GoLang Tutorial - Workspaces I
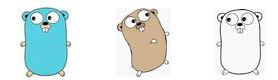
In this tutorial, we'll create two modules in a shared multi-module workspace, make changes across those modules, and see the results of those changes in a build.
$ mkdir workspace $ cd workspace
Our example will create a new module hello that will depend on the golang.org/x/example module.
$ mkdir hello $ cd hello $ go mod init example.com/hello go: creating new go.mod: module example.com/hello
Now, we want to add a dependency on the golang.org/x/example/hello/reverse package using go get
command.
It will download a Go package from the specified repository.
$ go get golang.org/x/example/hello/reverse go: downloading golang.org/x/example v0.0.0-20231013143937-1d6d2400d402 go: downloading golang.org/x/example/hello v0.0.0-20231013143937-1d6d2400d402 go: added golang.org/x/example/hello v0.0.0-20231013143937-1d6d2400d402
The go get
command is fetching the specified Go package and its dependencies,
and the output indicates the versions that have been downloaded and added to our project.
After running this command, we can use the package in our Go code, and it will be included in our Go module.
Let's create hello/hello.go with the following contents:
package main import ( "fmt" "golang.org/x/example/hello/reverse" ) func main() { fmt.Println(reverse.String("Hello")) }
Run the code:
$ go run . olleH
In this section, we'll create a go.work file to specify a workspace with the module.
In the workspace directory, run go work init
command to tell go to create a go.work file
for a workspace containing the modules in the ./hello directory.
$ go work init ./hello
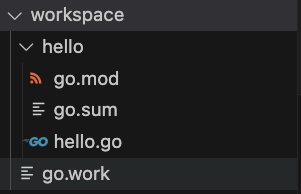
The go command produces a go.work file that looks like this:
$ cat go.work go 1.21.4 use ./hello
The go.work file has similar syntax to go.mod.
- The
go
directive tells Go which version of Go the file should be interpreted with. It's similar to the go directive in the go.mod file. - The
use
directive tells Go that the module in the hello directory should be main modules when doing a build. - So in any subdirectory of workspace the module (in this case, the one in the hello directory) will be active and treated as the main module.
Last time, we run the program in workspace/hello directory. But we can run it in workspace directory:
$ go run ./hello olleH
The Go command includes all the modules in the workspace as main modules. This allows us to refer to a package in the module, even outside the module.
Running the go run
command outside the module or the workspace would result in an error
because the go command wouldn't know which modules to use.
Go Tutorial
- GoLang Tutorial - HelloWorld
- Calling code in an external package & go.mod / go.sum files
- Workspaces
- Workspaces II
- Visual Studio Code
- Data Types and Variables
- byte and rune
- Packages
- Functions
- Arrays and Slices
- A function taking and returning a slice
- Conditionals
- Loops
- Maps
- Range
- Pointers
- Closures and Anonymous Functions
- Structs and receiver methods
- Value or Pointer Receivers
- Interfaces
- Web Application Part 0 (Introduction)
- Web Application Part 1 (Basic)
- Web Application Part 2 (Using net/http)
- Web Application Part 3 (Adding "edit" capability)
- Web Application Part 4 (Handling non-existent pages and saving pages)
- Web Application Part 5 (Error handling and template caching)
- Web Application Part 6 (Validating the title with a regular expression)
- Web Application Part 7 (Function Literals and Closures)
- Building Docker image and deploying Go application to a Kubernetes cluster (minikube)
- Serverless Framework (Serverless Application Model-SAM)
- Serverless Web API with AWS Lambda
- Arrays vs Slices with an array left rotation sample
- Variadic Functions
- Goroutines
- Channels ("<-")
- Channels ("<-") with Select
- Channels ("<-") with worker pools
- Defer
- GoLang Panic and Recover
- String Formatting
- JSON
- SQLite
- Modules 0: Using External Go Modules from GitHub
- Modules 1 (Creating a new module)
- Modules 2 (Adding Dependencies)
- AWS SDK for Go (S3 listing)
- Linked List
- Binary Search Tree (BST) Part 1 (Tree/Node structs with insert and print functions)
- Go Application Authentication I (BasicAuth, Bearer-Token-Based Authentication)
- Go Application Authentication II (JWT Authentication)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization