Microblog App 5 - Microposts resource II 2020
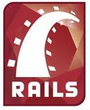
In our User model, each user can have many microposts as shown in the code below (app/models/user.rb):
class User < ActiveRecord::Base has_many :microposts end
Note that the name of the other model (microposts) is pluralized when declaring a has_many association.
We'll soon find this association on the "other side" of a belongs_to association. This association indicates that each instance of the model(user) has zero or more instances of another model (micropost).
A micropost belongs to a user (app/models/micropost.rb):
class Micropost < ActiveRecord::Base belongs_to :user validates :content, length: { maximum: 140 } end
So, why Associations?
Why do we need associations between models? Because they make common operations simpler and easier in our code.
As shown in the above example, we have a very simple Rails application that includes a model for users and a model for mocroposts. Each users can have many mocroposts.
For additional info about "Why", please visit Active Record Associations.
$ rails console Running via Spring preloader in process 16452 Loading development environment (Rails 4.2.5.1) irb(main):001:0> u = User.first User Load (0.7ms) SELECT "users".* FROM "users" ORDER BY "users"."id" ASC LIMIT 1 => #<User id: 1, name: "K Hong", email: "k@bogotobogo.com", created_at: "2016-01-20 04:31:48", updated_at: "2016-01-20 06:24:08"> irb(main):002:0> u.microposts Micropost Load (0.9ms) SELECT "microposts".* FROM "microposts" WHERE "microposts"."user_id" = ? [["user_id", 1]] => #<ActiveRecord::Associations::CollectionProxy [#<Micropost id: 1, content: "First post", user_id: 1, created_at: "2016-01-23 02:32:28", updated_at: "2016-01-23 02:32:28">, #<Micropost id: 2, content: "Second post", user_id: 1, created_at: "2016-01-23 02:32:54", updated_at: "2016-05-23 02:32:54">, #<Micropost id: 3, content: "bogotobogo - micropost 1, bogotobogo - micropost 2...", user_id: 1, created_at: "2016-01-23 03:29:28", updated_at: "2016-01-23 03:29:28">]>
We invoked the console with rails console at the command line, and then retrieved the first user from the database using User.first.
Here we have accessed the user's microposts, u.microposts.
Active Record automatically returns all the microposts with user_id equal to the id of u.
As we can see from the diagram below, both the User model and the Micropost model inherit from ActiveRecord::Base, which is the base class for models provided by ActiveRecord.
By inheriting from ActiveRecord::Base, our model objects communicate with the database.
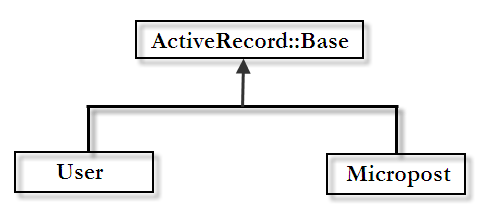
app/models/user.rb
class User < ActiveRecord::Base ... end
app/models/micropost.rb
class Micropost < ActiveRecord::Base ... end
The diagram shows the inheritance structure for controllers: both the Users controller and the Microposts controller inherit from the Application controller which inherits from ActionController::Base.
The ActionController::Base is the base class for controllers provided by the Rails library Action Pack.
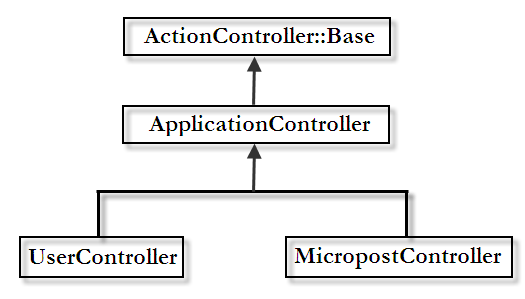
app/controllers/users_controller.rb
class UsersController < ApplicationController ... end
app/controllers/microposts_controller.rb
class MicropostsController < ApplicationController ... end
app/controllers/application_controller.rb
class ApplicationController < ActionController::Base ... end
By inheriting from ActionController::Base, both the Users and Microposts controllers have variety of features, such as rendering views as HTML, filtering inbound HTTP requests, manipulating model objects.
Since all Rails controllers inherit from ApplicationController, rules defined in the Application controller automatically apply to every action in the application.
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization