Ruby Hashes 2020
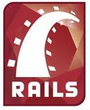
Hashes (aka associative arrays, maps, or dictionaries) are similar to arrays because they can be indexed collection of object references. However, while we index arrays with integers, we can index a hash with objects of any types. When we store a value in a hash, we're actually supplying two objects: the index (key) and the value pair. We can retrieve the value by indexing the hash with the same key.
The following example shows the use of hash literals: a list of key=>value pair between braces:
#!/usr/bin/env ruby # hash1.rb number = {'uno' => 'one', 'dos' => 'two', 'tres' => 'three'} puts number.length puts number['uno'] puts number
Output:
3 one {"uno"=>"one", "dos"=>"two", "tres"=>"three"}
Hashes have one significant advantage over arrays: they can use any object as an index. Hashes have a default value. This value is returned when an attempt is made to access keys that do not exist in the hash. By default this value is nil.
We may want to use a symbol for a key if not a quoted string.
#!/usr/bin/env ruby # hash2.rb number = Hash.new number[:uno] = 'One' number[:dos] = 'Two' number[:tres] = 'Three' puts number[:tres] number2 = {:uno=>'one', :dos=>'two', :tres=>'three'} puts number2
Output:
Three {:uno=>"one", :dos=>"two", :tres=>"three"}
We can use the following which gives us the same output:
#!/usr/bin/env ruby # hash3.rb number3 = {uno:'one', dos:'two', tres:'three'} puts number3
Output:
{:uno=>"one", :dos=>"two", :tres=>"three"}
However, for a numeric key, sym: syntax will not work:
not_ok= {1: 'uno'} # will not work ok = {1 => 'uno'} # will work
Here is a code doing loop with the hash:
#!/usr/bin/ruby # hash4.rb numbers = {uno:'one', dos:'two', tres:'three'} numbers.each do |k,v| puts k.to_s + ':' + v end
Output:
uno:one dos:two tres:three
Other functions of hash:
#!/usr/bin/ruby # hash5.rb numbers = {'uno'=>'one', 'dos'=>'two', 'tres'=>'three'} puts "Has numers 'uno' key? : " + numbers.has_key?('uno').to_s puts "Has numers 'two' value? : " + numbers.has_value?('two').to_s puts "Is numers is empty? : " + numbers.empty?.to_s puts "Size of numers? : " + numbers.size.to_s numbers.delete('tres') puts "Size of numers? : " + numbers.size.to_s
Output:
Has numers 'uno' key? : true Has numers 'two' value? : true Is numers is empty? : false Size of numers? : 3 Size of numers? : 2
Ruby on Rails
- Ruby On Rails Home
- Ruby - Input/Output, Objects, Load
- Ruby - Condition (if), Operators (comparison/logical) & case statement
- Ruby - loop, while, until, for, each, (..)
- Ruby - Functions
- Ruby - Exceptions (raise/rescue)
- Ruby - Strings (single quote vs double quote, multiline string - EOM, concatenation, substring, include, index, strip, justification, chop, chomp, split)
- Ruby - Class and Instance Variables
- Ruby - Class and Instance Variables II
- Ruby - Modules
- Ruby - Iterator : each
- Ruby - Symbols (:)
- Ruby - Hashes (aka associative arrays, maps, or dictionaries)
- Ruby - Arrays
- Ruby - Enumerables
- Ruby - Filess
- Ruby - code blocks and yield
- Rails - Embedded Ruby (ERb) and Rails html
- Rails - Partial template
- Rails - HTML Helpers (link_to, imag_tag, and form_for)
- Layouts and Rendering I - yield, content_for, content_for?
- Layouts and Rendering II - asset tag helpers, stylesheet_link_tag, javascript_include_tag
- Rails Project
- Rails - Hello World
- Rails - MVC and ActionController
- Rails - Parameters (hash, array, JSON, routing, and strong parameter)
- Filters and controller actions - before_action, skip_before_action
- The simplest app - Rails default page on a Shared Host
- Redmine Install on a Shared Host
- Git and BitBucket
- Deploying Rails 4 to Heroku
- Scaffold: A quickest way of building a blog with posts and comments
- Databases and migration
- Active Record
- Microblog 1
- Microblog 2
- Microblog 3 (Users resource)
- Microblog 4 (Microposts resource I)
- Microblog 5 (Microposts resource II)
- Simple_app I - rails html pages
- Simple_app II - TDD (Home/Help page)
- Simple_app III - TDD (About page)
- Simple_app IV - TDD (Dynamic Pages)
- Simple_app V - TDD (Dynamic Pages - Embedded Ruby)
- Simple_app VI - TDD (Dynamic Pages - Embedded Ruby, Layouts)
- App : Facebook and Twitter Authentication using Omniauth oauth2
- Authentication and sending confirmation email using Devise
- Adding custom fields to Devise User model and Customization
- Devise Customization 2. views/users
- Rails Heroku Deploy - Authentication and sending confirmation email using Devise
- Deploying a Rails 4 app on CentOS 7 production server with Apache and Passenger I
- Deploying a Rails 4 app on CentOS 7 production server with Apache and Passenger II
- OOPS! Deploying a Rails 4 app on CentOS 7 production server with Apache and Passenger (Trouble shooting)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization