2B. Selectors
(class attribute and this keyword)
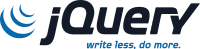
This is the continuation from 2A Selectors
In this chapter, we will see how we can use a class attribute as a selector. So, we need to modify the html file: j2_class.html.
<!doctype html> <html> <head> <meta charset="utf-8" /> <title>jQuery Selectors</title> </head> <body> <h1>This is test for jQuery selectors. </h1> <input id="inputButton" type="button" value="inputSelectorTest"> <br /> <div> <p>This is the <b>first div</b> text.</p> <p>This is the <b>second div</b> text.</p> <p class="classSelector">This is the <b>third div</b> text.</p> </div> <p>This is the <b>first not within a div</b> text.</p> <p>This is the <b class="classSelector">second not within a div</b> text.</p> <p>This is the <b>third not within a div</b> text.</p> <script type="text/javascript" src="http://code.jquery.com/jquery-2.1.0.min.js""></script> <script type="text/javascript" src="jQuery_class_selector.js"></script> <script> </script> </body> </html>
Since we put the class into the third line under div and the 2nd line that's not under div, the background of the first selection and the "b" portion of the second selection should be highlight when we click the button:
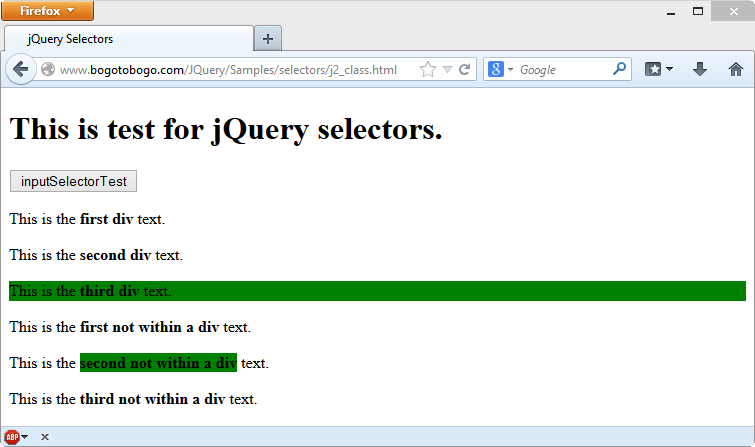
The js file should look like this:
$( document ).ready(function() { // if the button is clicked, make the div element blue $('#inputButton').click(function(){ // $('div').css('background-color','yellow'); // $('p').css('background-color','green'); // $('div, b, #inputButton').css('background-color','cyan'); // $('*').css('background-color','khaki'); // $('div > p').css('background-color','greenyellow'); // $('div > :nth-child(3)').css('background-color','powderblue'); // $('p:even').css('background-color','mistyrose'); $('.classSelector').css('background-color','green'); }); });
Note that we used dot('.') to select class in our js file.
If we want highlight only the 2nd cases of the previous example, we need to specify it by putting b infront of the class attribute in our js file:
$('b.classSelector').css('background-color','green');
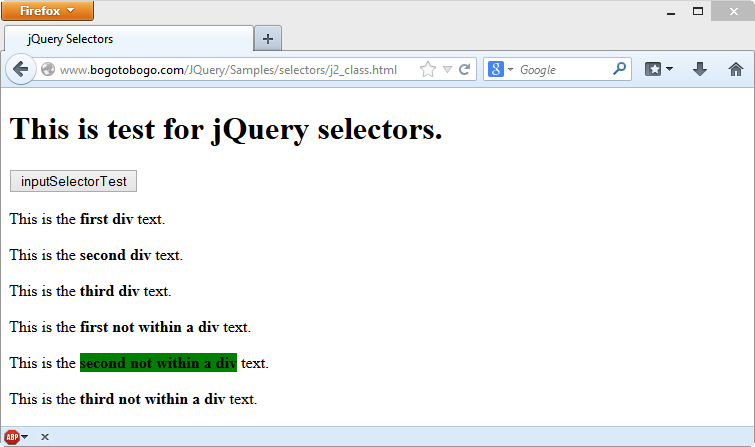
So far, the click event by the button was the only event that we can check. Now, we want not only the inputButton but also any <b> element to trigger event that can do some highlighting on a selected text. Also, we now want to use this selector as well. Since this is not a text string but a key word, we do not need to wrap with double quote(").
js file (jQuery_this_selector.js):
$( document ).ready(function() { // if the button is clicked, make the div element blue $('#inputButton, b').click(function(){ // $('div').css('background-color','yellow'); // $('p').css('background-color','green'); // $('div, b, #inputButton').css('background-color','cyan'); // $('*').css('background-color','khaki'); // $('div > p').css('background-color','greenyellow'); // $('div > :nth-child(3)').css('background-color','powderblue'); // $('p:even').css('background-color','mistyrose'); // $('.classSelector').css('background-color','green'); // $('b.classSelector').css('background-color','green'); $(this).css('background-color','green'); }); });
html file (j2_this_selector.html):
<!doctype html> <html> <head> <meta charset="utf-8" /> <title>jQuery Selectors</title> </head> <body> <h1>This is test for jQuery selectors. </h1> <input id="inputButton" type="button" value="inputSelectorTest"> <br /> <div> <p>This is the <b>first div</b> text.</p> <p>This is the <b>second div</b> text.</p> <p class="classSelector">This is the <b>third div</b> text.</p> </div> <p>This is the <b>first not within a div</b> text.</p> <p>This is the <b class="classSelector">second not within a div</b> text.</p> <p>This is the <b>third not within a div</b> text.</p> <script type="text/javascript" src="http://code.jquery.com/jquery-2.1.0.min.js""></script> <script type="text/javascript" src="jQuery_this_selector.js"></script> </body> </html>
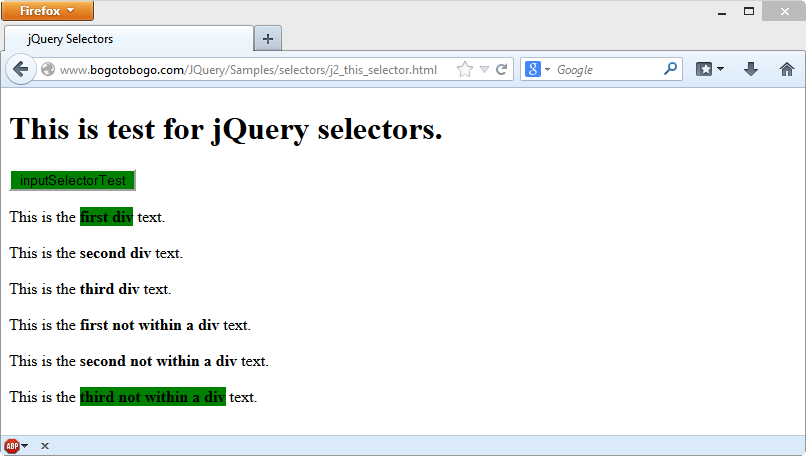
As we can see from the picture, whatever we click (b or inputButton), it will be highlight. The this selector is selecting the one just clicked.
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization