Ruby Input/Output, Objects, Load 2020
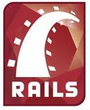
bogotobogo.com site search:
Input (gets) / output (puts)
Let's learn how to get some input from a user and how to print it:
# r1.rb # Reading input & printing output print "Enter a number:" num1 = gets.to_i print "Enter another number:" num2 = gets.to_i puts num1.to_s + " + " + num2.to_s + " = " + (num1+num2).to_s
Output:
$ ruby r1.rb Enter a number:10 Enter another number:20 10 + 20 = 30
Note that we used to_i and to_s for get method to convert a string to int or integer to string.
We can run the script as we do with shell script.
#!/usr/bin/ruby # r1.rb ...
Then:
$ chmod +x r1.rb $ ./r1.rb
5 arithmetic operators
#!/usr/bin/ruby # r2.rb # 5 Arithmetic operators puts "7 + 3 = " + (7+3).to_s puts "7 - 3 = " + (7-3).to_s puts "7 * 3 = " + (7*3).to_s puts "7 / 3 = " + (7/3).to_s puts "7 % 3 = " + (7%3).to_s
Output:
$ ./r2.rb 7 + 3 = 10 7 - 3 = 4 7 * 3 = 21 7 / 3 = 2 7 % 3 = 1
float
#!/usr/bin/ruby # r3.rb # floating point numbers num_one = 1.000 num_0999 = 0.999 puts "num_one - num_0999 = " + (num_one-num_0999).to_s
Output:
$ ./r3.rb num_one - num_0999 = 0.0010000000000000009
Object
Basically, everything in Ruby is an object.
#!/usr/bin/ruby # r4.rb # Objects puts 1.class puts 1.0123.class puts "A string".class
Output:
./r4.rb Fixnum Float String
File read/write
We can create a file like this:
#!/usr/bin/ruby # r6.rb # File write f = File.new("myfile.out","w") f.puts("Test") f.close
Output:
$ cat myfile.out Test
We can read from the file we created:
#!/usr/bin/ruby # r7.rb # File write / read f = File.new("myfile.out","w") f.puts("Test") f.close data = File.read("myfile.out") puts "reading from a file data = " + data
Output:
$ ./r7.rb reading from a file data = Test
Loading a Ruby file
We can load a Ruby file:
$ cat my_ruby.rb puts "Testing loading a 'rb' file"
Here is our code:
#!/usr/bin/ruby # r8.rb # loading 'rb' file load "my_ruby.rb"
Output:
$ ./r8.rb Testing loading a 'rb' file
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization