GoLang Tutorial - Modules 1 (Creating a new module)
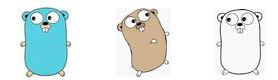
This post is an introduction to the basic operations needed to get started using modules.
A module is a collection of Go packages stored in a file tree with a go.mod file at its root. The go.mod file defines the module's module path, which is also the import path used for the root directory, and its dependency requirements, which are the other modules needed for a successful build. Each dependency requirement is written as a module path and a specific semantic version.
Starting from Go 1.13, module mode will be the default for all development.
Go code is grouped into packages, and packages are grouped into modules. Our module specifies dependencies needed to run our code, including the Go version and the set of other modules it requires.
Sourcing one module per repository:
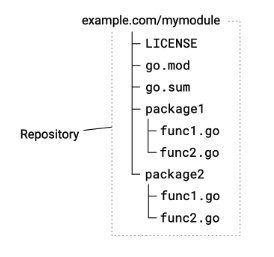
Sourcing multiple modules per repository:
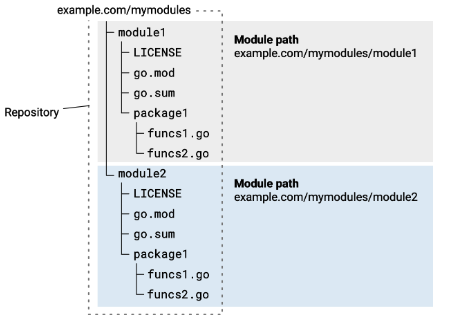
Here is the file structure we're going to produce:
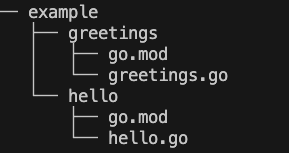
Let's create a new module.
First, we want to create a new, empty directory, greetings, cd into that directory:
$ mkdir greetings $ cd greetings
Run the go mod init
command, giving it the module path -- here, use example/greetings:
$ go mod init example/greetings go: creating new go.mod: module example/greetings
The go mod init
command creates a go.mod file to track code's dependencies.
So far, the file includes only the name of module and the supported Go version.
But as we add dependencies, the go.mod file will list the versions our code depends on.
This keeps builds reproducible and gives us direct control over which module versions to use.
greetings.go:
package greetings import "fmt" // Hello returns a greeting for the named person. func Hello(name string) string { // Return a greeting that embeds the name in a message. message := fmt.Sprintf("Hi, %v. Welcome!", name) return message }
In Go, a function whose name starts with a capital letter can be called by a function not in the same package. This is known in Go as an exported name.
In Go, the := operator is a shortcut for declaring and initializing a variable in one line, but we might have written the line as the following:
var message string message = fmt.Sprintf("Hi, %v. Welcome!", name)
Go Tutorial
- GoLang Tutorial - HelloWorld
- Calling code in an external package & go.mod / go.sum files
- Workspaces
- Workspaces II
- Visual Studio Code
- Data Types and Variables
- byte and rune
- Packages
- Functions
- Arrays and Slices
- A function taking and returning a slice
- Conditionals
- Loops
- Maps
- Range
- Pointers
- Closures and Anonymous Functions
- Structs and receiver methods
- Value or Pointer Receivers
- Interfaces
- Web Application Part 0 (Introduction)
- Web Application Part 1 (Basic)
- Web Application Part 2 (Using net/http)
- Web Application Part 3 (Adding "edit" capability)
- Web Application Part 4 (Handling non-existent pages and saving pages)
- Web Application Part 5 (Error handling and template caching)
- Web Application Part 6 (Validating the title with a regular expression)
- Web Application Part 7 (Function Literals and Closures)
- Building Docker image and deploying Go application to a Kubernetes cluster (minikube)
- Serverless Framework (Serverless Application Model-SAM)
- Serverless Web API with AWS Lambda
- Arrays vs Slices with an array left rotation sample
- Variadic Functions
- Goroutines
- Channels ("<-")
- Channels ("<-") with Select
- Channels ("<-") with worker pools
- Defer
- GoLang Panic and Recover
- String Formatting
- JSON
- SQLite
- Modules 0: Using External Go Modules from GitHub
- Modules 1 (Creating a new module)
- Modules 2 (Adding Dependencies)
- AWS SDK for Go (S3 listing)
- Linked List
- Binary Search Tree (BST) Part 1 (Tree/Node structs with insert and print functions)
- Go Application Authentication I (BasicAuth, Bearer-Token-Based Authentication)
- Go Application Authentication II (JWT Authentication)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization