2A. Selectors
(div, p, etc.)
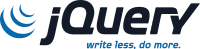
In this chapter, we'll use a selector feature of JQuery:
When we click "inputSelectorTest", it will highlight the background of a text with yellow. The text is within div tag.
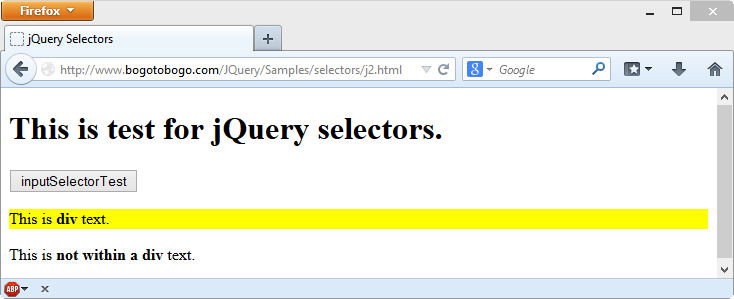
Here is the html file we're using in this example: j2.html:
<!doctype html> <html> <head> <meta charset="utf-8" /> <title>jQuery Selectors</title> </head> <body> <h1>This is test for jQuery selectors. </h1> <input id="inputButton" type="button" value="inputSelectorTest"> <br /> <div> <p>This is <b>div</b> text.</p> </div> <p>This is <b>not within a div</b> text.</p> <script type="text/javascript" src="http://code.jquery.com/jquery-2.1.0.min.js""></script> <script type="text/javascript" src="jQuery_id_div_selector.js"></script> <script> </script> </body> </html>
Let's start with the jQuery file, jQuery_id_div_selector.js.
As we discussed in the previous chapter, we put our script inside of:
$(document ).ready(function() { }
It checks whether the inputButton is clicked.
$(document ).ready(function() { // if the button is clicked, make the div element blue $('#inputButton').click(function(){ $('div').css('background-color','yellow'); }); });
If the click event occur, the background of the text with div tag will be highlighted in yellow. Note that when we select an id with $('') we need "#".
The css('background-color','yellow') statement is doing the highlighting, and the $('div') is the selector.
If we switch the selector to p from div in the js file:
$(document ).ready(function() { // if the button is clicked, make the div element blue $('#inputButton').click(function(){ $('p').css('background-color','green'); }); });
then, all the lines with p tag will be highlight as shown in the picture below:
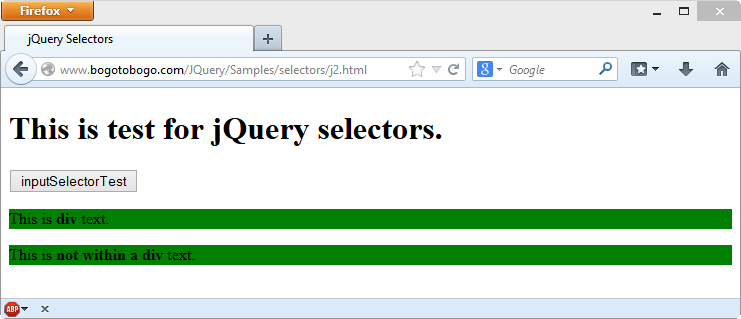
We can not only select just one tag but several:
$(document ).ready(function() { // if the button is clicked, make the div element blue $('#inputButton').click(function(){ $('div, b, #inputButton').css('background-color','cyan'); }); });
At the click event, all the elements selected can be highlighted:
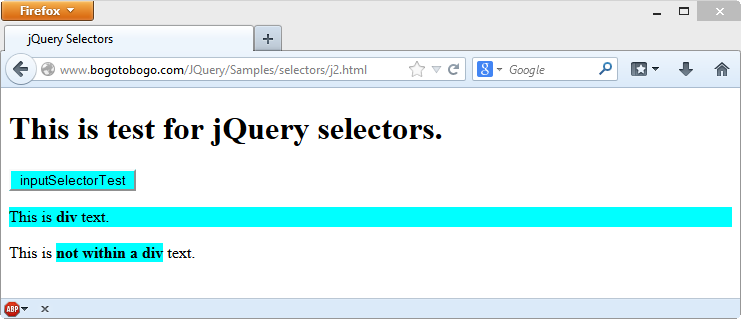
We can also select all using "*":
$(document ).ready(function() { // if the button is clicked, make the div element blue $('#inputButton').click(function(){ $('*').css('background-color','khaki'); }); });
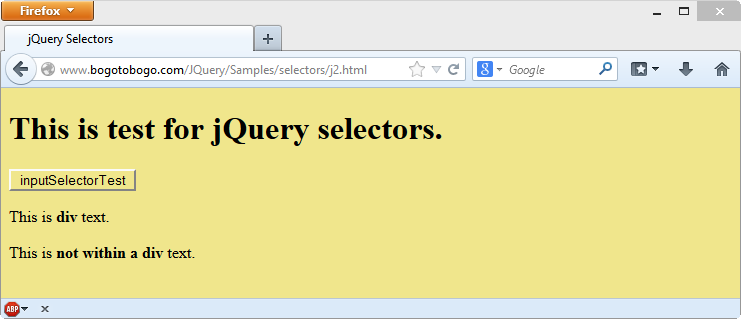
We may want to select only the text with "p" under "div":
$(document ).ready(function() { // if the button is clicked, make the div element blue $('#inputButton').click(function(){ $('div > p').css('background-color','greenyellow'); }); });
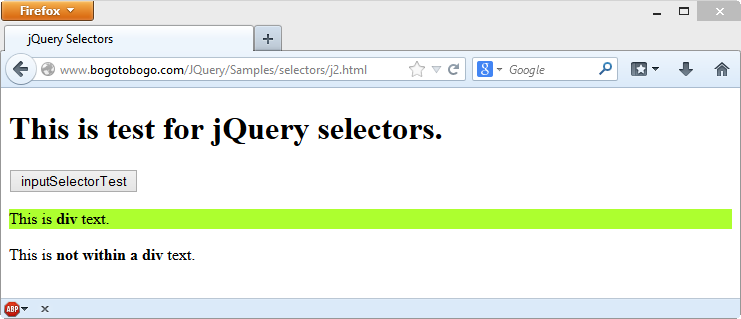
This time, we want to do the same thing but only do it to the 3rd child. Since we have only one child in the html, we need to add more lines j2B.html:
<!doctype html> <html> <head> <meta charset="utf-8" /> <title>jQuery Selectors</title> </head> <body> <h1>This is test for jQuery selectors. </h1> <input id="inputButton" type="button" value="inputSelectorTest"> <br /> <div> <p>This is the <b>first div</b> text.</p> <p>This is the <b>second div</b> text.</p> <p>This is the <b>third div</b> text.</p> </div> <p>This is the <b>first not within a div</b> text.</p> <p>This is the <b>second not within a div</b> text.</p> <p>This is the <b>third not within a div</b> text.</p> <script type="text/javascript" src="http://code.jquery.com/jquery-2.1.0.min.js""></script> <script type="text/javascript" src="jQuery_id_div_selector.js"></script> <script> </script> </body> </html>
To select only the 3rd child of p under div:
$(document ).ready(function() { // if the button is clicked, make the div element blue $('#inputButton').click(function(){ $('div > :nth-child(3)').css('background-color','powderblue'); }); });
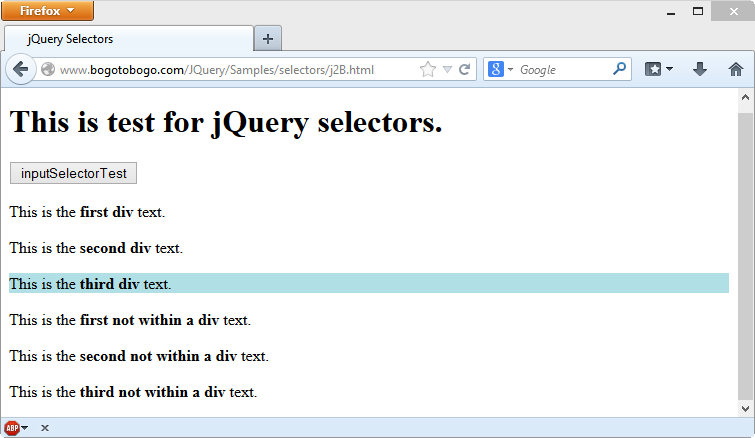
To select the even lines of p elements:
$('p:even').css('background-color','mistyrose');
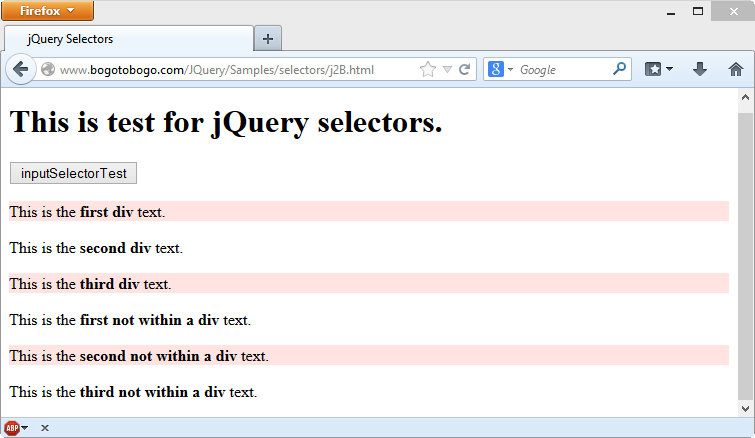
Note that it starts from 0.
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization