Model View Controller (MVC) 2020
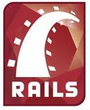
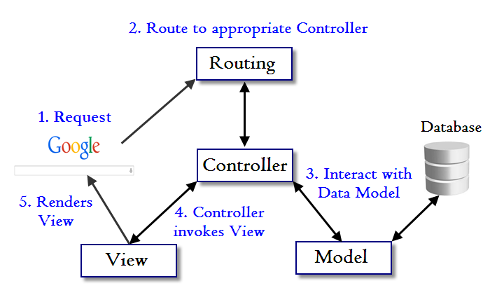
When interacting with our application, a browser sends a request, which is received by a web server and passed on to the Rails routing engine. The router receives the request and redirects to the appropriate controller class method based on the routing URL pattern.
The controller then takes over. In some cases, the controller will immediately render a view for the browser. More commonly for dynamic sites, the controller interacts with a model.
After invoking the model, the controller then renders the final view (HTML, CSS, and images) and returns the complete web page to the user's browser.
Rails has an application directory called app/ with three subdirectories: models, views, and controllers. This is the model-view-controller (MVC) architectural pattern, which enforces a separation between business logic from the input and presentation logic associated with a graphical user interface (GUI).
In the case of web applications, the business logic typically consists of data models for things like users, articles, and products, and the GUI is just a web page in a web browser.
When we first create an app, for example, DemoControllerApp, there is not much in our app/controllers directory. We only have application_controller.rb which has the following in it:
class ApplicationController < ActionController::Base # Prevent CSRF attacks by raising an exception. # For APIs, you may want to use :null_session instead. protect_from_forgery with: :exception end
ApplicationController inherits from ActionController::Base, which defines a number of helpful methods.
Suppose we want to create a users controller and corresponding user views, we run rails g controller users in our command line.
$ rails g controller users
This will create a users_controller.rb in our controllers directory, and will allow us to set up additional routes for our user model. This will also create users in our views directory.
What's in the users_controller.rb?
class UsersController < ApplicationController end
A controller is a Ruby class which inherits from ApplicationController and has methods just like any other class. When our application receives a request, the routing will determine which controller and action to run, then Rails creates an instance of that controller and runs the method with the same name as the action.
Let's define a new method. Just the empty definition:
class UsersController < ApplicationController def new end end
As an example, if a user goes to /users/new in our application to edit a profile, Rails will create an instance of UsersController and run the new method. Note that the empty method from the example above would work just fine because Rails will by default render the edit.html.erb view unless the action says otherwise.
The new method could make available to the view a @user instance variable by creating a new User:
class UsersController < ApplicationController def new @user = User.new end end
Only public methods are callable as actions. It is a best practice to lower the visibility of methods which are not intended to be actions, like auxiliary methods or filters.- Action Controller Overview.
Basic Rails routing is relying on controller and its actions. In other words, the Rails router recognizes URLs and dispatches them to a controller's action.
But sometimes we may need different approach. For example, to make a link to another page with the same controller. Especially, the page does not have any VERB actions.
It may look ugly and they are not RESTful controllers. So, I do not like it, but so far that's the only way I found.
In this example, I have a controller called googleplus and I want to have lots of pages under views/googleplus folder. How can I construct links to those pages?
Here it is:
app/controllers/googleplus_controller.rb:
class GoogleplusController < ApplicationController skip_authorization_check skip_before_action :authenticate_user! def index end def secondpage end end
config/routes.rb:
get '/googleplus' => 'googleplus#home', as: 'googleplus' get '/googleplus/secondpage'
Suppose, we have create 2 files in our views:
app/views/googleplus/index.html.erb app/views/googleplus/secondpage.html.erb
Then, we can create links with:
<%= link_to 'Page 1', googleplus_path %> <%= link_to 'Page 2', googleplus_secondpage_path %>
Bogotobogo's contents
To see more items, click left or right arrow.
Bogotobogo Image / Video Processing
Computer Vision & Machine Learning
with OpenCV, MATLAB, FFmpeg, and scikit-learn.
Bogotobogo's Video Streaming Technology
with FFmpeg, HLS, MPEG-DASH, H.265 (HEVC)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization