Installation on Ubuntu 14.04
- Qt5.5 Installation on Ubuntu 14.04
- Short note on deploying to Windows
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization