Qt5 Tutorial QPainter Transformations - 2020
In this tutorial, we will learn how Transformations influence the way that QPainter renders graphics primitives.
Here is an example of transformations Qt Doc QGradient.
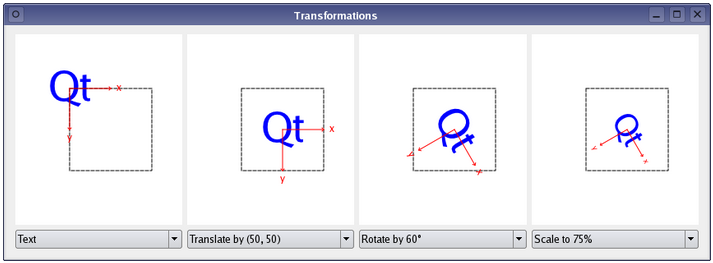
Normally, the QPainter operates on the associated device's own coordinate system, but it also has good support for coordinate transformations.
The default coordinate system of a paint device has its origin at the top-left corner. The x values increase to the right and the y values increase downwards. You can scale the coordinate system by a given offset using the QPainter::scale() function, you can rotate it clockwise using the QPainter::rotate() function and you can translate it (i.e. adding a given offset to the points) using the QPainter::translate() function. You can also twist the coordinate system around the origin (called shearing) using the QPainter::shear() function.
OK. Let's make own own example.
Qt->Qt Gui Application:
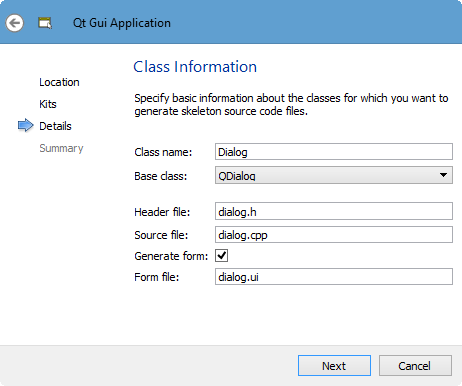
We will override QWidget::paintEvent(). That's because whenever there is a paint event, we can draw whatever we want. In other words, we let Qt know what to draw on our dialog box at the paint event.
Here is the modified dialog.h:
// dialog.h #ifndef DIALOG_H #define DIALOG_H #include <QDialog> #include <QPainter> namespace Ui { class Dialog; } class Dialog : public QDialog { Q_OBJECT public: explicit Dialog(QWidget *parent = 0); ~Dialog(); private: Ui::Dialog *ui; protected: void paintEvent(QPaintEvent *e); }; #endif // DIALOG_H
Here, we're going to reimplement paintEvent().
Here is the implementation file, dialog.cpp:
#include "dialog.h" #include "ui_dialog.h" Dialog::Dialog(QWidget *parent) : QDialog(parent), ui(new Ui::Dialog) { ui->setupUi(this); } Dialog::~Dialog() { delete ui; } void Dialog:: paintEvent(QPaintEvent *e) { { QPainter painter(this); QPen pen1(Qt::black); pen1.setWidth(5); QPen pen2(Qt::red); pen2.setWidth(5); QPen pen3(Qt::green); pen3.setWidth(5); QPen pen4(Qt::blue); pen4.setWidth(5); int x0 = 100; int y0 = 100; int width = 200; int height = 200; QRect rect(x0, y0, width, height); // original rectangle painter.setPen(pen1); painter.drawRect(rect); // rotation // rotate around (0,0) which is top-left // rect center (xc,yc) int xc = x0 + width/2; int yc = y0 + height/2; // order of transformation // (1) translate to top-left // (2) rotate // (3) move back to (xc,yc) // painter.translate(xc, yc); //(3) painter.rotate(45); //(2) painter.translate(-xc, -yc); //(1) painter.setPen(pen2); painter.drawRect(rect); // scale -> 1/2 painter.translate(xc, yc); painter.scale(0.5,0.5); painter.translate(-xc, -yc); painter.setPen(pen3); painter.drawRect(rect); // Back to the initial state // shear painter.resetTransform(); painter.translate(xc, yc); painter.shear(0.5,0.5); painter.translate(-xc, -yc); painter.setPen(pen4); painter.drawRect(rect); }
Note that when we do a transformation, the operation is performed based on the top-left corner. So, to get the expected result, we need to take the following three steps.
- Translate the object to the top-left corner.
- Do transformation.
- Translate the object back.
Important:
Any transformation is a matrix multiplication. Due to this, the order in the code should be reversed as shown in the rotation code.
If we run the code, we get:
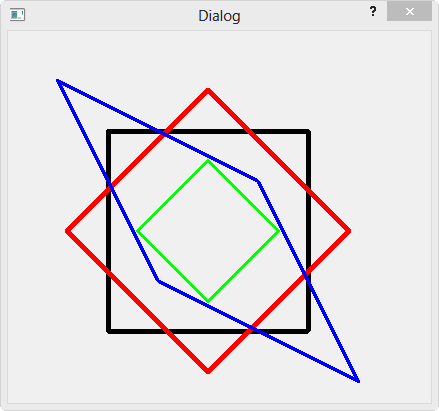
Qt 5 Tutorial
- Hello World
- Signals and Slots
- Q_OBJECT Macro
- MainWindow and Action
- MainWindow and ImageViewer using Designer A
- MainWindow and ImageViewer using Designer B
- Layouts
- Layouts without Designer
- Grid Layouts
- Splitter
- QDir
- QFile (Basic)
- Resource Files (.qrc)
- QComboBox
- QListWidget
- QTreeWidget
- QAction and Icon Resources
- QStatusBar
- QMessageBox
- QTimer
- QList
- QListIterator
- QMutableListIterator
- QLinkedList
- QMap
- QHash
- QStringList
- QTextStream
- QMimeType and QMimeDatabase
- QFile (Serialization I)
- QFile (Serialization II - Class)
- Tool Tips in HTML Style and with Resource Images
- QPainter
- QBrush and QRect
- QPainterPath and QPolygon
- QPen and Cap Style
- QBrush and QGradient
- QPainter and Transformations
- QGraphicsView and QGraphicsScene
- Customizing Items by inheriting QGraphicsItem
- QGraphicsView Animation
- FFmpeg Converter using QProcess
- QProgress Dialog - Modal and Modeless
- QVariant and QMetaType
- QtXML - Writing to a file
- QtXML - QtXML DOM Reading
- QThreads - Introduction
- QThreads - Creating Threads
- Creating QThreads using QtConcurrent
- QThreads - Priority
- QThreads - QMutex
- QThreads - GuiThread
- QtConcurrent QProgressDialog with QFutureWatcher
- QSemaphores - Producer and Consumer
- QThreads - wait()
- MVC - ModelView with QListView and QStringListModel
- MVC - ModelView with QTreeView and QDirModel
- MVC - ModelView with QTreeView and QFileSystemModel
- MVC - ModelView with QTableView and QItemDelegate
- QHttp - Downloading Files
- QNetworkAccessManager and QNetworkRequest - Downloading Files
- Qt's Network Download Example - Reconstructed
- QNetworkAccessManager - Downloading Files with UI and QProgressDialog
- QUdpSocket
- QTcpSocket
- QTcpSocket with Signals and Slots
- QTcpServer - Client and Server
- QTcpServer - Loopback Dialog
- QTcpServer - Client and Server using MultiThreading
- QTcpServer - Client and Server using QThreadPool
- Asynchronous QTcpServer - Client and Server using QThreadPool
- Qt Quick2 QML Animation - A
- Qt Quick2 QML Animation - B
- Short note on Ubuntu Install
- OpenGL with QT5
- Qt5 Webkit : Web Browser with QtCreator using QWebView Part A
- Qt5 Webkit : Web Browser with QtCreator using QWebView Part B
- Video Player with HTML5 QWebView and FFmpeg Converter
- Qt5 Add-in and Visual Studio 2012
- Qt5.3 Installation on Ubuntu 14.04
- Qt5.5 Installation on Ubuntu 14.04
- Short note on deploying to Windows
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization