Qt5 Tutorial QProgressDialog - 2020
In this tutorial, we will learn about QProgressDialog.
We'll start with Qt Gui Application using QDialog as a launching pad for the two types of QProgressDialogs: Modal and Modeless.
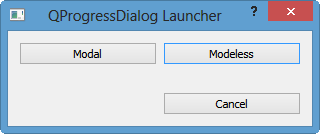
The QProgressDialog class provides feedback on the progress of a slow operation.
A progress dialog is used to give the user an indication of how long an operation is going to take, and to demonstrate that the application has not frozen. It can also give the user an opportunity to abort (cancel) the operation.
A common problem with progress dialogs is that it is difficult to know when to use them; operations take different amounts of time on different hardware. QProgressDialog offers a solution to this problem: it estimates the time the operation will take (based on time for steps), and only shows itself if that estimate is beyond minimumDuration() (4 seconds by default).
For QProgressDialog with QtConcurrent, please visit another tutorial - QtConcurrent QProgressDialog with QFutureWatcher.
A modal QProgressDialog is simpler to use for the programmer compared to a modeless QProgressDialog.
The steps are:
- Do the operation in a loop.
- Call setValue() at intervals.
- Check for cancellation with wasCanceled().
In our example, the modal QProgressDialog is launched when a modal-button on the lauching dialog is clicked.
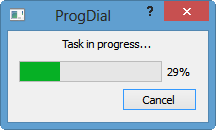
The code looks like this:
void Dialog::on_modalButton_clicked() { int numTasks = 100000; QProgressDialog progress("Task in progress...", "Cancel", 0, numTasks, this); progress.setWindowModality(Qt::WindowModal); for (int i = 0; i < numTasks; i++) { progress.setValue(i); if (progress.wasCanceled()) break; } progress.setValue(numTasks); }
We have two constructors for QProgressDialog class:
- QProgressDialog::QProgressDialog(QWidget * parent = 0, Qt::WindowFlags f = 0)
Constructs a progress dialog. Default settings:- The label text is empty.
- The cancel button text is (translated) "Cancel".
- minimum is 0.
- maximum is 100.
- QProgressDialog::QProgressDialog(const QString & labelText, const QString & cancelButtonText, int minimum, int maximum, QWidget * parent = 0, Qt::WindowFlags f = 0)
Constructs a progress dialog.- The labelText is the text used to remind the user what is progressing.
- The cancelButtonText is the text to display on the cancel button. If QString() is passed then no cancel button is shown.
- The minimum and maximum is the number of steps in the operation for which this progress dialog shows progress. For example, if the operation is to examine 50 files, this value minimum value would be 0, and the maximum would be 50. Before examining the first file, call setValue(0). As each file is processed call setValue(1), setValue(2), etc., finally calling setValue(50) after examining the last file.
- The parent argument is the dialog's parent widget. The parent, parent, and widget flags, f, are passed to the QDialog::QDialog() constructor.
Compared to a modal type, modeless type is more complicated.
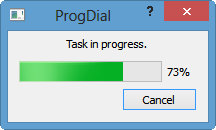
A modeless progress dialog is suitable for operations that take place in the background, where the user is able to interact with the application. Such operations are typically based on QTimer (or QObject::timerEvent()), QSocketNotifier, or QUrlOperator or performed in a separate thread. A QProgressBar in the status bar of our main window is often an alternative to a modeless progress dialog.
We need to have an event loop to be running, connect the canceled() signal to a slot that stops the operation, and call setValue() at intervals. The code used in this tutorial is:
void Dialog::on_modelessButton_clicked() { myTask = new MyTask; } ... // mytask.cpp #include "mytask.h" MyTask::MyTask(QObject *parent) : QObject(parent), steps(0) { pd = new QProgressDialog("Task in progress.", "Cancel", 0, 100000); connect(pd, SIGNAL(canceled()), this, SLOT(cancel())); t = new QTimer(this); connect(t, SIGNAL(timeout()), this, SLOT(perform())); t->start(0); } void MyTask::perform() { pd->setValue(steps); //... perform one percent of the operation steps++; if (steps > pd->maximum()) t->stop(); } void MyTask::cancel() { t->stop(); //... cleanup }
So, in this example, QProgressDialog is instantiated within the constructor of MyTask class which has the other methods such as preform() and cancel which are used as slots for the timeout() and canceled() signals, respectively.
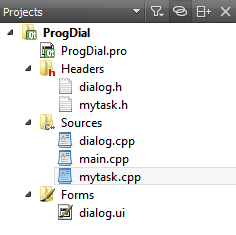
We can get all the project files from ProgDial.zip.
The files are listed below:
main.cpp:
#include "dialog.h" #include <QApplication> int main(int argc, char *argv[]) { QApplication a(argc, argv); Dialog w; w.show(); return a.exec(); }
dialog.h:
// dialog.h #ifndef DIALOG_H #define DIALOG_H #include <QDialog> #include "mytask.h" namespace Ui { class Dialog; } class Dialog : public QDialog { Q_OBJECT public: explicit Dialog(QWidget *parent = 0); ~Dialog(); private slots: void on_modalButton_clicked(); void on_modelessButton_clicked(); private: Ui::Dialog *ui; MyTask *myTask; }; #endif // DIALOG_H
dialog.cpp:
// dialog.cpp #include "dialog.h" #include "ui_dialog.h" #include <QProgressDialog> Dialog::Dialog(QWidget *parent) : QDialog(parent), ui(new Ui::Dialog) { ui->setupUi(this); } Dialog::~Dialog() { delete ui; } void Dialog::on_modalButton_clicked() { int numTasks = 100000; QProgressDialog progress("Task in progress...", "Cancel", 0, numTasks, this); progress.setWindowModality(Qt::WindowModal); for (int i = 0; i < numTasks; i++) { progress.setValue(i); if (progress.wasCanceled()) break; } progress.setValue(numTasks); } void Dialog::on_modelessButton_clicked() { myTask = new MyTask; }
mytask.h:
// mytask.h #ifndef MYTASK_H #define MYTASK_H #include <QObject> #include <QProgressDialog> #include <QTimer> class MyTask : public QObject { Q_OBJECT public: explicit MyTask(QObject *parent = 0); signals: public slots: void perform(); void cancel(); private: int steps; QProgressDialog *pd; QTimer *t; }; #endif // MYTASK_H
mytask.cpp:
// mytask.cpp #include "mytask.h" MyTask::MyTask(QObject *parent) : QObject(parent), steps(0) { pd = new QProgressDialog("Task in progress.", "Cancel", 0, 100000); connect(pd, SIGNAL(canceled()), this, SLOT(cancel())); t = new QTimer(this); connect(t, SIGNAL(timeout()), this, SLOT(perform())); t->start(0); } void MyTask::perform() { pd->setValue(steps); //... perform one percent of the operation steps++; if (steps > pd->maximum()) t->stop(); } void MyTask::cancel() { t->stop(); //... cleanup }
ProgDial.pro:
QT += core gui greaterThan(QT_MAJOR_VERSION, 4): QT += widgets TARGET = ProgDial TEMPLATE = app SOURCES += main.cpp\ dialog.cpp \ mytask.cpp HEADERS += dialog.h \ mytask.h FORMS += dialog.ui
Qt 5 Tutorial
- Hello World
- Signals and Slots
- Q_OBJECT Macro
- MainWindow and Action
- MainWindow and ImageViewer using Designer A
- MainWindow and ImageViewer using Designer B
- Layouts
- Layouts without Designer
- Grid Layouts
- Splitter
- QDir
- QFile (Basic)
- Resource Files (.qrc)
- QComboBox
- QListWidget
- QTreeWidget
- QAction and Icon Resources
- QStatusBar
- QMessageBox
- QTimer
- QList
- QListIterator
- QMutableListIterator
- QLinkedList
- QMap
- QHash
- QStringList
- QTextStream
- QMimeType and QMimeDatabase
- QFile (Serialization I)
- QFile (Serialization II - Class)
- Tool Tips in HTML Style and with Resource Images
- QPainter
- QBrush and QRect
- QPainterPath and QPolygon
- QPen and Cap Style
- QBrush and QGradient
- QPainter and Transformations
- QGraphicsView and QGraphicsScene
- Customizing Items by inheriting QGraphicsItem
- QGraphicsView Animation
- FFmpeg Converter using QProcess
- QProgress Dialog - Modal and Modeless
- QVariant and QMetaType
- QtXML - Writing to a file
- QtXML - QtXML DOM Reading
- QThreads - Introduction
- QThreads - Creating Threads
- Creating QThreads using QtConcurrent
- QThreads - Priority
- QThreads - QMutex
- QThreads - GuiThread
- QtConcurrent QProgressDialog with QFutureWatcher
- QSemaphores - Producer and Consumer
- QThreads - wait()
- MVC - ModelView with QListView and QStringListModel
- MVC - ModelView with QTreeView and QDirModel
- MVC - ModelView with QTreeView and QFileSystemModel
- MVC - ModelView with QTableView and QItemDelegate
- QHttp - Downloading Files
- QNetworkAccessManager and QNetworkRequest - Downloading Files
- Qt's Network Download Example - Reconstructed
- QNetworkAccessManager - Downloading Files with UI and QProgressDialog
- QUdpSocket
- QTcpSocket
- QTcpSocket with Signals and Slots
- QTcpServer - Client and Server
- QTcpServer - Loopback Dialog
- QTcpServer - Client and Server using MultiThreading
- QTcpServer - Client and Server using QThreadPool
- Asynchronous QTcpServer - Client and Server using QThreadPool
- Qt Quick2 QML Animation - A
- Qt Quick2 QML Animation - B
- Short note on Ubuntu Install
- OpenGL with QT5
- Qt5 Webkit : Web Browser with QtCreator using QWebView Part A
- Qt5 Webkit : Web Browser with QtCreator using QWebView Part B
- Video Player with HTML5 QWebView and FFmpeg Converter
- Qt5 Add-in and Visual Studio 2012
- Qt5.3 Installation on Ubuntu 14.04
- Qt5.5 Installation on Ubuntu 14.04
- Short note on deploying to Windows
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization