Qt5 Tutorial QBrush and QGradient - 2020
In this tutorial, we will learn QGradient.
The QGradient class is used in combination with QBrush to specify gradient fills. Qt currently supports three types of gradient fills:
- Linear gradients interpolate colors between start and end points.
- Simple radial gradients interpolate colors between a focal point and end points on a circle surrounding it.
- Extended radial gradients interpolate colors between a center and a focal circle.
- Conical gradients interpolate colors around a center point.
Here is an example of gradients Qt Doc QGradient.
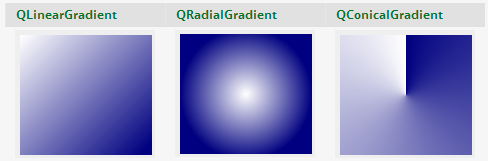
OK. Let's make own own example.
Qt->Qt Gui Application:
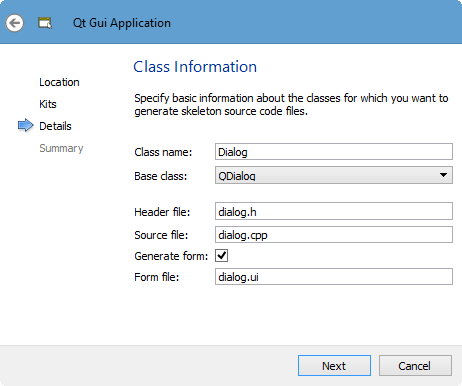
We will override QWidget::paintEvent(). That's because whenever there is a paint event, we can draw whatever we want. In other words, we let Qt know what to draw on our dialog box at the paint event.
Here is the modified dialog.h:
// dialog.h #ifndef DIALOG_H #define DIALOG_H #include <QDialog> #include <QPainter> #include <QLinearGradient> #include <QRadialGradient> #include <QConicalGradient> namespace Ui { class Dialog; } class Dialog : public QDialog { Q_OBJECT public: explicit Dialog(QWidget *parent = 0); ~Dialog(); private: Ui::Dialog *ui; protected: void paintEvent(QPaintEvent *e); }; #endif // DIALOG_H
Here, we're going to reimplement paintEvent().
Here is the implementation file, dialog.cpp:
#include "dialog.h" #include "ui_dialog.h" Dialog::Dialog(QWidget *parent) : QDialog(parent), ui(new Ui::Dialog) { ui->setupUi(this); } Dialog::~Dialog() { delete ui; } void Dialog:: paintEvent(QPaintEvent *e) { QPainter painter(this); // Gradient used with QBrush QLinearGradient linearGrad(QPointF(100, 100), QPointF(200, 200)); linearGrad.setColorAt(0, Qt::white); linearGrad.setColorAt(0.5, Qt::green); linearGrad.setColorAt(1, Qt::black); QRect rect_linear(50,50,200,200); painter.fillRect(rect_linear, linearGrad); QRadialGradient radialGrad(QPointF(400, 150), 100); radialGrad.setColorAt(0, Qt::white); radialGrad.setColorAt(0.5, Qt::green); radialGrad.setColorAt(1, Qt::black); QRect rect_radial(300,50,200,200); painter.fillRect(rect_radial, radialGrad); QConicalGradient conicalGrad(QPointF(650, 150), 90); conicalGrad.setColorAt(0, Qt::white); conicalGrad.setColorAt(0.5, Qt::green); conicalGrad.setColorAt(1, Qt::black); QRect rect_conical(550,50,200,200); painter.fillRect(rect_conical, conicalGrad); }
If we run the code, we get:
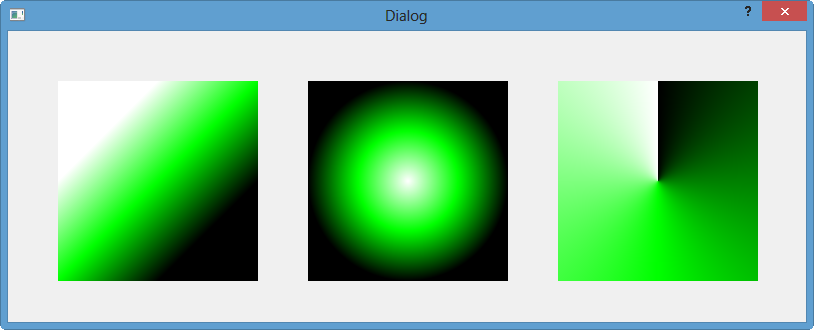
Qt 5 Tutorial
- Hello World
- Signals and Slots
- Q_OBJECT Macro
- MainWindow and Action
- MainWindow and ImageViewer using Designer A
- MainWindow and ImageViewer using Designer B
- Layouts
- Layouts without Designer
- Grid Layouts
- Splitter
- QDir
- QFile (Basic)
- Resource Files (.qrc)
- QComboBox
- QListWidget
- QTreeWidget
- QAction and Icon Resources
- QStatusBar
- QMessageBox
- QTimer
- QList
- QListIterator
- QMutableListIterator
- QLinkedList
- QMap
- QHash
- QStringList
- QTextStream
- QMimeType and QMimeDatabase
- QFile (Serialization I)
- QFile (Serialization II - Class)
- Tool Tips in HTML Style and with Resource Images
- QPainter
- QBrush and QRect
- QPainterPath and QPolygon
- QPen and Cap Style
- QBrush and QGradient
- QPainter and Transformations
- QGraphicsView and QGraphicsScene
- Customizing Items by inheriting QGraphicsItem
- QGraphicsView Animation
- FFmpeg Converter using QProcess
- QProgress Dialog - Modal and Modeless
- QVariant and QMetaType
- QtXML - Writing to a file
- QtXML - QtXML DOM Reading
- QThreads - Introduction
- QThreads - Creating Threads
- Creating QThreads using QtConcurrent
- QThreads - Priority
- QThreads - QMutex
- QThreads - GuiThread
- QtConcurrent QProgressDialog with QFutureWatcher
- QSemaphores - Producer and Consumer
- QThreads - wait()
- MVC - ModelView with QListView and QStringListModel
- MVC - ModelView with QTreeView and QDirModel
- MVC - ModelView with QTreeView and QFileSystemModel
- MVC - ModelView with QTableView and QItemDelegate
- QHttp - Downloading Files
- QNetworkAccessManager and QNetworkRequest - Downloading Files
- Qt's Network Download Example - Reconstructed
- QNetworkAccessManager - Downloading Files with UI and QProgressDialog
- QUdpSocket
- QTcpSocket
- QTcpSocket with Signals and Slots
- QTcpServer - Client and Server
- QTcpServer - Loopback Dialog
- QTcpServer - Client and Server using MultiThreading
- QTcpServer - Client and Server using QThreadPool
- Asynchronous QTcpServer - Client and Server using QThreadPool
- Qt Quick2 QML Animation - A
- Qt Quick2 QML Animation - B
- Short note on Ubuntu Install
- OpenGL with QT5
- Qt5 Webkit : Web Browser with QtCreator using QWebView Part A
- Qt5 Webkit : Web Browser with QtCreator using QWebView Part B
- Video Player with HTML5 QWebView and FFmpeg Converter
- Qt5 Add-in and Visual Studio 2012
- Qt5.3 Installation on Ubuntu 14.04
- Qt5.5 Installation on Ubuntu 14.04
- Short note on deploying to Windows
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization