Qt5 Tutorial MainWindow and ImageViewer using Creator - Part B - 2020
This tutorial is the continuation from the previous section, Main Window for ImageViewer A.
We'll finish the Actions from the menu we built in the previous tutorial. In other words, we need to connect each QAction to the appropriate slot.
We're going to connect each action to a appropriate slot using Qt Designer. The equivalent code is shown below:
connect(openAct, SIGNAL(triggered()), this, SLOT(open())); connect(printAct, SIGNAL(triggered()), this, SLOT(print())); connect(exitAct, SIGNAL(triggered()), this, SLOT(close())); connect(zoomInAct, SIGNAL(triggered()), this, SLOT(zoomIn())); connect(zoomOutAct, SIGNAL(triggered()), this, SLOT(zoomOut())); connect(normalSizeAct, SIGNAL(triggered()), this, SLOT(normalSize())); connect(fitToWindowAct, SIGNAL(triggered()), this, SLOT(fitToWindow())); connect(aboutAct, SIGNAL(triggered()), this, SLOT(about())); connect(aboutQtAct, SIGNAL(triggered()), qApp, SLOT(aboutQt()));
Let's open up Designer by double-clicking the imageviewer.ui to connect actions to slots.
Right click on the openAtc in the Action Editor, and select "Go to slot..."
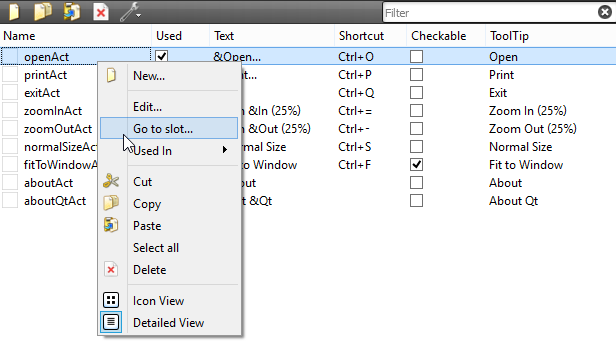
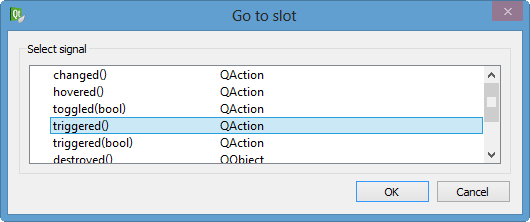
Hit OK.
Then, it will generate a code for us into imageviewer.cpp:
void ImageViewer::on_openAct_triggered() { }
Designer also writes the slot declaration to the header file, imageviewer.h, as well.
void on_openAct_triggered();
Let's switch the name of the slot to open() as shown earlier in the equivalent code. It's not necessary but we just want to have the same code in the Qt Example.
Do the same to all the actions triggered.
Also, we need to put the pointers to those actions into imageviewer.h:
QAction *openAct; QAction *printAct; QAction *exitAct; QAction *zoomInAct; QAction *zoomOutAct; QAction *normalSizeAct; QAction *fitToWindowAct; QAction *aboutAct; QAction *aboutQtAct;
Even though we defined pointers to the widgets or actions of UI in header file as shown above, we haven't assigned them any objects. So, we need to do that now in imageviewer.cpp:
... openAct = ui->openAct; printAct = ui->printAct; exitAct = ui->exitAct; zoomInAct = ui->zoomInAct; zoomOutAct = ui->zoomOutAct; normalSizeAct = ui->normalSizeAct; fitToWindowAct = ui->fitToWindowAct; aboutAct = ui->aboutAct; aboutQtAct = ui->aboutQtAct; ...
In that way, we can use the pointer openAct->* instead of ui->openAct->*.
From this point, the remaining process is almost the same as the original Qt tutorial.
We want to implement the functionality of the on_actionOpen_triggered() slot. We changed the name to open().
void ImageViewer::open() { qDebug() << "open()"; QString fileName = QFileDialog::getOpenFileName(this, tr("Open File"), QDir::currentPath()); if (!fileName.isEmpty()) { QImage image(fileName); if (image.isNull()) { QMessageBox::information(this, tr("Image Viewer"), tr("Cannot load %1.").arg(fileName)); return; } imageLabel->setPixmap(QPixmap::fromImage(image)); scaleFactor = 1.0; printAct->setEnabled(true); fitToWindowAct->setEnabled(true); updateActions(); if (!fitToWindowAct->isChecked()) imageLabel->adjustSize(); } }
The first step is asking the user for the name of the file to open. Qt comes with QFileDialog, which is a dialog from which the user can select a file.
The static getOpenFileName() function displays a modal file dialog. It returns the file path of the file selected, or an empty string if the user canceled the dialog. If the file could not be opened, we use QMessageBox to display a dialog with an error message. If the user presses Cancel, QFileDialog returns an empty string.
Unless the file name is a empty string, we check if the file's format is an image format by constructing a QImage which tries to load the image from the file. If the constructor returns a null image, we use a QMessageBox to alert the user.
The QMessageBox class provides a modal dialog with a short message, an icon, and some buttons. As with QFileDialog the easiest way to create a QMessageBox is to use its static convenience functions. QMessageBox provides a range of different messages arranged along two axes: severity (question, information, warning and critical) and complexity (the number of necessary response buttons). In this particular example an information message with an OK button (the default) is sufficient, since the message is part of a normal operation.
If the format is supported, we display the image in imageLabel by setting the label's pixmap. Then we enable the Print and Fit to Window menu entries and update the rest of the view menu entries. The Open and Exit entries are enabled by default.
If the Fit to Window option is turned off, the QScrollArea::widgetResizable property is false and it is our responsibility (not QScrollArea's) to give the QLabel a reasonable size based on its contents. We call {QWidget::adjustSize()}{adjustSize()} to achieve this, which is essentially the same as
imageLabel->resize(imageLabel->pixmap()->size());
void ImageViewer::zoomIn() { scaleImage(1.25); } void ImageViewer::zoomOut() { scaleImage(0.8); } void ImageViewer::normalSize() { imageLabel->adjustSize(); scaleFactor = 1.0; }
We implement the zooming slots using the private scaleImage() function. We set the scaling factors to 1.25 and 0.8, respectively. These factor values ensure that a Zoom In action and a Zoom Out action will cancel each other (since 1.25 * 0.8 == 1), and in that way the normal image size can be restored using the zooming features.
When zooming, we use the QLabel's ability to scale its contents. Such scaling doesn't change the actual size hint of the contents. And since the adjustSize() function use those size hint, the only thing we need to do to restore the normal size of the currently displayed image is to call adjustSize() and reset the scale factor to 1.0.
void ImageViewer::fitToWindow() { bool fitToWindow = fitToWindowAct->isChecked(); scrollArea->setWidgetResizable(fitToWindow); if (!fitToWindow) { normalSize(); } updateActions(); }
The fitToWindow() slot is called each time the user toggled the Fit to Window option. If the slot is called to turn on the option, we tell the scroll area to resize its child widget with the QScrollArea::setWidgetResizable() function. Then we disable the Zoom In, Zoom Out and Normal Size menu entries using the private updateActions() function.
If the QScrollArea::widgetResizable property is set to false (the default), the scroll area honors the size of its child widget. If this property is set to true, the scroll area will automatically resize the widget in order to avoid scroll bars where they can be avoided, or to take advantage of extra space. But the scroll area will honor the minimum size hint of its child widget independent of the widget resizable property. So in this example we set imageLabel's size policy to ignored in the constructor, to avoid that scroll bars appear when the scroll area becomes smaller than the label's minimum size hint.
If the slot is called to turn off the option, the {QScrollArea::setWidgetResizable} property is set to false. We also restore the image pixmap to its normal size by adjusting the label's size to its content. And in the end we update the view menu entries.
void ImageViewer::updateActions() { zoomInAct->setEnabled(!fitToWindowAct->isChecked()); zoomOutAct->setEnabled(!fitToWindowAct->isChecked()); normalSizeAct->setEnabled(!fitToWindowAct->isChecked()); }
The private updateActions() function enables or disables the Zoom In, Zoom Out and Normal Size menu entries depending on whether the Fit to Window option is turned on or off.
void ImageViewer::scaleImage(double factor) { Q_ASSERT(imageLabel->pixmap()); scaleFactor *= factor; imageLabel->resize(scaleFactor * imageLabel->pixmap()->size()); adjustScrollBar(scrollArea->horizontalScrollBar(), factor); adjustScrollBar(scrollArea->verticalScrollBar(), factor); zoomInAct->setEnabled(scaleFactor < 3.0); zoomOutAct->setEnabled(scaleFactor > 0.333); }
In scaleImage(), we use the factor parameter to calculate the new scaling factor for the displayed image, and resize imageLabel. Since we set the scaledContents property to true in the constructor, the call to QWidget::resize() will scale the image displayed in the label. We also adjust the scroll bars to preserve the focal point of the image.
At the end, if the scale factor is less than 33.3% or greater than 300%, we disable the respective menu entry to prevent the image pixmap from becoming too large, consuming too much resources in the window system.
void ImageViewer::adjustScrollBar(QScrollBar *scrollBar, double factor) { scrollBar->setValue(int(factor * scrollBar->value() + ((factor - 1) * scrollBar->pageStep()/2))); }
Whenever we zoom in or out, we need to adjust the scroll bars in consequence. It would have been tempting to simply call
scrollBar->setValue(int(factor * scrollBar->value()));
but this would make the top-left corner the focal point, not the center. Therefore we need to take into account the scroll bar handle's size (the page step).
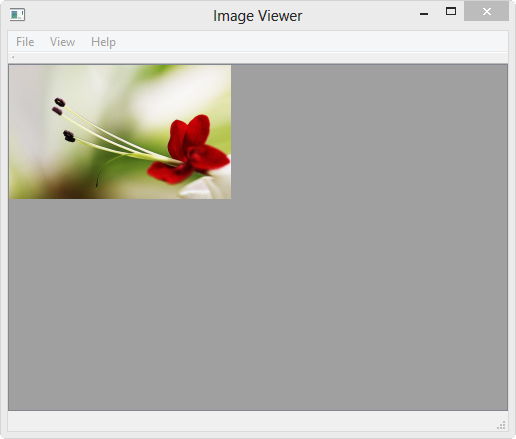
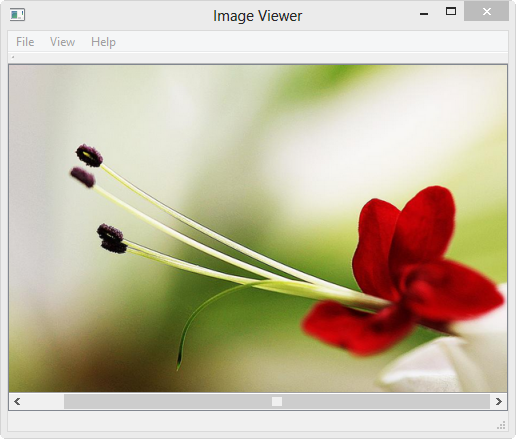
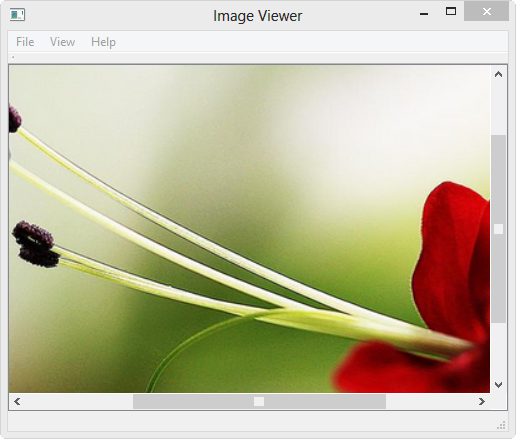
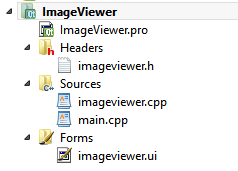
Qt 5 Tutorial
- Hello World
- Signals and Slots
- Q_OBJECT Macro
- MainWindow and Action
- MainWindow and ImageViewer using Designer A
- MainWindow and ImageViewer using Designer B
- Layouts
- Layouts without Designer
- Grid Layouts
- Splitter
- QDir
- QFile (Basic)
- Resource Files (.qrc)
- QComboBox
- QListWidget
- QTreeWidget
- QAction and Icon Resources
- QStatusBar
- QMessageBox
- QTimer
- QList
- QListIterator
- QMutableListIterator
- QLinkedList
- QMap
- QHash
- QStringList
- QTextStream
- QMimeType and QMimeDatabase
- QFile (Serialization I)
- QFile (Serialization II - Class)
- Tool Tips in HTML Style and with Resource Images
- QPainter
- QBrush and QRect
- QPainterPath and QPolygon
- QPen and Cap Style
- QBrush and QGradient
- QPainter and Transformations
- QGraphicsView and QGraphicsScene
- Customizing Items by inheriting QGraphicsItem
- QGraphicsView Animation
- FFmpeg Converter using QProcess
- QProgress Dialog - Modal and Modeless
- QVariant and QMetaType
- QtXML - Writing to a file
- QtXML - QtXML DOM Reading
- QThreads - Introduction
- QThreads - Creating Threads
- Creating QThreads using QtConcurrent
- QThreads - Priority
- QThreads - QMutex
- QThreads - GuiThread
- QtConcurrent QProgressDialog with QFutureWatcher
- QSemaphores - Producer and Consumer
- QThreads - wait()
- MVC - ModelView with QListView and QStringListModel
- MVC - ModelView with QTreeView and QDirModel
- MVC - ModelView with QTreeView and QFileSystemModel
- MVC - ModelView with QTableView and QItemDelegate
- QHttp - Downloading Files
- QNetworkAccessManager and QNetworkRequest - Downloading Files
- Qt's Network Download Example - Reconstructed
- QNetworkAccessManager - Downloading Files with UI and QProgressDialog
- QUdpSocket
- QTcpSocket
- QTcpSocket with Signals and Slots
- QTcpServer - Client and Server
- QTcpServer - Loopback Dialog
- QTcpServer - Client and Server using MultiThreading
- QTcpServer - Client and Server using QThreadPool
- Asynchronous QTcpServer - Client and Server using QThreadPool
- Qt Quick2 QML Animation - A
- Qt Quick2 QML Animation - B
- Short note on Ubuntu Install
- OpenGL with QT5
- Qt5 Webkit : Web Browser with QtCreator using QWebView Part A
- Qt5 Webkit : Web Browser with QtCreator using QWebView Part B
- Video Player with HTML5 QWebView and FFmpeg Converter
- Qt5 Add-in and Visual Studio 2012
- Qt5.3 Installation on Ubuntu 14.04
- Qt5.5 Installation on Ubuntu 14.04
- Short note on deploying to Windows
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization