Qt5 Tutorial Customizing Items by inheriting QGraphicsItem - 2020
In this tutorial, we will learn QGraphicsItems.
The QGraphicsItems class is the base class for all graphical items in a QGraphicsScene
It provides a light-weight foundation for writing your own custom items. This includes defining the item's geometry, collision detection, its painting implementation and item interaction through its event handlers. QGraphicsItem is part of the Graphics View Framework.
Qt provides a set of standard graphics items for the most common shapes. These are:
- QGraphicsEllipseItem provides an ellipse item
- QGraphicsLineItem provides a line item
- QGraphicsPathItem provides an arbitrary path item
- QGraphicsPixmapItem provides a pixmap item
- QGraphicsPolygonItem provides a polygon item
- QGraphicsRectItem provides a rectangular item
- QGraphicsSimpleTextItem provides a simple text label item
- QGraphicsTextItem provides an advanced text browser item
![]()
Picture source: http://qt-project.org/doc/qt-5.0/qtwidgets/qgraphicsitem.html
We make our customized item.
When the used clicks on the item, it will change its color and shape.
Here is the normal picture:
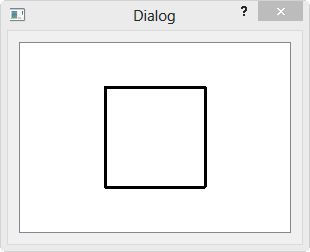
But when the mouse is pressed, it changes to circle with red color:
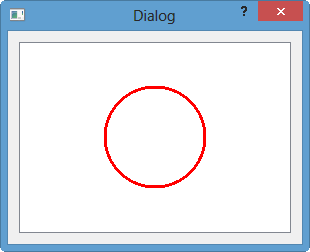
This tutorial is a continuation of the previous turotial for QGraphicsView and QGraphicsScene. We'll do some customization to the QGraphicsItems such as changing item colors when we click.
Qt->Qt Gui Application:
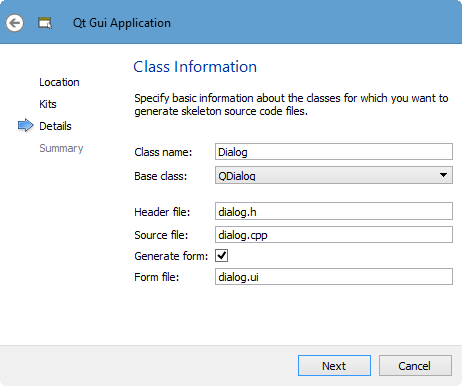
We need to put GraphicsView into our dialog box:
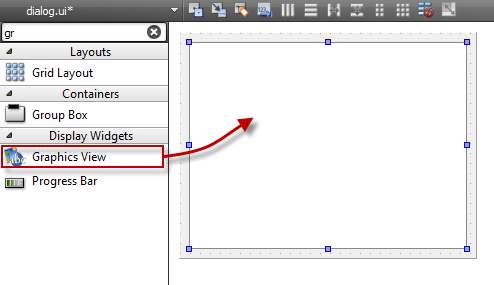
We want to create a class MyItem:
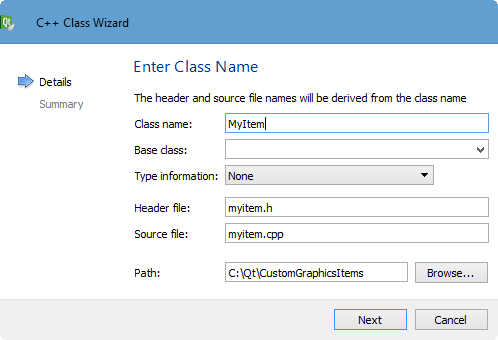
As we see from the picture below, to customize our item, we override inherited virtual function, paint(), and mouse events such as mousePressEvent() and mouseReleaseEvent(). Also we need to specify the bounding box as well.
To write your own graphics item, you first create a subclass of QGraphicsItem, and then start by implementing its two pure virtual public functions: boundingRect(), which returns an estimate of the area painted by the item, and paint(), which implements the actual painting.
- from http://qt-project.org/doc/qt-5.0/qtwidgets/qgraphicsitem.html.
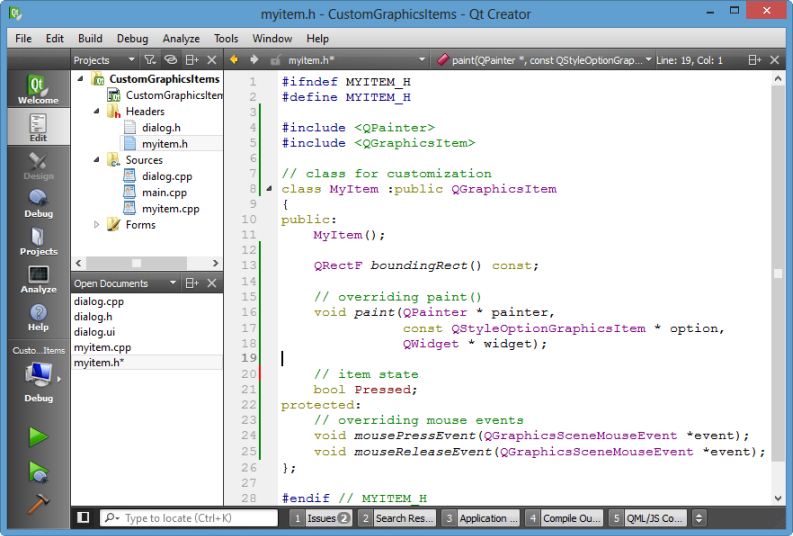
Now, we need to implement the methods in the myitem header file.
Right mouse click on the prototype -> Refactor -> Add Definition in myitem.cpp:
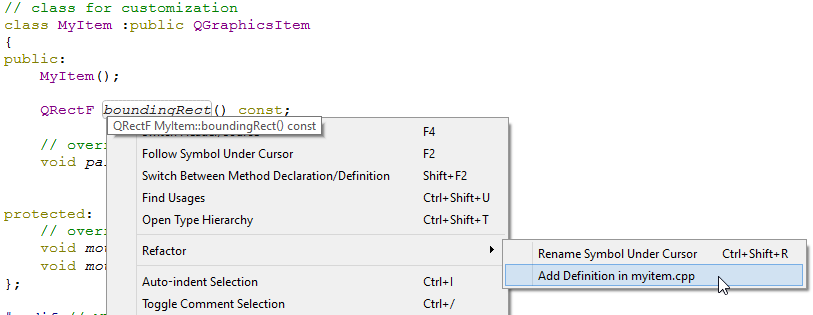
As mentioned earlier, since MyItem inherits QGraphicsItem, it should reimplement the virtual functions. Here is the modified cpp file: myitem.cpp:
// myitem.cpp #include "myitem.h" MyItem::MyItem() { Pressed = false; setFlag(ItemIsMovable); } QRectF MyItem::boundingRect() const { // outer most edges return QRectF(0,0,100,100); } void MyItem::paint(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget) { QRectF rect = boundingRect(); if(Pressed) { QPen pen(Qt::red, 3); painter->setPen(pen); painter->drawEllipse(rect); } else { QPen pen(Qt::black, 3); painter->setPen(pen); painter->drawRect(rect); } } void MyItem::mousePressEvent(QGraphicsSceneMouseEvent *event) { Pressed = true; update(); QGraphicsItem::mousePressEvent(event); } void MyItem::mouseReleaseEvent(QGraphicsSceneMouseEvent *event) { Pressed = false; update(); QGraphicsItem::mouseReleaseEvent(event); }
Here is the header, myitem.h:
// myitem.h #ifndef MYITEM_H #define MYITEM_H #include <QPainter> #include <QGraphicsItem> // class for customization class MyItem :public QGraphicsItem { public: MyItem(); QRectF boundingRect() const; // overriding paint() void paint(QPainter * painter, const QStyleOptionGraphicsItem * option, QWidget * widget); // item state bool Pressed; protected: // overriding mouse events void mousePressEvent(QGraphicsSceneMouseEvent *event); void mouseReleaseEvent(QGraphicsSceneMouseEvent *event); }; #endif // MYITEM_H
So, we have customized item.
Now, what?
In the dialog.cpp below, we're automatically adding our item to the scene when the dialog (it has GraphicsView) opens up:
// dialog.cpp #include "dialog.h" #include "ui_dialog.h" Dialog::Dialog(QWidget *parent) : QDialog(parent), ui(new Ui::Dialog) { ui->setupUi(this); scene = new QGraphicsScene(this); ui->graphicsView->setScene(scene); // create our object and add it to the scene item = new MyItem(); scene->addItem(item); } Dialog::~Dialog() { delete ui; }
Header file, dialog.h:
// dialog.h #ifndef DIALOG_H #define DIALOG_H #include <QDialog> #include <QGraphicsScene> #include "myitem.h" namespace Ui { class Dialog; } class Dialog : public QDialog { Q_OBJECT public: explicit Dialog(QWidget *parent = 0); ~Dialog(); private: Ui::Dialog *ui; QGraphicsScene *scene; MyItem *item; }; #endif // DIALOG_H
We can grab all files for the project: CustomGraphicsItems.zip.
Qt 5 Tutorial
- Hello World
- Signals and Slots
- Q_OBJECT Macro
- MainWindow and Action
- MainWindow and ImageViewer using Designer A
- MainWindow and ImageViewer using Designer B
- Layouts
- Layouts without Designer
- Grid Layouts
- Splitter
- QDir
- QFile (Basic)
- Resource Files (.qrc)
- QComboBox
- QListWidget
- QTreeWidget
- QAction and Icon Resources
- QStatusBar
- QMessageBox
- QTimer
- QList
- QListIterator
- QMutableListIterator
- QLinkedList
- QMap
- QHash
- QStringList
- QTextStream
- QMimeType and QMimeDatabase
- QFile (Serialization I)
- QFile (Serialization II - Class)
- Tool Tips in HTML Style and with Resource Images
- QPainter
- QBrush and QRect
- QPainterPath and QPolygon
- QPen and Cap Style
- QBrush and QGradient
- QPainter and Transformations
- QGraphicsView and QGraphicsScene
- Customizing Items by inheriting QGraphicsItem
- QGraphicsView Animation
- FFmpeg Converter using QProcess
- QProgress Dialog - Modal and Modeless
- QVariant and QMetaType
- QtXML - Writing to a file
- QtXML - QtXML DOM Reading
- QThreads - Introduction
- QThreads - Creating Threads
- Creating QThreads using QtConcurrent
- QThreads - Priority
- QThreads - QMutex
- QThreads - GuiThread
- QtConcurrent QProgressDialog with QFutureWatcher
- QSemaphores - Producer and Consumer
- QThreads - wait()
- MVC - ModelView with QListView and QStringListModel
- MVC - ModelView with QTreeView and QDirModel
- MVC - ModelView with QTreeView and QFileSystemModel
- MVC - ModelView with QTableView and QItemDelegate
- QHttp - Downloading Files
- QNetworkAccessManager and QNetworkRequest - Downloading Files
- Qt's Network Download Example - Reconstructed
- QNetworkAccessManager - Downloading Files with UI and QProgressDialog
- QUdpSocket
- QTcpSocket
- QTcpSocket with Signals and Slots
- QTcpServer - Client and Server
- QTcpServer - Loopback Dialog
- QTcpServer - Client and Server using MultiThreading
- QTcpServer - Client and Server using QThreadPool
- Asynchronous QTcpServer - Client and Server using QThreadPool
- Qt Quick2 QML Animation - A
- Qt Quick2 QML Animation - B
- Short note on Ubuntu Install
- OpenGL with QT5
- Qt5 Webkit : Web Browser with QtCreator using QWebView Part A
- Qt5 Webkit : Web Browser with QtCreator using QWebView Part B
- Video Player with HTML5 QWebView and FFmpeg Converter
- Qt5 Add-in and Visual Studio 2012
- Qt5.3 Installation on Ubuntu 14.04
- Qt5.5 Installation on Ubuntu 14.04
- Short note on deploying to Windows
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization