GoLang Tutorial - Modules 2 (Adding Dependencies)
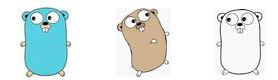
In the previous section (Modules 1 (Creating a new module) ), we created a greetings module. In this section, we'll write code to make calls to the Hello function in the module. We'll write code we can execute as an application, and which calls code in the greetings module.
Create a hello directory for our Go module source code. The hello directory is where we'll write our caller:
$ mkdir hello $ cd hello $ go mod init example/hello go: creating new go.mod: module example/hello go: to add module requirements and sums: go mod tidy
package main import ( "fmt" "example/greetings" ) func main() { // Get a greeting message and print it. message := greetings.Hello("Bogotobogo") fmt.Println(message) }
The code does the following:
- Declare a main package:
In Go, code executed as an application must be in a main package. - Import two packages:
example/greetings and the fmt package. This gives our code access to functions in those packages. Importing example/greetings (the package contained in the module we created earlier) gives us access to the Hello function. We also import fmt, with functions for handling input and output text (such as printing text to the console). - Get a greeting by calling the greetings package's Hello function.
Because we haven't published the module yet, we need to adapt the example/hello module so that
it can find the example/greetings code on our local file system.
To do that, use the go mod edit
command to edit the example/hello module to redirect Go tools
from its module path (where the module isn't) to the local directory (where it is).
$ go mod edit -replace example/greetings=../greetings
The command specifies that example/greetings should be replaced with ../greetings for the purpose of locating the dependency. After we run the command, the go.mod file in the hello directory should include a replace directive:
module example/hello go 1.21.4 replace example/greetings => ../greetings
Then, from the command prompt in the hello directory,
run the go mod tidy
command to synchronize the example/hello module's dependencies,
adding those required by the code, but not yet tracked in the module:
$ go mod tidy go: found example/greetings in example/greetings v0.0.0-00010101000000-000000000000
Now, the example.com/hello module's go.mod file should look like this:
module example/hello go 1.21.4 replace example/greetings => ../greetings require example/greetings v0.0.0-00010101000000-000000000000
Let's run the code:
$ go run . Hi, Bogotobogo. Welcome!
Here is our file structures so far:
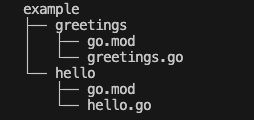
- modules:
Modules are how Go manages dependencies.
Refs: Go Modules Reference
A module is a collection of packages and it is identified by a module path, which is declared in a go.mod file, together with information about the module's dependencies.
A module path is the canonical name for a module, declared with the module directive in the module's go.mod file. A module's path is the prefix for package paths within the module.
- go mod init:
usage:go mod init [module-path]
example:go mod init example.com
Thego mod init
command initializes and writes a new go.mod file in the current directory, in effect creating a new module rooted at the current directory. The go.mod file must not already exist.init
accepts one optional argument, the module path for the new module. - go mod tidy:
usage:go mod tidy [-e] [-v] [-go=version] [-compat=version]
go mod tidy
ensures that the go.mod file matches the source code in the module. It adds any missing module requirements necessary to build the current module's packages and dependencies, and it removes requirements on modules that don't provide any relevant packages. It also adds any missing entries toand removes unnecessary entries.
We can change the module path to github.com from example though it's a good practice to use a valid domain or path that uniquely identifies our module.
The final files look like this:

example/greetings/greetings.go:
package greetings import "fmt" func Hello(name string) string { message := fmt.Sprintf("Hi, %v. Welcome!", name) return message }
$ go mod init github.com/greetings go: creating new go.mod: module github.com/greetings go: to add module requirements and sums: go mod tidy
example/greetings/go.mod:
module github.com/greetings go 1.21.4
example/hello/hello.go:
package main import ( "fmt" "github.com/greetings" ) func main() { message := greetings.Hello("Bogotobogo") fmt.Println(message) }
$ cd hello $ go mod init github.com/hello go: creating new go.mod: module github.com/hello go: to add module requirements and sums: go mod tidy
example/hello/go.mod:
module github.com/hello go 1.21.4
$ go mod edit -replace github.com/greetings=../greetings
example/hello/go.mod
module github.com/hello go 1.21.4 replace github.com/greetings => ../greetings
$ go mod tidy go: found github.com/greetings in github.com/greetings v0.0.0-00010101000000-000000000000
example/hello/go.mod
module github.com/hello go 1.21.4 replace github.com/greetings => ../greetings require github.com/greetings v0.0.0-00010101000000-000000000000
$ go run hello.go Hi, Bogotobogo. Welcome!
Go Tutorial
- GoLang Tutorial - HelloWorld
- Calling code in an external package & go.mod / go.sum files
- Workspaces
- Workspaces II
- Visual Studio Code
- Data Types and Variables
- byte and rune
- Packages
- Functions
- Arrays and Slices
- A function taking and returning a slice
- Conditionals
- Loops
- Maps
- Range
- Pointers
- Closures and Anonymous Functions
- Structs and receiver methods
- Value or Pointer Receivers
- Interfaces
- Web Application Part 0 (Introduction)
- Web Application Part 1 (Basic)
- Web Application Part 2 (Using net/http)
- Web Application Part 3 (Adding "edit" capability)
- Web Application Part 4 (Handling non-existent pages and saving pages)
- Web Application Part 5 (Error handling and template caching)
- Web Application Part 6 (Validating the title with a regular expression)
- Web Application Part 7 (Function Literals and Closures)
- Building Docker image and deploying Go application to a Kubernetes cluster (minikube)
- Serverless Framework (Serverless Application Model-SAM)
- Serverless Web API with AWS Lambda
- Arrays vs Slices with an array left rotation sample
- Variadic Functions
- Goroutines
- Channels ("<-")
- Channels ("<-") with Select
- Channels ("<-") with worker pools
- Defer
- GoLang Panic and Recover
- String Formatting
- JSON
- SQLite
- Modules 0: Using External Go Modules from GitHub
- Modules 1 (Creating a new module)
- Modules 2 (Adding Dependencies)
- AWS SDK for Go (S3 listing)
- Linked List
- Binary Search Tree (BST) Part 1 (Tree/Node structs with insert and print functions)
- Go Application Authentication I (BasicAuth, Bearer-Token-Based Authentication)
- Go Application Authentication II (JWT Authentication)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization