Active Records 2020
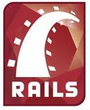
Active Record is the M in MVC.
Active Records are used in Ruby as a means of persisting data so that it can be recalled for later use even after we've closed the program that created the data.
The active Record pattern is used to access data stored in relational databases, and it allows us to perform CRUD operations without worrying about the specific underying database technology (SQLite, MySQL, or PostgresSQL, etc).
Most software application today are written using OO language, and often it is necessary to persist the objects associated with these applications.
However, the classes and objects associated with an OO language are incompatible with the structure of relational databases. Active Records' object-relational mapping (ORM) does a mapping between OO language constructs and relational database constructs.
The ORM provided by Active Records automatically converts object into constructs that can be stored in a database and converts them back upon retrieval. This creates, in effect, a 'virtual objct database' that can be used from within an OO language.
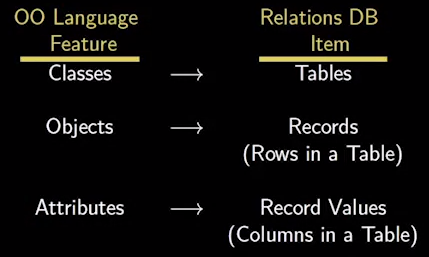
From class.coursera.org/webapplications.
Using the functionality provided by ActiveRecord module, we can do the followings:
- Establish a connection to a database.
- Create database tables.
- Specify associations between tables that correspond to associations between the Ruby classes.
- Establish an ORM between Ruby classes/objects/attributes and the tables/rows/columns in the underlying database.
- Perform CRUD operations on Ruby ActiveRecord objects.
The ActiveRecord module is built into Rails so that the functionalities listed above are utilized when we create a Rails app and run scaffold and model generators.
The ActiveRecord::Base.establish_connection method uses the information in ./config/database.yml in order to connect a Rails application to a database:
# SQLite version 3.x # gem install sqlite3 # # Ensure the SQLite 3 gem is defined in your Gemfile # gem 'sqlite3' # default: &default; adapter: sqlite3 pool: 5 timeout: 5000 development: <<: *default database: db/development.sqlite3 # Warning: The database defined as "test" will be erased and # re-generated from your development database when you run "rake". # Do not set this db to the same as development or production. test: <<: *default database: db/test.sqlite3 production: <<: *default database: db/production.sqlite3
The ActiveRecord::Migration object is used to incrementally evolve our database over time:
class CreatePosts < ActiveRecord::Migration def change create_table :posts do |t| t.string :title t.text :body t.timestamps end end end
The ActiveRecord::Schema.define method, in ./db/schema:
ActiveRecord::Schema.define(version: 20141031050956) do create_table "comments", force: true do |t| t.integer "post_id" t.text "body" t.datetime "created_at" t.datetime "updated_at" end create_table "posts", force: true do |t| t.string "title" t.text "body" t.datetime "created_at" t.datetime "updated_at" end endis created by inspecting the database and then expressing its structure programmically using a portable (database-independent) DSL (Domain Specific Language). This can be loaded into any database that ActiveRecord supports.
By default, Active Record uses some naming conventions to find out how the mapping between models and database tables should be created.
Rails will pluralize our class names to find the respective database table. So, for a class Book, we should have a database table called books.
The Rails pluralization mechanisms are very powerful, being capable to pluralize (and singularize) both regular and irregular words.
When using class names composed of two or more words, the model class name should follow the Ruby conventions, using the CamelCase form, while the table name must contain the words separated by underscores.
Examples:
- Database Table - Plural with underscores separating words (e.g., book_clubs).
- Model Class - Singular with the first letter of each word capitalized (e.g., BookClub).
For more info, please check Active Record Basics.
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization