Simple App II (TDD - Home /Help page) 2020
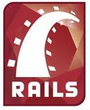
We'll begin by adding some content to the Home page using test-driven development, including a top-level heading (<h1>) with the content Simple App. The first step is to generate an integration test (request spec) for our static pages:
$ rails generate integration_test static_pages invoke rspec create spec/requests/static_pages_spec.rb
This creates the static_pages_spec.rb in the spec/requests directory:
require 'spec_helper' describe "StaticPages" do describe "GET /static_pages" do it "works! (now write some real specs)" do # Run the generator again with the --webrat flag if you want to use webrat methods/matchers get static_pages_index_path response.status.should be(200) end end end
We want to replace it with a code to test the contents of the Home page:
require 'spec_helper' describe "Static pages" do describe "Home page" do it "should have the content 'Simple App'" do visit '/static_pages/home' expect(page).to have_content('Simple App') end end end
RSpec uses the general malleability of Ruby to define a domain-specific language (DSL) built just for testing. The important point is that you do not need to understand RSpec's syntax to be able to use RSpec. It may seem like magic at first, but RSpec and Capybara are designed to read more or less like English.
The contains a describe block with one example, i.e., a block starting with it "..." do:
describe "Home page" do it "should have the content 'Simple App'" do visit '/static_pages/home' expect(page).to have_content('Simple App') end end
The first line
describe "Home page" do
indicates that we are describing the Home page. This description is just a string, and it can be anything we want; RSpec doesn't care, but we and other human readers probably do. Then the spec says that when we visit the Home page at /static_pages/home, the content should contain the words "Simple App". As with the first line, what goes inside the quote marks is irrelevant to RSpec, and is intended to be descriptive to human readers. Then the line:
visit '/static_pages/home'
uses the Capybara function visit to simulate visiting the URL /static_pages/home in a browser.
the line
expect(page).to have_content('Simple App')
uses the page variable (also provided by Capybara) to express the expectation that the resulting page should have the right content.
To get the test to run properly, we have to add a line to the spec/spec_helper.rb file, as shown below:
RSpec.configure do |config| ... config.include Capybara::DSL end
The file is copied to spec/ when we run 'rails generate rspec:install'.
To actually run the test, we'll use the rspec command at the command line (executed with bundle exec to ensure that RSpec runs in the environment specified by our Gemfile.
$ bundle exec rspec spec/requests/static_pages_spec.rb
This yields a failing test:
F Failures: 1) Static pages Home page should have the content 'Simple App' Failure/Error: expect(page).to have_content('Simple App') expected #has_content?("Simple App") to return true, got false # ./spec/requests/static_pages_spec.rb:9:in `block (3 levels) in <top (required)>' Finished in 0.40747 seconds 1 example, 1 failure Failed examples: rspec ./spec/requests/static_pages_spec.rb:7 # Static pages Home page should have the content 'Simple App' Randomized with seed 23348
Current page (app/views/static_pages/home.html.erb) looks like this:
<h1>StaticPages#home</h1> <p>Find me in app/views/static_pages/home.html.erb</p>
We need to replace with the following code with the content Simple App, which should get the test to pass.
<h1>Simple App</h1> <p> This is the home page for the <a href="http://www.bogotobogo.com/RubyOnRails/RubyOnRails_Simple_App_2_TDD.php">Ruby on Rails Tutorial</a> simple application. </p>
Let's run the test again to see the effect:
$ bundle exec rspec spec/requests/static_pages_spec.rb . Finished in 0.42273 seconds 1 example, 0 failures Randomized with seed 39845
We need to add code to test the contents of the Help page. (spec/requests/static_pages_spec.rb):
require 'spec_helper' describe "Static pages" do describe "Home page" do it "should have the content 'Simple App'" do visit '/static_pages/home' expect(page).to have_content('Simple App') end end describe "Help page" do it "should have the content 'Help'" do visit '/static_pages/help' expect(page).to have_content('Help') end end end
Then run the tests:
$ bundle exec rspec spec/requests/static_pages_spec.rb
The test should fail.
The code to get a passing test for the Help page (app/views/static_pages/help.html.erb):
<h1>Help</h1> <p> Get help on the Ruby on Rails Tutorial at the <a href="http://localhost:3000/static_pages/help">Rails Tutorial help page</a>. </p>
Now the tests should now pass:
$ bundle exec rspec spec/requests/static_pages_spec.rb .. Finished in 0.47866 seconds 2 examples, 0 failures Randomized with seed 59258
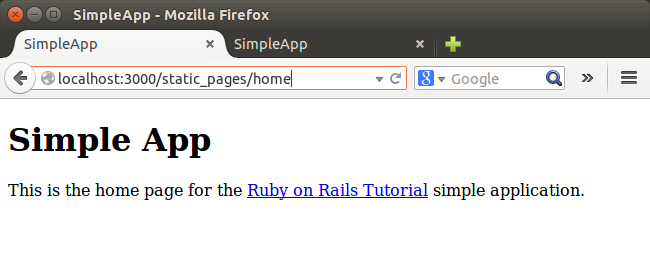
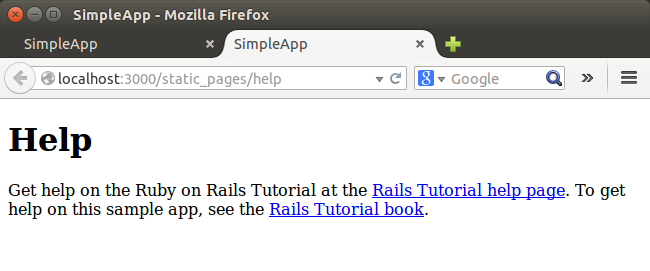
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization