Ruby - loop, while, until, for, each, (..) 2020
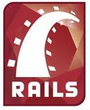
bogotobogo.com site search:
loop
Let's print out even numbers up to 10. We'll use loop:
#!/usr/bin/ruby # loop.rb # How to loop n = 0 loop do n += 1 next unless (n % 2) == 0 break if n > 10 puts n end
Output:
$ ./loop.rb 2 4 6 8 10
while
Let's print out even numbers up to 10. This time, we'll use while:
#!/usr/bin/ruby # while.rb # How to while n = 0 while (n <= 10) n += 1 next unless (n % 2) == 0 puts n end
Output:
$ ./while.rb 2 4 6 8 10
until
Let's print out even numbers up to 10. This time, we'll use until:
#!/usr/bin/ruby # until.rb # How to until n = 0 until (n > 10) n += 1 next unless (n % 2) == 0 puts n end
Output:
$ ./until.rb 2 4 6 8 10
for
Let's print out even numbers up to 10. This time, we'll use for:
#!/usr/bin/ruby # for.rb # How to for list = [0,1,2,3,4,5,6,7,8,9,10] for n in list next unless (n % 2) == 0 puts "#{n}" end
Output:
$ ./for.rb 0 2 4 6 8 10
If we want the output in one line, we can use print instead:
#!/usr/bin/ruby # for2.rb # How to for list = [0,1,2,3,4,5,6,7,8,9,10] for n in list next unless (n % 2) == 0 print "#{n} " end
Output:
$ ./for2.rb 0 2 4 6 8 10
each
Let's print out even numbers up to 10. This time, we'll use each:
#!/usr/bin/ruby # each.rb # How to each list = [0,1,2,3,4,5,6,7,8,9,10] list.each do |n| next unless (n % 2) == 0 print "#{n}, " end
Output:
$ ./each.rb 0, 2, 4, 6, 8, 10,
We can simplify a little bit by using range(n1..n10)
#!/usr/bin/ruby # range.rb # How to range (0..10).each do |n| next unless (n % 2) == 0 print "#{n}, " end
Output:
$ ./range.rb 0, 2, 4, 6, 8, 10,
Ruby on Rails
- Ruby On Rails Home
- Ruby - Input/Output, Objects, Load
- Ruby - Condition (if), Operators (comparison/logical) & case statement
- Ruby - loop, while, until, for, each, (..)
- Ruby - Functions
- Ruby - Exceptions (raise/rescue)
- Ruby - Strings (single quote vs double quote, multiline string - EOM, concatenation, substring, include, index, strip, justification, chop, chomp, split)
- Ruby - Class and Instance Variables
- Ruby - Class and Instance Variables II
- Ruby - Modules
- Ruby - Iterator : each
- Ruby - Symbols (:)
- Ruby - Hashes (aka associative arrays, maps, or dictionaries)
- Ruby - Arrays
- Ruby - Enumerables
- Ruby - Filess
- Ruby - code blocks and yield
- Rails - Embedded Ruby (ERb) and Rails html
- Rails - Partial template
- Rails - HTML Helpers (link_to, imag_tag, and form_for)
- Layouts and Rendering I - yield, content_for, content_for?
- Layouts and Rendering II - asset tag helpers, stylesheet_link_tag, javascript_include_tag
- Rails Project
- Rails - Hello World
- Rails - MVC and ActionController
- Rails - Parameters (hash, array, JSON, routing, and strong parameter)
- Filters and controller actions - before_action, skip_before_action
- The simplest app - Rails default page on a Shared Host
- Redmine Install on a Shared Host
- Git and BitBucket
- Deploying Rails 4 to Heroku
- Scaffold: A quickest way of building a blog with posts and comments
- Databases and migration
- Active Record
- Microblog 1
- Microblog 2
- Microblog 3 (Users resource)
- Microblog 4 (Microposts resource I)
- Microblog 5 (Microposts resource II)
- Simple_app I - rails html pages
- Simple_app II - TDD (Home/Help page)
- Simple_app III - TDD (About page)
- Simple_app IV - TDD (Dynamic Pages)
- Simple_app V - TDD (Dynamic Pages - Embedded Ruby)
- Simple_app VI - TDD (Dynamic Pages - Embedded Ruby, Layouts)
- App : Facebook and Twitter Authentication using Omniauth oauth2
- Authentication and sending confirmation email using Devise
- Adding custom fields to Devise User model and Customization
- Devise Customization 2. views/users
- Rails Heroku Deploy - Authentication and sending confirmation email using Devise
- Deploying a Rails 4 app on CentOS 7 production server with Apache and Passenger I
- Deploying a Rails 4 app on CentOS 7 production server with Apache and Passenger II
- OOPS! Deploying a Rails 4 app on CentOS 7 production server with Apache and Passenger (Trouble shooting)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization