Ruby - Condition (if), Operators (comparison/logical) & case statement 2020
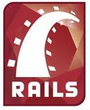
bogotobogo.com site search:
if-elsif-else-end
Let's learn how to use conditional statement in Ruby:
#!/usr/bin/ruby # r9.rb # if - elsif - else - end age = 12 if (age <= 2) puts "You're a baby" elsif (age > 2 ) && (age < 12 ) puts "You're a kid" else puts "You're almost there" end
Output:
$ ./r9.rb You're almost there
Comparison operators
Here are the common operators in Ruby.
==, !=, > , <, =>, <=
Other comparison operators are:
Operator | Description | Example |
---|---|---|
<=> | Combined comparison operator. Returns 0 if first operand equals second, 1 if first operand is greater than the second and -1 if first operand is less than the second. | (10 <=> 20) returns -1 |
=== | Used to test equality within a when clause of a case statement.. | (1...10) === 5 returns true |
.eql? | True if the receiver and argument have both the same type and equal values. | 1 == 1.0 returns true, but 1.eql?(1.0) is false |
equal? | True if the receiver and argument have the same object id. | a=10, b=20: if aObj is duplicate of bObj then aObj == bObj is true, a.equal?bObj is false but a.equal?aObj is true. |
Another comparison operator, unless. It is just if in reverse. It's a conditional statement that executes only if the condition is false:
#!/usr/bin/ruby # r10.rb # comparison operators : unless age = 12 unless age > 5 puts "No school" else puts "Go to school" end
Output:
$ ./r10.rb Go to school
Logical operators
#!/usr/bin/ruby # r11.rb # logical operators puts "true && false = " + (true && false).to_s puts "true || false = " + (true || false).to_s puts "!true = " + (!true).to_s
Output:
$ ./r11.rb true && false = false true || false = true !true = false
case
#!/usr/bin/ruby # r12.rb # case statement number = gets.chomp # remove a new line case number when "one", "uno" puts "1" exit when "two", "dos" puts "2" exit else puts "too big" end
Output:
$ ./r12.rb dos 2 k@laptop:~/TEST/RUBY$ ./r12.rb 10 too big
Ternary condition
#!/usr/bin/ruby # r13.rb # ternary condition age = 86 puts (age >= 70? "old" : "still young")
Output:
$ ./r13.rb old
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization