3. Events
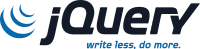
Events are usually triggered by the end user's interaction with the page such as text input or the mouse move or page load/unload events.
jQuery provides methods for most browser events such as .click(), .blur(), .focus(), .change(), etc. These are shorthand for jQuery's .on() method which is useful for binding the same handler function to multiple events, when we want to provide data to the event hander, when we are working with custom events, or when we want to pass an object of multiple events and handlers.
Here is our html file with 3 headings, j3_events.html:
<html> <head> <meta charset="utf-8" /> <title>jQuery Selectors</title> </head> <body> <h1>This is a test for jQuery events. </h1> <h1>1st heading</h1> <h1>2nd heading</h1> <h1>3rd heading</h1> <script type="text/javascript" src="http://code.jquery.com/jquery-2.1.0.min.js""></script> <script type="text/javascript" src="jQuery_events.js"></script> <script> </script> </body> </html>
The js file, jQuery_events.js:
$( document ).ready(function() { $('h1').click(function(){ $(this).css('backgroundColor', 'yellow'); }); });
Actually, we've done this in previous chapters. When a user clicks h1 text, the background of the line will be colored in yellow:
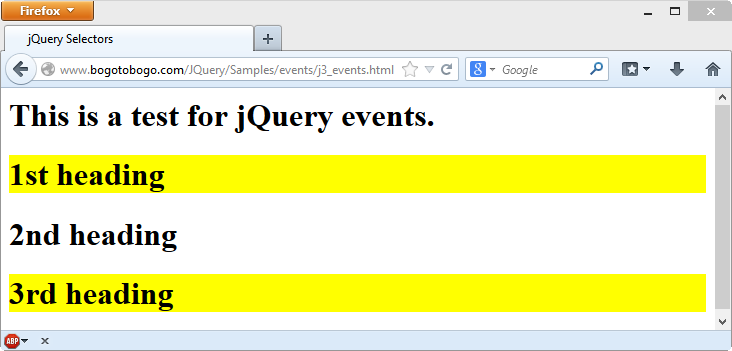
Let's play with the mouse events: when it enters into a h1 text, the background turns into yellow and when it leaves out of the text, the background turns into green.
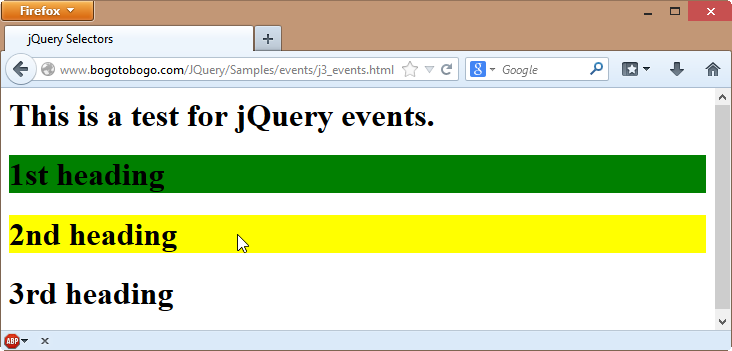
The js files looks like this:
$( document ).ready(function() { $('h1').mouseenter(function(){ $(this).css('backgroundColor', 'yellow'); }); $('h1').mouseleave(function(){ $(this).css('backgroundColor', 'green'); }); });
We can see the similar things happening for the mouse up/down events.
We can make some tweeks to the above example by unbinding the event from the selector. In other words, when we go back to the h1 text, it does not turn back into yellow for the mouseenter event but stays at the same green color as it left.
Here is a new js file:
$( document ).ready(function() { $('h1').mouseenter(function(){ $(this).css('backgroundColor', 'yellow'); }); $('h1').mouseleave(function(){ $(this).css('backgroundColor', 'green'); $(this).unbind(); }); });
Let's think about the following line which the this has been replaced with "*":
$( document ).ready(function() { $('h1').mouseenter(function(){ $(this).css('backgroundColor', 'yellow'); }); $('h1').mouseleave(function(){ $(this).css('backgroundColor', 'green'); $("*").unbind(); }); });
With the js file, it will work for once. In other words, suppose the mouse entered and left the first heading, then moved into the 2nd line, the enter/leave events were no longer bound to change the background color. All gone and only the first line remained in green.
Another thing we should know about the bind() method is that we can supply a specific event as an argument. For example, we can give mouseleave as a parameter to the bind().
$( document ).ready(function() { $('h1').mouseenter(function(){ $(this).css('backgroundColor', 'yellow'); }); $('h1').mouseleave(function(){ $(this).css('backgroundColor', 'green'); $("*").unbind("mouseleave"); }); });
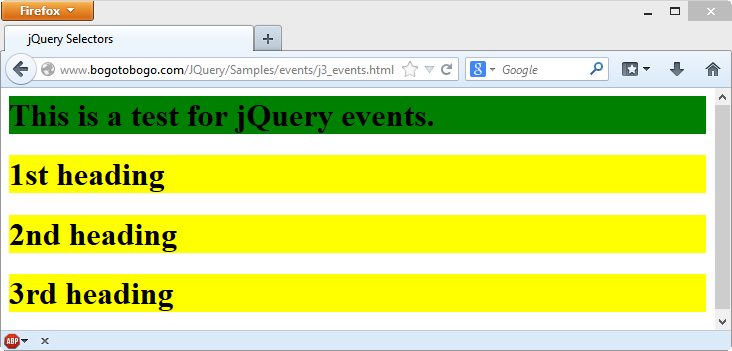
As we can see from the picture, the mouseleave works only for the first time, after that it no longer has any effect since it's been unbound. As a result, only the first one remained green. If we move the mouse on the title text, it will change the background color to yellow since the mouseenter still has bind().
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization