GoLang Tutorial - Workspaces II
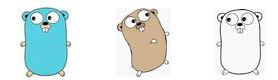
Continued from Workspaces.
In this section, we'll download a copy of the Git repo containing the golang.org/x/example/hello module, add it to the workspace, and then add a new function to it that we will use from the hello program.
Also note that there is no real repo for golang.org/x/example/hello because it is an example module. Example modules are typically located in the go/example directory of the Go source tree. The x in golang.org/x/example/hello is a prefix that indicates that the module is experimental. Experimental modules are not considered to be part of the Go standard library, and they may not be as stable or well-supported as core modules.
From the workspace directory, run the git command to clone the repository:
$ git clone https://go.googlesource.com/example
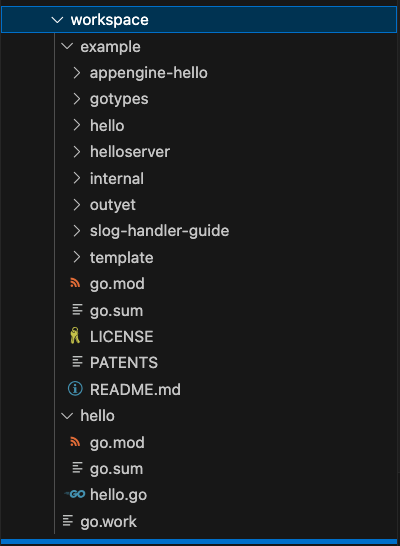
As show in the picture, the Git repo was just checked out into ./example. The source code for the golang.org/x/example/hello module is in ./example/hello. Add it to the workspace:
$ go work use ./example/hello
The go work use
command adds a new module to the go.work file. It will now look like this:
go 1.21.4 use ( ./example/hello ./hello )
Benefits of using a go.work file:
There are several benefits to using a go.work file:
- It allows us to develop multiple Go modules in the same workspace.
- It allows us to use private modules that are not published to the registry.
- It can help to improve the performance of Go builds by reducing the number of network requests required.
When to use a go.work file:
We should use a go.work file if we are developing multiple Go modules in the same workspace, or if we need to use private modules that are not published to the registry.
When not to use a go.work file:
If we are only developing a single Go module, and we do not need to use private modules, then we do not need to use a go.work file.
We'll add a new function to reverse a number to the golang.org/x/example/hello/reverse package.
Create a new file named int.go in the workspace/example/hello/reverse directory containing the following contents:
package reverse import "strconv" // Int returns the decimal reversal of the integer i. func Int(i int) int { i, _ = strconv.Atoi(String(strconv.Itoa(i))) return i }
The package imports the strconv package. This package provides functions for converting strings to other types and vice versa, including functions for converting integers to strings (Itoa) and strings to integers (Atoi).
The use of the underscore (_) is an indicator that the error returned by strconv.Atoi is being ignored. This is common when the error is not expected to occur or when it's intentionally being ignored.
Also, we want to modify the workspace/hello/hello.go program to use the function.
package main import ( "fmt" "golang.org/x/example/hello/reverse" ) func main() { fmt.Println(reverse.String("Hello"), reverse.Int(12345)) }
From the workspace directory, run:
$ go run ./hello olleH 54321
The Go command finds the example.com/hello module specified in the command line in the hello directory specified by the go.work file, and similarly resolves the golang.org/x/example/hello/reverse import using the go.work file.
Note that the x in golang.org/x/example/hello is a prefix that indicates that the module is experimental. Experimental modules are not considered to be part of the Go standard library, and they may not be as stable or well-supported as core modules.
Go Tutorial
- GoLang Tutorial - HelloWorld
- Calling code in an external package & go.mod / go.sum files
- Workspaces
- Workspaces II
- Visual Studio Code
- Data Types and Variables
- byte and rune
- Packages
- Functions
- Arrays and Slices
- A function taking and returning a slice
- Conditionals
- Loops
- Maps
- Range
- Pointers
- Closures and Anonymous Functions
- Structs and receiver methods
- Value or Pointer Receivers
- Interfaces
- Web Application Part 0 (Introduction)
- Web Application Part 1 (Basic)
- Web Application Part 2 (Using net/http)
- Web Application Part 3 (Adding "edit" capability)
- Web Application Part 4 (Handling non-existent pages and saving pages)
- Web Application Part 5 (Error handling and template caching)
- Web Application Part 6 (Validating the title with a regular expression)
- Web Application Part 7 (Function Literals and Closures)
- Building Docker image and deploying Go application to a Kubernetes cluster (minikube)
- Serverless Framework (Serverless Application Model-SAM)
- Serverless Web API with AWS Lambda
- Arrays vs Slices with an array left rotation sample
- Variadic Functions
- Goroutines
- Channels ("<-")
- Channels ("<-") with Select
- Channels ("<-") with worker pools
- Defer
- GoLang Panic and Recover
- String Formatting
- JSON
- SQLite
- Modules 0: Using External Go Modules from GitHub
- Modules 1 (Creating a new module)
- Modules 2 (Adding Dependencies)
- AWS SDK for Go (S3 listing)
- Linked List
- Binary Search Tree (BST) Part 1 (Tree/Node structs with insert and print functions)
- Go Application Authentication I (BasicAuth, Bearer-Token-Based Authentication)
- Go Application Authentication II (JWT Authentication)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization