Simple App III (TDD - About page) 2020
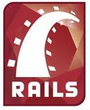
We've made Home and Help page using rails generate command in the previous chapter (Simple_app II - TDD (Home/Help)). In the chapter, we'll add About page using step by step TDD approach (by writing a test and running RSpec at each step).
Here is a code to test the contents of the About page (spec/requests/static_pages_spec.rb):
require 'spec_helper' describe "Static pages" do describe "Home page" do it "should have the content 'Simple App'" do visit '/static_pages/home' expect(page).to have_content('Simple App') end end describe "Help page" do it "should have the content 'Help'" do visit '/static_pages/help' expect(page).to have_content('Help') end end describe "About page" do it "should have the content 'About Us'" do visit '/static_pages/about' expect(page).to have_content('About Us') end end end
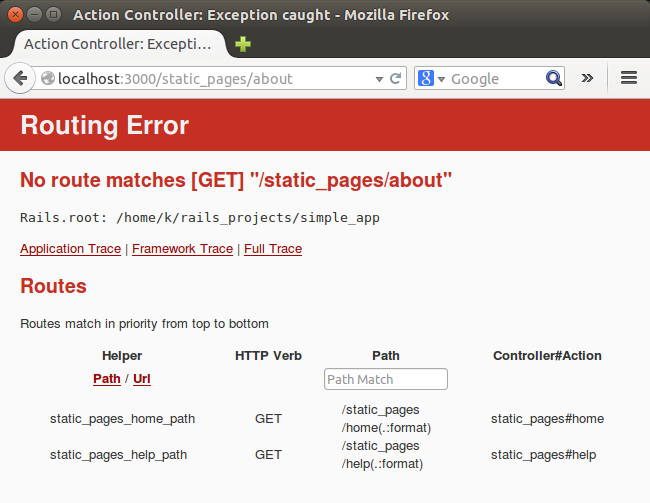
If we run the RSpec example using:
$ bundle exec rspec spec/requests/static_pages_spec.rb
the output includes the following complaint:
Failures: 1) Static pages About page should have the content 'About Us' Failure/Error: visit '/static_pages/about' ActionController::RoutingError: No route matches [GET] "/static_pages/about" # ./spec/requests/static_pages_spec.rb:24:in `block (3 levels) in <top (required)>'
So, we need to add /static_pages/about to the routes file (config/routes.rb):
Rails.application.routes.draw do get 'static_pages/home' get 'static_pages/help' get 'static_pages/about' ... end
If we re-run:
$ bundle exec rspec spec/requests/static_pages_spec.rb
It complains:
1) Static pages About page should have the content 'About Us' Failure/Error: visit '/static_pages/about' AbstractController::ActionNotFound: The action 'about' could not be found for StaticPagesController # ./spec/requests/static_pages_spec.rb:24:in `block (3 levels) in <top (required)>'
To fix the problem, we need to add an about action in the StaticPages controller. The StaticPages controller with added about action.(app/controllers/static_pages_controller.rb) looks like this:
class StaticPagesController < ApplicationController def home end def help end def about end end
Now run RSpec again:
$ bundle exec rspec spec/requests/static_pages_spec.rb
We still get complain:
1) Static pages About page should have the content 'About Us' Failure/Error: visit '/static_pages/about' ActionView::MissingTemplate: Missing template static_pages/about, application/about with {:locale=>[:en], :formats=>[:html], :variants=>[], :handlers=>[:erb, :builder, :raw, :ruby, :jbuilder, :coffee]}. Searched in: * "/home/k/rails_projects/simple_app/app/views"
To solve this issue, we add the about view. This involves creating a new file called about.html.erb in the app/views/static_pages directory with the contents shown in the code below:
<h1>About Us</h1> <p> The <a href="http://www.bogotobogo.com/RubyOnRails/RubyOnRails.php">Ruby on Rails Tutorial</a> is a project to make a book and screencasts to teach web development with <a href="http://rubyonrails.org/">Ruby on Rails</a>. This is the sample application for the tutorial. </p>
This time it works:
$ bundle exec rspec spec/requests/static_pages_spec.rb ... Finished in 0.46148 seconds 3 examples, 0 failures Randomized with seed 30262
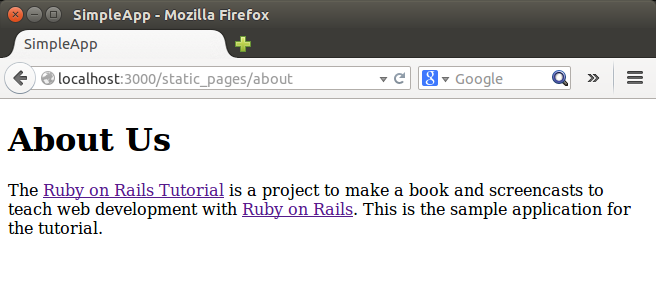
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization