GoLang Tutorial - Defer
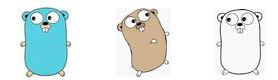
Defer is used to ensure that a function call is performed later in a program's execution, usually for purposes of cleanup. In other words, defer statement defers the execution of a function until the surrounding function returns.
Deferred function call is guaranteed to run in every case including panic. We don't have this guarantee when we just place a function call at end of surrounding function.
Moreover a deffered function call can handle panic by calling recover builtin function. This cannot be done by function at the end of surrounding function.
Each deffered call is put on stack and executed in reverse order (LIFO) at the time surrounding function ends. The reversed order helps correctly deallocated resources.
Here is the code that clearly shows defer really defers the execution:
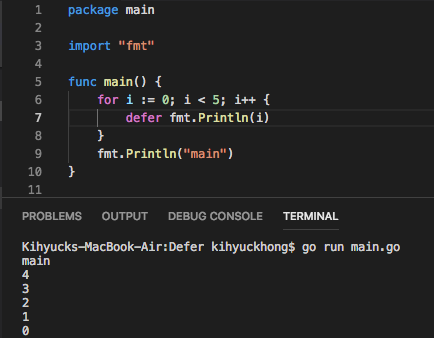
Here is another sample code using defer which makes it sure the the file will be closed no matter what happens:
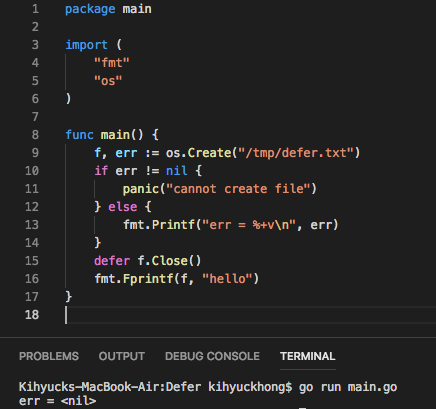
We created a file and then close when we're done. Immediately after getting a file object with Create(), we deferred the closing of that file with Close(). This was executed at the end of the enclosing function (main), after Fprintf() has finished.
$ cat /tmp/defer.txt hello
If we want to create a file under the directory(tmp2) that's not exists, the code we get to panic. Still, the deferred function (f.Close()) will be executed:
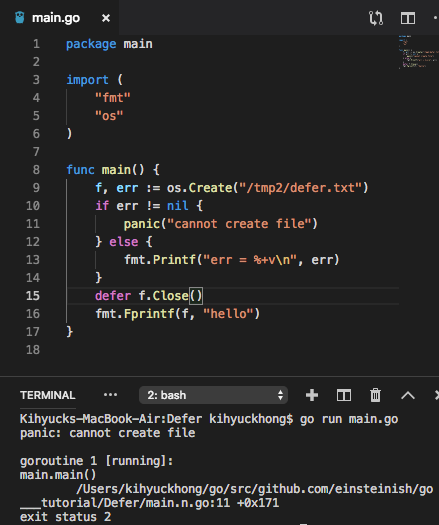
Go Tutorial
- GoLang Tutorial - HelloWorld
- Calling code in an external package & go.mod / go.sum files
- Workspaces
- Workspaces II
- Visual Studio Code
- Data Types and Variables
- byte and rune
- Packages
- Functions
- Arrays and Slices
- A function taking and returning a slice
- Conditionals
- Loops
- Maps
- Range
- Pointers
- Closures and Anonymous Functions
- Structs and receiver methods
- Value or Pointer Receivers
- Interfaces
- Web Application Part 0 (Introduction)
- Web Application Part 1 (Basic)
- Web Application Part 2 (Using net/http)
- Web Application Part 3 (Adding "edit" capability)
- Web Application Part 4 (Handling non-existent pages and saving pages)
- Web Application Part 5 (Error handling and template caching)
- Web Application Part 6 (Validating the title with a regular expression)
- Web Application Part 7 (Function Literals and Closures)
- Building Docker image and deploying Go application to a Kubernetes cluster (minikube)
- Serverless Framework (Serverless Application Model-SAM)
- Serverless Web API with AWS Lambda
- Arrays vs Slices with an array left rotation sample
- Variadic Functions
- Goroutines
- Channels ("<-")
- Channels ("<-") with Select
- Channels ("<-") with worker pools
- Defer
- GoLang Panic and Recover
- String Formatting
- JSON
- SQLite
- Modules 0: Using External Go Modules from GitHub
- Modules 1 (Creating a new module)
- Modules 2 (Adding Dependencies)
- AWS SDK for Go (S3 listing)
- Linked List
- Binary Search Tree (BST) Part 1 (Tree/Node structs with insert and print functions)
- Go Application Authentication I (BasicAuth, Bearer-Token-Based Authentication)
- Go Application Authentication II (JWT Authentication)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization