Qt5 Tutorial QtConcurrent QProgressDialog with QFutureWatcher - 2020
In this tutorial, we'll learn how to use QProgressDialog with QtConcurrent. Also, we'll learn how to use the QFuture class of the QtConcurrent module to execute a long lasting operation in a separated thread and how to monitor the progress and state with the QFutureWatcher class.
We'll see how the QtConcurrent detects ideal number of threads for the system and make them work for our massive task in parallel.
I've already covered the QProgressDialog in my tutorial: QProgress Dialog - Modal and Modeless.
But there are some methods (actually slots) of QProgressDialog class we haven't used, and some of them will appear in this tutorial.
They are:
- void reset()
Resets the progress dialog. The progress dialog becomes hidden if autoClose() is true. - void setLabelText(const QString & text)
- void setRange(int minimum, int maximum)
Sets the progress dialog's minimum and maximum values to minimum and maximum, respectively.
If maximum is smaller than minimum, minimum becomes the only legal value.
If the current value falls outside the new range, the progress dialog is reset with reset().
When we do multi-threading coding, thanks to QtConcurrent, we may not necessarily deal with low-level threading primitives such as mutexes, read-write locks, wait conditions, or semaphores. Instead, we just write our code using high-level APIs that QtConcurrent.
Among those APIs, we should know QtConcurrent::map() since it will be used in this tutorial. What it does is it applies a function to every item in a container, modifying the items in-place. For example:
QtConcurrent::map(vector, function)So, to every item in the vector, the function will be applied.
QFuture class represents the result of an asynchronous computation. We've already used this to get the return value from QtConcurrent::run() in our previous tutorial (a href="http://www.bogotobogo.com/Qt/Qt5_QtConcurrent_RunFunction_QThread.php" target="_blank">Creating QThreads using QtConcurrent):
QFuturet1 = QtConcurrent::run(myRunFunction, QString("A"));
The QFutureWatcher class allows us to monitor a QFuture using signals and slots.
QFutureWatcher provides information and notifications about a QFuture. Use the setFuture() function to start watching a particular QFuture. The future() function returns the future set with setFuture().
In our example, the following signal will be used:
- void canceled()
- void finished()
- void progressRangeChanged(int minimum, int maximum)
- void progressValueChanged(int progressValue)
Also in this tutorial, we'll use:
void QFutureWatcher::setFuture(const QFuturefunction. This starts watching the given future. One of the signals might be emitted for the current state of the future. For example, if the future is already stopped, the finished signal will be emitted. To avoid a race condition, it is important to call this function after doing the connections as we do in this tutorial:& future)
QObject::connect ... futureWatcher.setFuture(QtConcurrent::map(vector, spin));
Let's run the code:
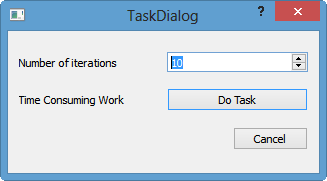
The UI of this sample application consists of two buttons, one to start the time consuming work and one to cancel the launcher itself. We get the maximum iteration from the spinBox.
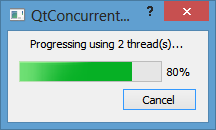
The progress of the operation is visualized by a progress bar. We can cancel the task at any time. Above the cancel is a label that shows the number of CPU cores that are used for the calculation.
iteration 0 in thread 0x1d64 iteration 1 in thread 0x7b8 iteration 2 in thread 0x1d64 iteration 3 in thread 0x7b8 iteration 4 in thread 0x1d64 iteration 5 in thread 0x7b8 iteration 6 in thread 0x1d64 iteration 8 in thread 0x1d64 iteration 7 in thread 0x7b8 iteration 9 in thread 0x1d64 Canceled? false
The ProgressDialog is the core class in this tutorial that contains all the business logic.
It provides the two slots to start and cancel the calculation and properties that reflect the state and progress of the calculation. ProgressDialog contains a QFutureWatcher as member variable, which is used to monitor the progress and state of a QFuture instance. For this the signals of the future watcher are connected against private slots inside the constructor of ProgressDialog.
void TaskDialog::on_doTaskButton_clicked() { // get the number of iterations int iterations = ui->spinBoxIterations->text().toInt(); // Prepare the vector. QVector<int> vector; for (int i = 0; i < iterations; ++i) vector.append(i); // Create a progress dialog. QProgressDialog dialog; dialog.setLabelText(QString("Progressing using %1 thread(s)...").arg(QThread::idealThreadCount())); // Create a QFutureWatcher and connect signals and slots. // Monitor progress changes of the future QFutureWatcher<void> futureWatcher; QObject::connect(&futureWatcher;, SIGNAL(finished()), &dialog;, SLOT(reset())); QObject::connect(&dialog;, SIGNAL(canceled()), &futureWatcher;, SLOT(cancel())); QObject::connect(&futureWatcher;, SIGNAL(progressRangeChanged(int,int)), &dialog;, SLOT(setRange(int,int))); QObject::connect(&futureWatcher;, SIGNAL(progressValueChanged(int)), &dialog;, SLOT(setValue(int))); // Start the computation. futureWatcher.setFuture(QtConcurrent::map(vector, spin)); // Display the dialog and start the event loop. dialog.exec(); futureWatcher.waitForFinished(); // Query the future to check if was canceled. qDebug() << "Canceled?" << futureWatcher.future().isCanceled(); }
When we hit "Do Task" button on the TaskDialog, the on_doTaskButton_clicked() slot is invoked.
After getting the number of iterations
int iterations = ui->spinBoxIterations->text().toInt();
a vector is filled with some dummy value that act as input values for the task.
// Prepare the vector. QVector<int> vector; for (int i = 0; i < iterations; ++i) vector.append(i);Then the actual calculation is started by calling QtConcurrent::map().
// Start the computation. futureWatcher.setFuture(QtConcurrent::map(vector, spin));This method from the QtConcurrent module takes a container and a function as parameters and executes the function once for each entry in the container in a separated thread. The function spin() we use in this example just burns some CPU cycles:
void spin(int& iteration) { const int work = 1000 * 1000 * 40; volatile int v = 0; for (int j = 0; j < work; ++j) ++v; qDebug() << "iteration" << iteration << "in thread" << QThread::currentThreadId(); }
Note that the call to QtConcurrent::map() does not block until the task has finished but returns a QFuture object instead, which acts as a handle to monitor the progress and state of the task.
We set the returned future on the future watcher member variable and now the future watcher will emit the appropriated signals as soon as the future changes its progress or state.
When the user wants to cancel the calculation and clicks on the 'Cancel' button, then the canceled() signal is invoked, which calls cancel() on the QFutureWatcher object. This will cause the running task (and the used threads) to terminate.
QObject::connect(&dialog;, SIGNAL(canceled()), &futureWatcher;, SLOT(cancel()));
Internally the QtConcurrent module uses the QThreadPool class for distributing the calculation over multiple threads. The thread pool uses as many threads in parallel as returned by QThread::idealThreadCount(), which by default is the number of cores of the device the application is running on.
QProgressDialog dialog; dialog.setLabelText(QString("Progressing using %1 thread(s)...").arg(QThread::idealThreadCount()));
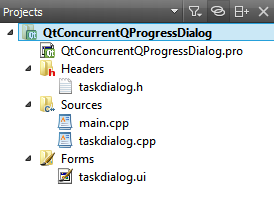
Qt 5 Tutorial
- Hello World
- Signals and Slots
- Q_OBJECT Macro
- MainWindow and Action
- MainWindow and ImageViewer using Designer A
- MainWindow and ImageViewer using Designer B
- Layouts
- Layouts without Designer
- Grid Layouts
- Splitter
- QDir
- QFile (Basic)
- Resource Files (.qrc)
- QComboBox
- QListWidget
- QTreeWidget
- QAction and Icon Resources
- QStatusBar
- QMessageBox
- QTimer
- QList
- QListIterator
- QMutableListIterator
- QLinkedList
- QMap
- QHash
- QStringList
- QTextStream
- QMimeType and QMimeDatabase
- QFile (Serialization I)
- QFile (Serialization II - Class)
- Tool Tips in HTML Style and with Resource Images
- QPainter
- QBrush and QRect
- QPainterPath and QPolygon
- QPen and Cap Style
- QBrush and QGradient
- QPainter and Transformations
- QGraphicsView and QGraphicsScene
- Customizing Items by inheriting QGraphicsItem
- QGraphicsView Animation
- FFmpeg Converter using QProcess
- QProgress Dialog - Modal and Modeless
- QVariant and QMetaType
- QtXML - Writing to a file
- QtXML - QtXML DOM Reading
- QThreads - Introduction
- QThreads - Creating Threads
- Creating QThreads using QtConcurrent
- QThreads - Priority
- QThreads - QMutex
- QThreads - GuiThread
- QtConcurrent QProgressDialog with QFutureWatcher
- QSemaphores - Producer and Consumer
- QThreads - wait()
- MVC - ModelView with QListView and QStringListModel
- MVC - ModelView with QTreeView and QDirModel
- MVC - ModelView with QTreeView and QFileSystemModel
- MVC - ModelView with QTableView and QItemDelegate
- QHttp - Downloading Files
- QNetworkAccessManager and QNetworkRequest - Downloading Files
- Qt's Network Download Example - Reconstructed
- QNetworkAccessManager - Downloading Files with UI and QProgressDialog
- QUdpSocket
- QTcpSocket
- QTcpSocket with Signals and Slots
- QTcpServer - Client and Server
- QTcpServer - Loopback Dialog
- QTcpServer - Client and Server using MultiThreading
- QTcpServer - Client and Server using QThreadPool
- Asynchronous QTcpServer - Client and Server using QThreadPool
- Qt Quick2 QML Animation - A
- Qt Quick2 QML Animation - B
- Short note on Ubuntu Install
- OpenGL with QT5
- Qt5 Webkit : Web Browser with QtCreator using QWebView Part A
- Qt5 Webkit : Web Browser with QtCreator using QWebView Part B
- Video Player with HTML5 QWebView and FFmpeg Converter
- Qt5 Add-in and Visual Studio 2012
- Qt5.3 Installation on Ubuntu 14.04
- Qt5.5 Installation on Ubuntu 14.04
- Short note on deploying to Windows
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization