Simple App IV (TDD - Dynamic Pages) 2020
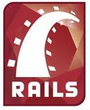
We've made Home and Help page using rails generate command in the previous chapter (Simple_app II - TDD (Home/Help)) and About page (Simple_app III - TDD (About page)). In the chapter, we'll convert static pages into dynamic pages.
We'll start by writing tests for the titles, then add the titles themselves, and finally use a layout file to refactor the resulting pages and eliminate duplication.
users table:
Page | URL | Base title | Variable title |
---|---|---|---|
Home | /static_pages/home | "Ruby on Rails Tutorial Simple App" | "Home" |
Help | /static_pages/help | "Ruby on Rails Tutorial Simple App" | "Help" |
About | /static_pages/about | "Ruby on Rails Tutorial Simple App" | "About" |
By the end of this section, all three of our static pages will have titles of the form "Ruby on Rails Tutorial Simple App | Home", "Ruby on Rails Tutorial Simple App | Help", and "Ruby on Rails Tutorial Simple App | About".
We'll build on the tests in spec/requests/static_pages_spec.rb:
require 'spec_helper' describe "Static pages" do describe "Home page" do it "should have the content 'Simple App'" do visit '/static_pages/home' expect(page).to have_content('Simple App') end end describe "Help page" do it "should have the content 'Help'" do visit '/static_pages/help' expect(page).to have_content('Help') end end describe "About page" do it "should have the content 'About Us'" do visit '/static_pages/about' expect(page).to have_content('About Us') end end end
We'll add title tests following the model in the table above.
spec/requests/static_pages_spec.rb:
it "should have the right title" do visit '/static_pages/home' expect(page).to have_title("Ruby on Rails Tutorial Simple App | Home") end
This uses the have_title method, which checks for an HTML title with the given content. The code checks to see that the content inside the title tag is:
"Ruby on Rails Tutorial Simple App | Home"
The content do not have to be an exact match; any substring works as well, such as this:
expect(page).to have_title("Home")
Adding new tests for each of our three static pages, gives us our new StaticPages test (spec/requests/static_pages_spec.rb):
require 'spec_helper' describe "Static pages" do describe "Home page" do it "should have the content 'Simple App'" do visit '/static_pages/home' expect(page).to have_content('Simple App') end it "should have the title 'Home'" do visit '/static_pages/home' expect(page).to have_title("Ruby on Rails Tutorial Simple App | Home") end end describe "Help page" do it "should have the content 'Help'" do visit '/static_pages/help' expect(page).to have_content('Help') end it "should have the title 'Help'" do visit '/static_pages/help' expect(page).to have_title("Ruby on Rails Tutorial Simple App | Help") end end describe "About page" do it "should have the content 'About Us'" do visit '/static_pages/about' expect(page).to have_content('About Us') end it "should have the title 'About Us'" do visit '/static_pages/about' expect(page).to have_title("Ruby on Rails Tutorial Simple App | About Us") end end end
If we run:
$ bundle exec rspec spec/requests/static_pages_spec.rb
We'll get message for failed tests.
The view for the Home page (app/views/static_pages/home.html.erb):
<!DOCTYPE html> <html> <head> <title>Ruby on Rails Tutorial Simple App | Home</title> </head> <body> <h1>Simple App</h1> <p> This is the home page for the <a href="http://www.bogotobogo.com/RubyOnRails/RubyOnRails.php">Ruby on Rails Tutorial</a> simple application. </p> </body> </html>
Note that we're using <title>Ruby on Rails Tutorial Simple App | Home</title> for a new title.
The view for the Help page (app/views/static_pages/help.html.erb):
<!DOCTYPE html> <html> <head> <title>Ruby on Rails Tutorial Simple App | Help</title> </head> <body> <h1>Help</h1> <p> Get help on the Ruby on Rails Tutorial at the <a href="http://www.bogotobogo.com/RubyOnRails/RubyOnRails.php">Rails Tutorial help page</a>. </p> </body> </html>
The view for the Help page (app/views/static_pages/about.html.erb):
<html> <head> <title>Ruby on Rails Tutorial Simple App | About Us</title> </head> <body> <h1>About Us</h1> <p> The <a href="http://www.bogotobogo.com/RubyOnRails/RubyOnRails.php">Ruby on Rails Tutorial</a> is a project to make a book and screencasts to teach web development with <a href="http://www.bogotobogo.com/RubyOnRails/RubyOnRails.php">Ruby on Rails</a>. This is the simple application for the tutorial. </p> </body> </html>
If we run:
$ bundle exec rspec spec/requests/static_pages_spec.rb
we pass the test.
...... Finished in 0.56164 seconds 6 examples, 0 failures Randomized with seed 52439
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization