Devise - Adding custom fields to Devise User model and Customization 2020
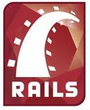
In this chapter, we'll extend the previous code from Authentication and sending confirmation email using Devise.
We're going to custom fields to Devise User model and also add views for Devise routes.
Source available : Github : Rails4-Devise-Authentication-Confirmation.
We want to add a field to Devise User model: name. Let's create a new migration file:
$ rails generate migration add_name_to_users name:string invoke active_record create db/migrate/20160130022556_add_name_to_users.rb
As we can see from the output, the rails generate migration command created a migration file under db/migrate with a name called 20160130022556_add_name_to_users.rb
Let's check the newly created migration file:
class AddNameToUsers < ActiveRecord::Migration def change add_column :users, :name, :string end end
We can now migrate our database:
$ rake db:migrate == 20160130022556 AddNameToUsers: migrating =================================== -- add_column(:users, :name, :string) -> 0.0069s == 20160130022556 AddNameToUsers: migrated (0.0072s) ==========================
We may want to add a new field for name to the Sign-up form new.html.erb under views/devise/registrations/ folder:
<div class="field"> <%= f.label :name %><br /> <%= f.text_field :first_name, autofocus: true %> </div>
Let's add name field to edit.html.erb file under views/registrations folder:
<h2>Edit <%= resource_name.to_s.humanize %></h2> <%= form_for(resource, as: resource_name, url: registration_path(resource_name), html: { method: :put }) do |f| %> <%= devise_error_messages! %> <div class="field"> <%= f.label :name %><br /> <%= f.text_field :name, autofocus: true %></div> <div class="field"> <%= f.label :email %><br /> <%= f.email_field :email, autofocus: true %> </div>
As we can see, now we have a new field for name in our sign-up form:
After sign-up:
Once confirmed we can login:
After logged in, we want to edit the profile:
Click on "Edit profile":
Click "Update", and go back to the page, and we can find the name field was NOT saved at all:
To make the update work, we need to override the devise controller.
The reason that the update's not working is is because the way how Rails 4 deals with mass assignment.
For security reasons, we have to explicitly permit parameters inside each controller. So, we just want to add permissions to application controller as following:
class ApplicationController < ActionController::Base # Prevent CSRF attacks by raising an exception. # For APIs, you may want to use :null_session instead. protect_from_forgery with: :exception before_filter :configure_permitted_parameters, if: :devise_controller? protected def configure_permitted_parameters devise_parameter_sanitizer.for(:sign_up) { |u| u.permit(:name, :email, :password) } devise_parameter_sanitizer.for(:account_update) { |u| u.permit(:name, :email, :password, :current_password) } end end
After the fix, if we go back to "Edit profile" page, this time, we can see the name was saved!
For more customization, please visit next tutorial: Devise Customization 2. views/users
Ruby on Rails
- Ruby On Rails Home
- Ruby - Input/Output, Objects, Load
- Ruby - Condition (if), Operators (comparison/logical) & case statement
- Ruby - loop, while, until, for, each, (..)
- Ruby - Functions
- Ruby - Exceptions (raise/rescue)
- Ruby - Strings (single quote vs double quote, multiline string - EOM, concatenation, substring, include, index, strip, justification, chop, chomp, split)
- Ruby - Class and Instance Variables
- Ruby - Class and Instance Variables II
- Ruby - Modules
- Ruby - Iterator : each
- Ruby - Symbols (:)
- Ruby - Hashes (aka associative arrays, maps, or dictionaries)
- Ruby - Arrays
- Ruby - Enumerables
- Ruby - Filess
- Ruby - code blocks and yield
- Rails - Embedded Ruby (ERb) and Rails html
- Rails - Partial template
- Rails - HTML Helpers (link_to, imag_tag, and form_for)
- Layouts and Rendering I - yield, content_for, content_for?
- Layouts and Rendering II - asset tag helpers, stylesheet_link_tag, javascript_include_tag
- Rails Project
- Rails - Hello World
- Rails - MVC and ActionController
- Rails - Parameters (hash, array, JSON, routing, and strong parameter)
- Filters and controller actions - before_action, skip_before_action
- The simplest app - Rails default page on a Shared Host
- Redmine Install on a Shared Host
- Git and BitBucket
- Deploying Rails 4 to Heroku
- Scaffold: A quickest way of building a blog with posts and comments
- Databases and migration
- Active Record
- Microblog 1
- Microblog 2
- Microblog 3 (Users resource)
- Microblog 4 (Microposts resource I)
- Microblog 5 (Microposts resource II)
- Simple_app I - rails html pages
- Simple_app II - TDD (Home/Help page)
- Simple_app III - TDD (About page)
- Simple_app IV - TDD (Dynamic Pages)
- Simple_app V - TDD (Dynamic Pages - Embedded Ruby)
- Simple_app VI - TDD (Dynamic Pages - Embedded Ruby, Layouts)
- App : Facebook and Twitter Authentication using Omniauth oauth2
- Authentication and sending confirmation email using Devise
- Adding custom fields to Devise User model and Customization
- Devise Customization 2. views/users
- Rails Heroku Deploy - Authentication and sending confirmation email using Devise
- Deploying a Rails 4 app on CentOS 7 production server with Apache and Passenger I
- Deploying a Rails 4 app on CentOS 7 production server with Apache and Passenger II
- OOPS! Deploying a Rails 4 app on CentOS 7 production server with Apache and Passenger (Trouble shooting)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization