Simple App 1 2020
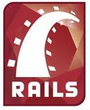
We need to create a new Rails project, this time called simple_app:
$ cd ~/rails_projects $ rails new simple_app --skip-test-unit $ cd simple_app
The --skip-test-unit option to the rails command tells Rails not to generate a test directory associated with the default Test::Unit framework.
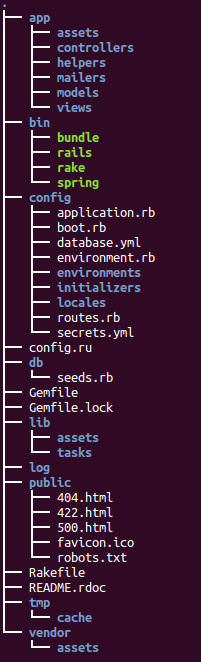
We need to update the Gemfile with the gems needed by our application. On the other hand, for our simple application we need two gems: the gem for RSpec and the gem for the RSpec library specific to Rails.
... group :development, :test do gem 'rspec-rails', '2.14.1' end group :test do gem 'selenium-webdriver', '2.35.1' gem 'capybara', '2.1.0' end group :production do gem 'pg', '0.15.1' gem 'rails_12factor', '0.0.2' end ...
The file includes rspec-rails in a development environment so that we have access to RSpec-specific generators, and it includes it in test mode in order to run the tests. We donât have to install RSpec itself because it is a dependency of rspec-rails and will thus be installed automatically. We also include the Capybara gem, which allows us to simulate a userâs interaction with the sample application using a natural English-like syntax, together with Selenium, one of Capybaraâs dependencies.
We also include the PostgreSQL and static assets gems in production for deployment.
To install and include the new gems, we run bundle update and bundle install:
$ bundle install --without production $ bundle update $ bundle install
We'll use a generate script to make controllers. Since we'll be making a controller to handle static pages, we'll call it the StaticPages controller. We'll also plan to make actions for a Home page, a Help page, and an About page. The generate script takes an optional list of actions, so we'll include two of the initial actions directly on the command line.
$ rails generate controller StaticPages home help --no-test-framework create app/controllers/static_pages_controller.rb route get 'static_pages/help' route get 'static_pages/home' invoke erb create app/views/static_pages create app/views/static_pages/home.html.erb create app/views/static_pages/help.html.erb invoke helper create app/helpers/static_pages_helper.rb invoke assets invoke coffee create app/assets/javascripts/static_pages.js.coffee invoke scss create app/assets/stylesheets/static_pages.css.scss
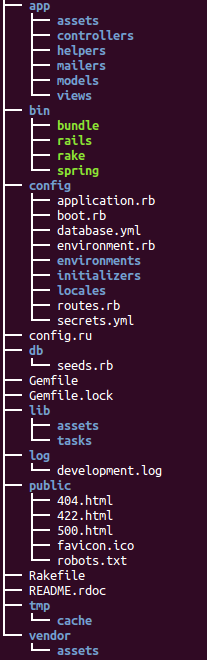
Since we generated home and help actions, the routes file already has a rule for each one:
config/routes.rb:
Rails.application.routes.draw do get 'static_pages/home' get 'static_pages/help' ... end
The rule get "static_pages/home" maps requests for the URL /static_pages/home to the home action in the StaticPages controller. Also, by using get we arrange for the route to respond to a GET request. In other words, we generate a home action inside the StaticPages controller we automatically get a page at the address /static_pages/home:
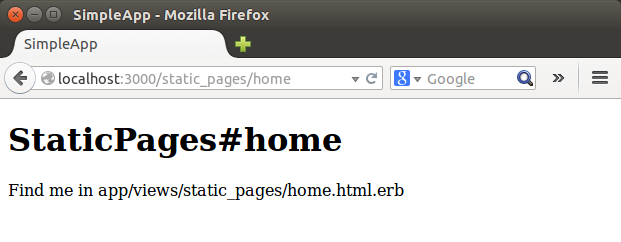
app/controllers/static_pages_controller.rb
class StaticPagesController < ApplicationController def home end def help end end
when visiting the URL /static_pages/home, Rails looks in the StaticPages controller and executes the code in the home action, and then renders the view corresponding to the action. In our case, the home action is empty, so all visiting /static_pages/home does is render the view (home.html.erb):
app/views/static_pages/home.html.erb
<h1>StaticPages#home</h1> <p>Find me in app/views/static_pages/home.html.erb</p>
app/views/static_pages/help.html.erb
<h1>StaticPages#help</h1> <p>Find me in app/views/static_pages/help.html.erb</p>
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization