Ruby Symbols (:) 2020
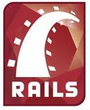
Symbol is the most basic Ruby object we can create. It's just a name and an internal ID. Since a given symbol name refers to the same object throughout a Ruby program, Symbols are useful and more efficient than strings. Two strings with the same contents are two different objects, but for any given name, there is only one Symbol object. This can save both time and memory.
A symbol appears to be a variable name but it's prefixed with a colon (:) such as :action, :items, and :id.
We don't need to pre-declare a symbol. They are guaranteed to be unique. There's no need to assign some kind of value to a symbol since Ruby takes care of that for us. Ruby also guarantees that no matter where it appears in our program, a particular symbol will have the same value.
In other words, we can consider the colon to mean a named thing, so :id is a thing named id.
- :id as the name of the variable id.
- plain id as the value of the variable.
#!/usr/bin/env ruby # sym1.rb puts "string".object_id puts "string".object_id puts :item.object_id puts :item.object_id
Output: 17960080 17960020 357448 357448
The rule of thumb - which one to use, variable or symbol?
- If the contents (the sequence of characters) of the object are important, we use a string.
- If the identity of the object is important, we use a symbol.
Ruby maintains a symbol table to hold them. Symbols are names - names of instance variables, methods, classes. So if there is a method called update_blog, there is automatically a symbol :update_blog. Ruby keeps its symbol table handy at all times, so can find out what's on it at any time by calling Symbol.all_symbols.
A Symbol object is created by prefixing an operator, string, variable, constant, method, class name with a colon (:). The symbol object will be unique for each different name but does not refer to a particular instance of the name, for the duration of a program's execution. Thus, while Sym can be a constant in one context, it also can be a method or a class in different context, the Symbol :Sym will be the same object in all three contexts.
#!/usr/bin/env ruby # sym2.rb class Blog puts :Blog.object_id.to_s def blog puts :blog.object_id.to_s @blog = 99 puts :blog.object_id.to_s end end b = Blog.new b.blog
Output:
357548 357608 357608
We can also transform a string into a symbol and vice-versa:
puts "string".to_sym.class # symbol puts :symbol.to_s.class # string
Ruby on Rails
- Ruby On Rails Home
- Ruby - Input/Output, Objects, Load
- Ruby - Condition (if), Operators (comparison/logical) & case statement
- Ruby - loop, while, until, for, each, (..)
- Ruby - Functions
- Ruby - Exceptions (raise/rescue)
- Ruby - Strings (single quote vs double quote, multiline string - EOM, concatenation, substring, include, index, strip, justification, chop, chomp, split)
- Ruby - Class and Instance Variables
- Ruby - Class and Instance Variables II
- Ruby - Modules
- Ruby - Iterator : each
- Ruby - Symbols (:)
- Ruby - Hashes (aka associative arrays, maps, or dictionaries)
- Ruby - Arrays
- Ruby - Enumerables
- Ruby - Filess
- Ruby - code blocks and yield
- Rails - Embedded Ruby (ERb) and Rails html
- Rails - Partial template
- Rails - HTML Helpers (link_to, imag_tag, and form_for)
- Layouts and Rendering I - yield, content_for, content_for?
- Layouts and Rendering II - asset tag helpers, stylesheet_link_tag, javascript_include_tag
- Rails Project
- Rails - Hello World
- Rails - MVC and ActionController
- Rails - Parameters (hash, array, JSON, routing, and strong parameter)
- Filters and controller actions - before_action, skip_before_action
- The simplest app - Rails default page on a Shared Host
- Redmine Install on a Shared Host
- Git and BitBucket
- Deploying Rails 4 to Heroku
- Scaffold: A quickest way of building a blog with posts and comments
- Databases and migration
- Active Record
- Microblog 1
- Microblog 2
- Microblog 3 (Users resource)
- Microblog 4 (Microposts resource I)
- Microblog 5 (Microposts resource II)
- Simple_app I - rails html pages
- Simple_app II - TDD (Home/Help page)
- Simple_app III - TDD (About page)
- Simple_app IV - TDD (Dynamic Pages)
- Simple_app V - TDD (Dynamic Pages - Embedded Ruby)
- Simple_app VI - TDD (Dynamic Pages - Embedded Ruby, Layouts)
- App : Facebook and Twitter Authentication using Omniauth oauth2
- Authentication and sending confirmation email using Devise
- Adding custom fields to Devise User model and Customization
- Devise Customization 2. views/users
- Rails Heroku Deploy - Authentication and sending confirmation email using Devise
- Deploying a Rails 4 app on CentOS 7 production server with Apache and Passenger I
- Deploying a Rails 4 app on CentOS 7 production server with Apache and Passenger II
- OOPS! Deploying a Rails 4 app on CentOS 7 production server with Apache and Passenger (Trouble shooting)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization