Ruby - Functions 2020
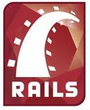
bogotobogo.com site search:
function 1
In this article, we'll learn how to use functions:
#!/usr/bin/ruby # f1.rb # How to function def addition(n1, n2) return n1.to_i + n2.to_i end puts addition(3,9)
Output:
$ ./f1.rb 12
Note that we cannot change global variable within a function:
#!/usr/bin/ruby # f2.rb # How to function x = 10 def change(x) x=99 end change(x) puts "x = #{x}"
Output:
$ ./f2.rb x = 10
As we can see from the output, the variable x retains its value after the function call.
Binary serach
As a practice, here is a code for binary search:
#!/usr/bin/ruby # bin.rb def bin(a,n) s = 0 e = a.length - 1 mid = (s + e)/2 while ( mid >= s and mid <= e) if (n < a[mid]) e = mid - 1 elsif (n > a[mid]) s = mid + 1 else return true end mid = (s + e)/2 end return false end # input arr = [1,3,5,7,9,11,13,15,17,19] # try find = [1,9,17,0,21,8] find.each do |item| puts bin(arr,item) end
Output:
true true true false false false
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization