6A. CSS
Multiple CSS attributes
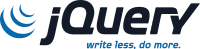
jQuery methods can manipulate the DOM in some manner and a few of them simply change one of the attributes of an element, while others set an element's style properties (CSS). Still others modify entire elements themselves by inserting, copying, removing, and so on. All of these methods are referred to as setters because they change the values of properties.
A few of the methods such as .attr(), .html(), and .val() act as getters which are retrieving information from DOM elements for later use.
In this chapter, we're going to see how we can manipulate CSS element via jQuery.
Here is our new html file (j6_css_manipulation.html):
<html> <head> <meta charset="utf-8" /> <title>CSS Manipulation via jQuery</title> <style type="text/css"> .smaller (font-size: 15px; } .emp {text-decoration: underline; color: green;} </style> </head> <body> <h1>This is test for CSS Manipulation via jQuery. </h1> <h1>1st heading</h1> <h1>2nd heading</h1> <h1>3rd heading</h1> <script type="text/javascript" src="http://code.jquery.com/jquery-2.1.0.min.js""></script> <script type="text/javascript" src="jQuery_css_manipulation.js"></script> <script> </script> </body> </html>
The following picture shows the initial html before there are any actions from jQuery:
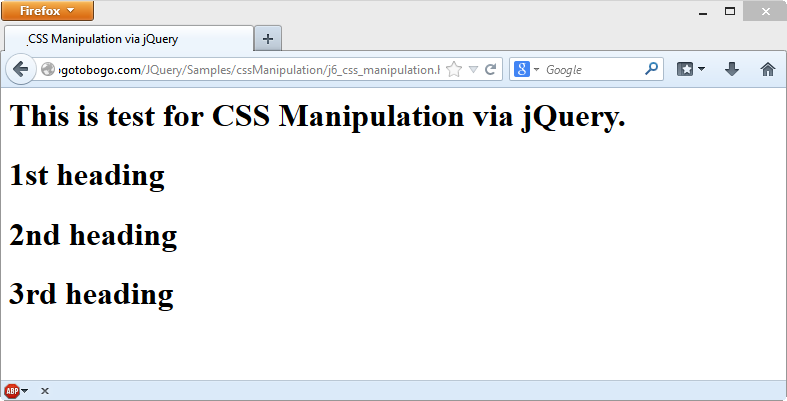
Here is our js file, jQuery_css_manipulation.js. It starts like this:
$( document ).ready(function() { $('h1').mouseenter(function(){ $(this).css("margin-left", "50px"); }); });
As we can see from the js file, when the mouse enters into h1 element, the text will have a left-margin set as 50px.
The picture below is the one when the mouse entered into the 2nd heading:
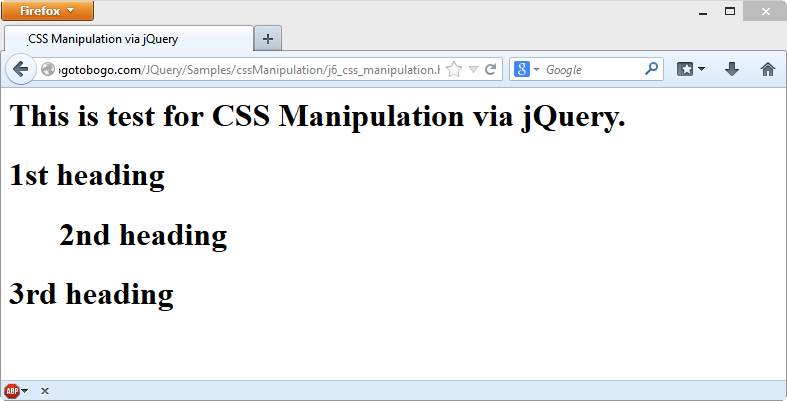
In the previous section, we manipulate only one property of CSS:
$(this).css("margin-left", "50px");
However, if we want to play with multiple properties at once, the syntax needs to be changed:
$(this).css( {"margin-left": "50px"});
We enclosed all the properties with curly braces ({}), and we need a comma between property and its value.
$( document ).ready(function() { $('h1').mouseenter(function(){ $(this).css({ "background-color": "YellowGreen", "margin-left" : "+=50px" }); }); });
The playback below shows what's happening:
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization