1. (document).ready()
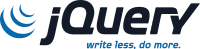
- One of the biggest challenges in JavaScript programming is cross-browser inconsistencies. With jQuery, most of the code we write will run exactly the same on all the major browsers.
- Javascript's experts provide us quick solution with jQuery. We do not have to write complicated javascripts any more.
In this chapter, we'll use a very simple html lines:
When we click "Click this to toggle the hidden text" (h1 tag), it will toggle a hidden text with i tag.
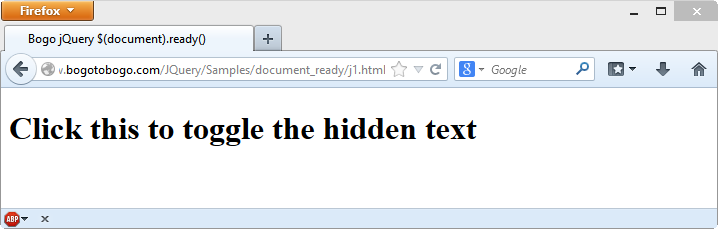
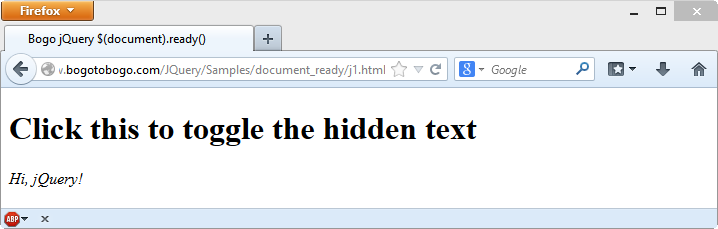
Let's start with the simplest jQuery:
$(document).ready();
We used to have the following code instead:
window.onload = function() { // code }
However, the code doesn't run until all loads (such as images and ads) are finished downloading. So, to run code as soon as the document is ready to be manipulated, we use jQuery's ready() event function.
More exact form looks like this:
$( document ).ready(function() { // our code here. });
With the ready(), we can run our javascript code as soon as our html document has been loaded.
Here is our js file: jQuery_document_ready.js:
$( document ).ready(function() { // hide the text with "i" tag $("i").hide(); // if a text with h1 tag is clicked, // the next will be toggled $("h1").click(function() { $(this).next().slideToggle(100); }); });
Here is the html file: j1.html:
<!doctype html> <html> <head> <meta charset="utf-8" /> <title>Bogo jQuery $(document).ready()</title> </head> <body> <h1>Click this to toggle the hidden text</h1> <i>Hi, jQuery!</i> <script type="text/javascript" src="http://code.jquery.com/jquery-2.1.0.min.js"></script> <script type="text/javascript" src="jQuery_document_ready.js"></script> <script> </script> </body> </html>
We have two js files in the j1.html file:
- http://code.jquery.com/jquery-2.1.0.min.js - we need this to use jQuery.
Or we can down load it from http://jquery.com/download/ and put the file in our local directory. In that case, we can refer it something like this:<script type="text/javascript" src="jquery-2.1.0.min.js"></script>
In either case, the src attribute in the <script> element must point to a copy of jQuery. - jQuery_document_ready.js - we need this to customize the behavior of our html.
Note that the order of the two js files should be the same as in the example.
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization