Microblog App 4 - Microposts resource 2020
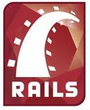
Now that we've completed a quick overview of the Users resource (Microblog 3), in this chapter, we will examine microposts resource.
In this section, we'll generate scaffold code for the microposts resource using rails generate scaffold implementing the data model as shown in the table below:
microposts table:
microposts | |
id | integer |
content | string |
user_id | integer |
$ rails generate scaffold Micropost content:string user_id:integer Running via Spring preloader in process 16008 invoke active_record create db/migrate/20160127235735_create_microposts.rb create app/models/micropost.rb invoke test_unit create test/models/micropost_test.rb create test/fixtures/microposts.yml invoke resource_route route resources :microposts invoke scaffold_controller create app/controllers/microposts_controller.rb invoke erb create app/views/microposts create app/views/microposts/index.html.erb create app/views/microposts/edit.html.erb create app/views/microposts/show.html.erb create app/views/microposts/new.html.erb create app/views/microposts/_form.html.erb invoke test_unit create test/controllers/microposts_controller_test.rb invoke helper create app/helpers/microposts_helper.rb invoke test_unit invoke jbuilder create app/views/microposts/index.json.jbuilder create app/views/microposts/show.json.jbuilder invoke assets invoke coffee create app/assets/javascripts/microposts.coffee invoke scss create app/assets/stylesheets/microposts.scss invoke scss identical app/assets/stylesheets/scaffolds.scss
We need to run the migration to update our database with the new data model (microposts):
$ bundle exec rake db:migrate == 20160127235735 CreateMicroposts: migrating ================================= -- create_table(:microposts) -> 0.0191s == 20160127235735 CreateMicroposts: migrated (0.0201s) ========================
The scaffold generator has updated the Rails routes file with a rule for Microposts resource:
Rails.application.routes.draw do resources :microposts resources :users end
The resources :microposts routing rule maps micropost URLs to actions in the Microposts controller as shown in the following table:
HTTP request | URL | Action | Purpose |
---|---|---|---|
GET | /microposts | index | page to list all microposts |
GET | /microposts/1 | show | page to show micropost with id 1 |
GET | /microposts/new | new | page to make a new micropost |
POST | /microposts | create | create a new micropost |
GET | /microposts/1/edit | edit | page to edit micropost with id 1 |
PATCH | /microposts/1 | update | update micropost with id 1 |
DELETE | /microposts/1 | destroy | delete micropost with id 1 |
The Microposts controller (app/controllers/microposts_controller.rb) looks like this:
class MicropostsController < ApplicationController before_action :set_micropost, only: [:show, :edit, :update, :destroy] def index ... end def show ... end def new ... end def edit ... end def create ... end def update ... end def destroy ... end end
We enter information at the new microposts page, /microposts/new:
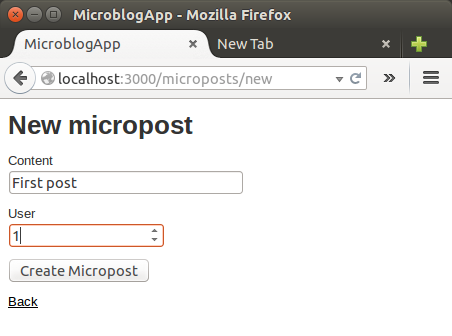
After creating two microposts, we can see the list:
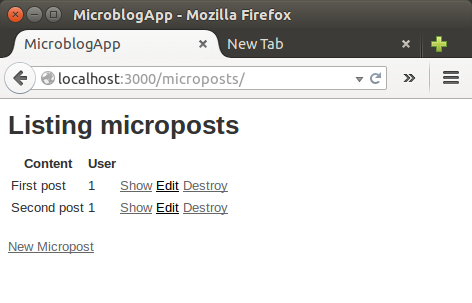
To implement length constraint in Rails, we ca use a length validation (app/models/micropost.rb):
class Micropost < ActiveRecord::Base validates :content, length: { maximum: 140 } end
We can see the validation is working from the picture below (more than 140 characters):
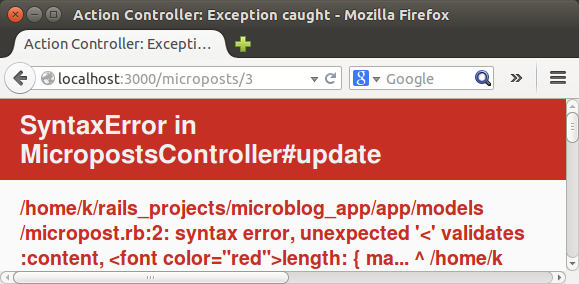
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization