GoLang Tutorial - AWS SDK for Go (S3 listing)
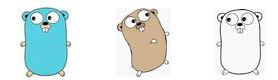
aws-sdk-go is the official AWS SDK for the Go programming language.
The SDK is composed of two main components, SDK core, and service clients.
The SDK core packages are all available under the aws package at the root of the SDK. Each client for a supported AWS service is available within its own package under the service folder at the root of the SDK.
- aws - SDK core: it provides common shared types such as Config, Logger, and utilities to make working with API parameters easier.
- service - Clients: is is for AWS services. All services supported by the SDK are available under this folder.
Use go get
to retrieve the SDK to add it to our GOPATH workspace, or project's Go module dependencies:
$ go get github.com/aws/aws-sdk-go go: finding github.com/aws/aws-sdk-go v1.19.23 go: finding github.com/jmespath/go-jmespath v0.0.0-20180206201540-c2b33e8439af go: downloading github.com/aws/aws-sdk-go v1.19.23 go: extracting github.com/aws/aws-sdk-go v1.19.23
This will add the following line to go.mod:
require github.com/aws/aws-sdk-go v1.19.23 // indirect
For more on module, please visit Modules 1 (Creating a new module) or Modules 2 (Adding Dependencies).
To update the SDK use go get -u
to retrieve the latest version of the SDK:
$ go get -u github.com/aws/aws-sdk-go go: finding github.com/jmespath/go-jmespath latest
Because we're using Go modules, our go get
defaulted to the latest tagged release version of the SDK. To get a specific release version of the SDK use @<tag> in our go get
command:
$ go get github.com/aws/aws-sdk-go@v1.15.77
To get the latest SDK repository change use @latest.
$ go get github.com/aws/aws-sdk-go@latest
The following sample from wsdocs/aws-doc-sdk-examples lists the buckets in our account. There are no command line arguments.
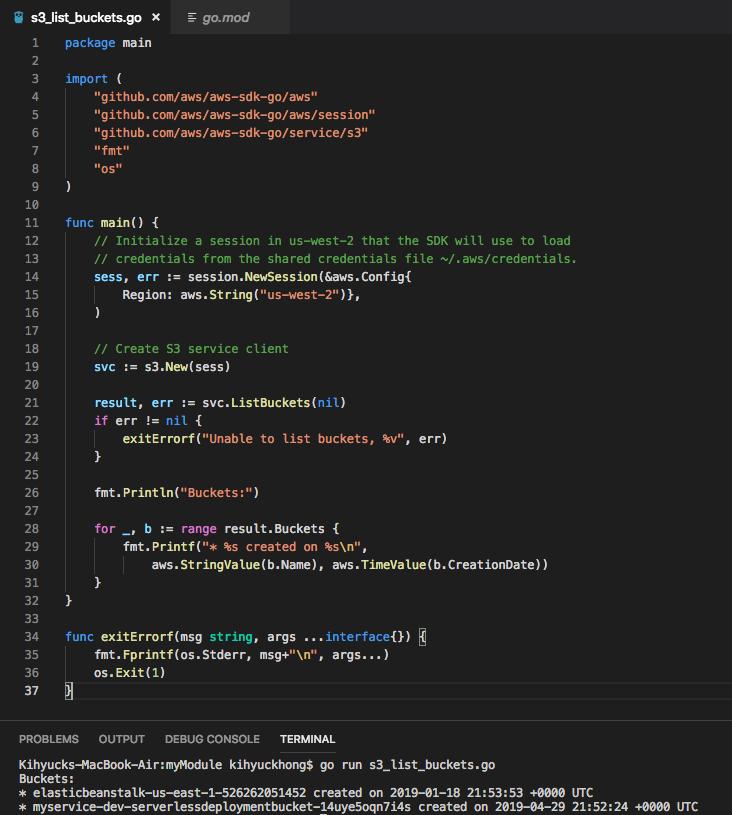
The code: s3_list_buckets.go
First, it adds the following statements to import the Go and AWS SDK for Go packages used in the example:
import ( "github.com/aws/aws-sdk-go/aws" "github.com/aws/aws-sdk-go/aws/session" "github.com/aws/aws-sdk-go/service/s3" "fmt" "os" )
Then, creates a function we use to display errors and exit:
func exitErrorf(msg string, args ...interface{}) { fmt.Fprintf(os.Stderr, msg+"\n", args...) os.Exit(1) }
Note that a parameter type prefixed with three dots (...) is used. It is called a variadic parameter. That means we can pass any number or arguments into that parameter (check Passing arguments to ... parameters).
Initialize the session that the SDK uses to load credentials from the shared credentials file ~/.aws/credentials, and create a new Amazon S3 service client:
sess, err := session.NewSession(&aws.Config{ Region: aws.String("us-west-2")}, ) // Create S3 service client svc := s3.New(sess)
Next, calls ListBuckets. Passing nil means no filters are applied to the returned list. If an error occurs, call exitErrorf. If no error occurs, loop through the buckets, printing the name and creation date of each bucket.
result, err := svc.ListBuckets(nil) if err != nil { exitErrorf("Unable to list buckets, %v", err) } fmt.Println("Buckets:") for _, b := range result.Buckets { fmt.Printf("* %s created on %s\n", aws.StringValue(b.Name), aws.TimeValue(b.CreationDate)) }
Go Tutorial
- GoLang Tutorial - HelloWorld
- Calling code in an external package & go.mod / go.sum files
- Workspaces
- Workspaces II
- Visual Studio Code
- Data Types and Variables
- byte and rune
- Packages
- Functions
- Arrays and Slices
- A function taking and returning a slice
- Conditionals
- Loops
- Maps
- Range
- Pointers
- Closures and Anonymous Functions
- Structs and receiver methods
- Value or Pointer Receivers
- Interfaces
- Web Application Part 0 (Introduction)
- Web Application Part 1 (Basic)
- Web Application Part 2 (Using net/http)
- Web Application Part 3 (Adding "edit" capability)
- Web Application Part 4 (Handling non-existent pages and saving pages)
- Web Application Part 5 (Error handling and template caching)
- Web Application Part 6 (Validating the title with a regular expression)
- Web Application Part 7 (Function Literals and Closures)
- Building Docker image and deploying Go application to a Kubernetes cluster (minikube)
- Serverless Framework (Serverless Application Model-SAM)
- Serverless Web API with AWS Lambda
- Arrays vs Slices with an array left rotation sample
- Variadic Functions
- Goroutines
- Channels ("<-")
- Channels ("<-") with Select
- Channels ("<-") with worker pools
- Defer
- GoLang Panic and Recover
- String Formatting
- JSON
- SQLite
- Modules 0: Using External Go Modules from GitHub
- Modules 1 (Creating a new module)
- Modules 2 (Adding Dependencies)
- AWS SDK for Go (S3 listing)
- Linked List
- Binary Search Tree (BST) Part 1 (Tree/Node structs with insert and print functions)
- Go Application Authentication I (BasicAuth, Bearer-Token-Based Authentication)
- Go Application Authentication II (JWT Authentication)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization