GoLang Tutorial - Go Application Authentication I (BasicAuth & Bearer-Token-Based Authentication)
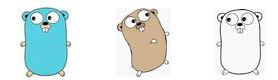
The following code is a simple Go (Golang) program that uses the Gin web framework to create a basic HTTP server.
It defines a single route ("/resource") that responds to GET requests with a JSON payload, and starts the server on port 8080.
main.go:
package main import ( "net/http" "github.com/gin-gonic/gin" ) func main() { r := gin.Default() r.GET("/resource", func(c *gin.Context) { c.JSON(http.StatusOK, gin.H{ "data": "resource data", }) }) r.Run() // Listening and serving HTTP on :8080 }
- package main: This line indicates that this Go file belongs to the main package. In Go, the main package is special, and it is the entry point for the executable programs.
- import:
import ( "net/http" "github.com/gin-gonic/gin" )
Here, the code imports two packages: net/http for handling HTTP requests and responses, and github.com/gin-gonic/gin for using the Gin web framework. - Gin Router Initialization:
r := gin.Default()
This line creates a new Gin router with default middleware. The gin.Default() function sets up some default middleware handlers, including logging and recovery. - HTTP Route Definition:
r.GET("/resource", func(c *gin.Context) { c.JSON(http.StatusOK, gin.H{ "data": "resource data", }) })
- Server Start:
r.Run() // Listening and serving HTTP on :8080
This line starts the HTTP server and listens on the default port ":8080". The Run method is a convenient way to start the server. The program will block here until the server is stopped (e.g., by pressing Ctrl+C).
$ go mod init example.com go: creating new go.mod: module example.com go: to add module requirements and sums: go mod tidy $ go mod tidy go: finding module for package github.com/gin-gonic/gin go: downloading github.com/gin-gonic/gin v1.9.1 go: found github.com/gin-gonic/gin in github.com/gin-gonic/gin v1.9.1 go: downloading github.com/gin-contrib/sse v0.1.0 go: downloading github.com/mattn/go-isatty v0.0.19 go: downloading golang.org/x/net v0.10.0 go: downloading github.com/stretchr/testify v1.8.3 go: downloading google.golang.org/protobuf v1.30.0 go: downloading github.com/bytedance/sonic v1.9.1 go: downloading github.com/goccy/go-json v0.10.2 go: downloading github.com/json-iterator/go v1.1.12 go: downloading github.com/go-playground/validator/v10 v10.14.0 go: downloading github.com/pelletier/go-toml/v2 v2.0.8 go: downloading github.com/ugorji/go/codec v1.2.11 go: downloading gopkg.in/yaml.v3 v3.0.1 go: downloading golang.org/x/sys v0.8.0 go: downloading github.com/davecgh/go-spew v1.1.1 go: downloading github.com/pmezard/go-difflib v1.0.0 go: downloading github.com/modern-go/concurrent v0.0.0-20180306012644-bacd9c7ef1dd go: downloading github.com/modern-go/reflect2 v1.0.2 go: downloading github.com/gabriel-vasile/mimetype v1.4.2 go: downloading github.com/go-playground/universal-translator v0.18.1 go: downloading github.com/leodido/go-urn v1.2.4 go: downloading golang.org/x/crypto v0.9.0 go: downloading golang.org/x/text v0.9.0 go: downloading github.com/go-playground/locales v0.14.1 go: downloading github.com/chenzhuoyu/base64x v0.0.0-20221115062448-fe3a3abad311 go: downloading golang.org/x/arch v0.3.0 go: downloading github.com/twitchyliquid64/golang-asm v0.15.1 go: downloading github.com/klauspost/cpuid/v2 v2.2.4 go: downloading github.com/go-playground/assert/v2 v2.2.0 go: downloading github.com/google/go-cmp v0.5.5 go: downloading gopkg.in/check.v1 v0.0.0-20161208181325-20d25e280405 go: downloading golang.org/x/xerrors v0.0.0-20191204190536-9bdfabe68543
$ go run main.go [GIN-debug] [WARNING] Creating an Engine instance with the Logger and Recovery middleware already attached. [GIN-debug] [WARNING] Running in "debug" mode. Switch to "release" mode in production. - using env: export GIN_MODE=release - using code: gin.SetMode(gin.ReleaseMode) [GIN-debug] GET /resource --> main.main.func1 (3 handlers) [GIN-debug] [WARNING] You trusted all proxies, this is NOT safe. We recommend you to set a value. Please check https://pkg.go.dev/github.com/gin-gonic/gin#readme-don-t-trust-all-proxies for details. [GIN-debug] Environment variable PORT is undefined. Using port :8080 by default [GIN-debug] Listening and serving HTTP on :8080
Once the service is running, we can test the endpoint using a REST HTTP client, in our case, thunder client.
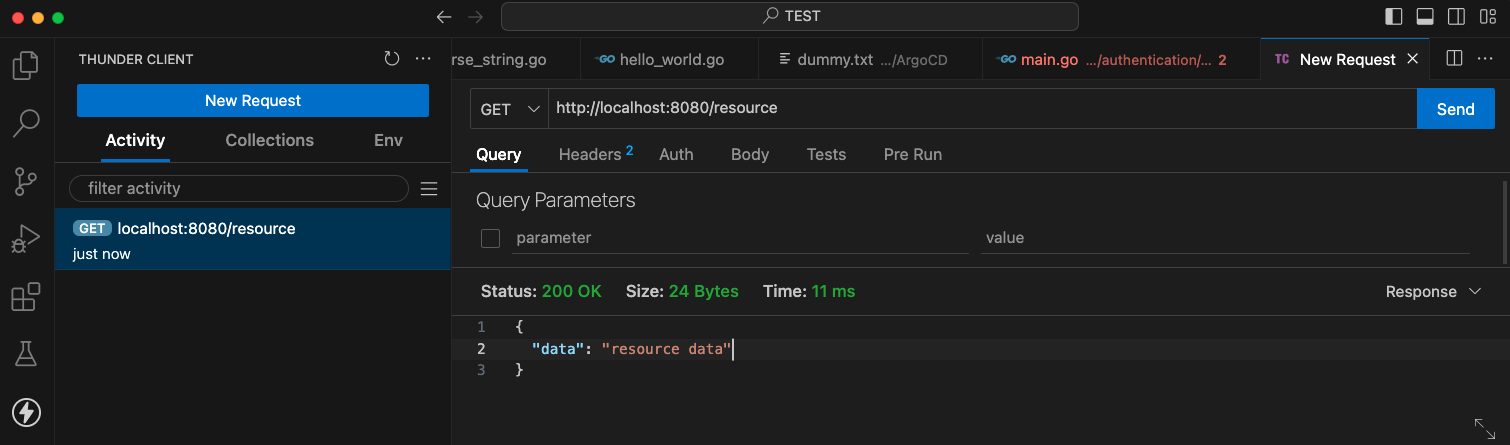
Basic Authentication (BasicAuth) is a simple authentication scheme built into the HTTP protocol.
It's important to note that Basic Authentication transmits the username and password in an easily decodable base64-encoded format. While this provides a basic level of security, it is not considered secure for transmitting sensitive information over an unencrypted connection. Therefore, it is often recommended to use Basic Authentication only over HTTPS (HTTP Secure) to encrypt the communication between the client and the server.
Note that many web applications and APIs use more secure authentication methods, such as OAuth or token-based authentication, especially when dealing with sensitive data.
Add the BasicAuth to the code with Gin:
func main() { // Create a new Gin router with default middleware r := gin.Default() // Define a GET endpoint at "/resource" with Basic Authentication r.GET("/resource", gin.BasicAuth(gin.Accounts{ "admin": "secret", }), func(c *gin.Context) { // Handler function for the "/resource" endpoint c.JSON(http.StatusOK, gin.H{ "data": "resource data", }) }) // Run the server and listen on :8080 r.Run() // Listening and serving HTTP on :8080 }
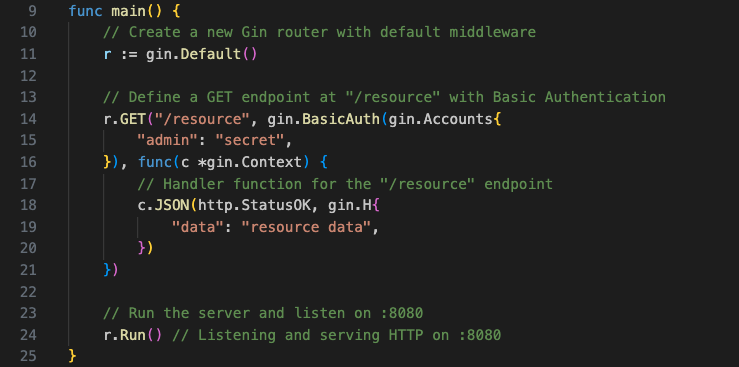
- Endpoint Definition:
r.GET("/resource", ...)
It defines a route for handling HTTP GET requests at the path /resource endpoint. This means that when a client makes a GET request to the /resource endpoint path on the server, the following code will be executed. - Basic Authentication Middleware:
gin.BasicAuth(gin.Accounts{ "admin": "secret", })
This line sets up basic authentication middleware for the /resource endpoint. It uses thegin.BasicAuth
middleware provided by the Gin framework. Thegin.Accounts
map specifies the allowed username ("admin") and its corresponding password ("secret"). This means that clients attempting to access the /resource endpoint must provide the correct username and password in the HTTP headers to authenticate. - Endpoint Handler Function:
func(c *gin.Context) { // Handler function for the "/resource" endpoint c.JSON(http.StatusOK, gin.H{ "data": "resource data", }) }
This is the handler function that will be executed when a client successfully authenticates and accesses the /resource endpoint. In this case, it returns a JSON response with a simple data field. We might replace this code with the actual logic for handling the resource request.
The Basic auth header can be added by simply following the URL pattern http://admin:secret@0.0.0.0:8080/resource:
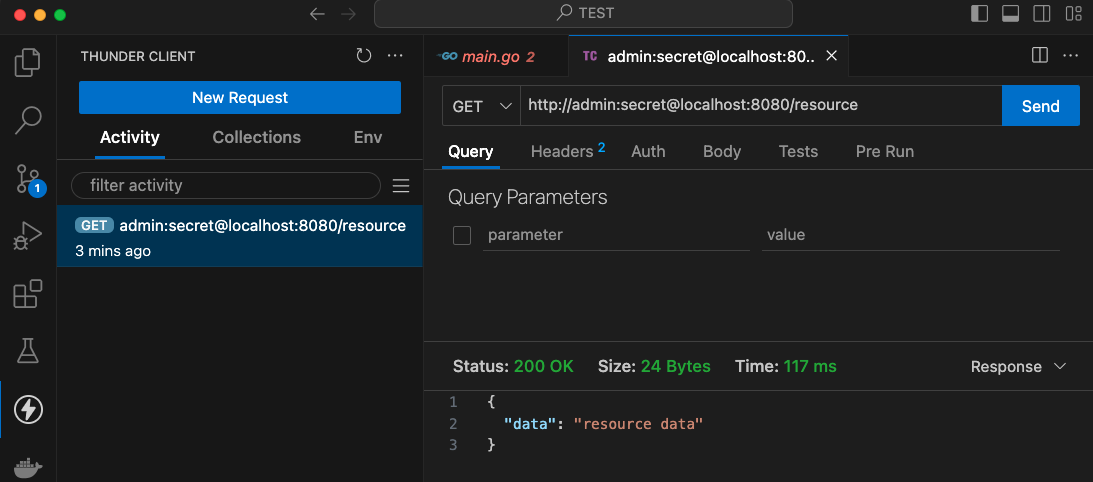
We could use curl
as well:
$ echo -n "admin:secret" | base64 YWRtaW46c2VjcmV0 $ curl -v -H "Authorization: Basic YWRtaW46c2VjcmV0" http://localhost:8080/resource * Trying 127.0.0.1:8080... * Connected to localhost (127.0.0.1) port 8080 (#0) > GET /resource HTTP/1.1 > Host: localhost:8080 > User-Agent: curl/8.1.2 > Accept: */* > Authorization: Basic YWRtaW46c2VjcmV0 > < HTTP/1.1 200 OK < Content-Type: application/json; charset=utf-8 < Date: Mon, 20 Nov 2023 23:48:49 GMT < Content-Length: 24 < * Connection #0 to host localhost left intact
"Bearer-Token-Based Authentication" is often referred to simply as "Bearer Authentication" or "Token Authentication."
It is part of the OAuth 2.0 standard and relies on the use of bearer tokens to authenticate requests. Here's an overview of how Bearer Token-Based Authentication works:
- Token Generation: A user authenticates with a server (authentication server) using their credentials. Upon successful authentication, the server issues a token to the client. This token is often a JSON Web Token (JWT) or a similar format.
- Token Inclusion:
The client includes this token in the HTTP headers of its requests when accessing protected resources on a server.
The token is typically included in the "Authorization" header using the "Bearer" authentication scheme. For example:
Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJpc3MiOiJodHRwczovL2V4YW1w
- Bearer Token Usage: The server, upon receiving a request with a bearer token, validates the token to ensure its authenticity and integrity. If the token is valid and has the necessary permissions, the server grants access to the requested resource.
- Stateless Authentication: Bearer Token-Based Authentication is often stateless, meaning the server does not need to store any information about the client session. The entire authentication context is encoded within the token itself.
- Expiration and Refresh: Bearer tokens typically have an expiration time. Clients may need to obtain a new token by refreshing it with the authentication server when the current token expires.
With Bearer Token Authentication, the username and password are utilized only once during the initial authentication process to obtain the token. After that, the token is used for subsequent requests. This is more secure than sending the username and password with every request. Bearer tokens can be easily revoked if they are compromised or leaked. This provides a mechanism for preventing unauthorized access even if a token falls into the wrong hands. Revoking a token invalidates it, requiring the client to obtain a new token for continued access.
Bearer tokens are usually random strings, and the server cannot decode them to extract information about the token owner. Unlike some other authentication methods, the token itself does not carry detailed information about the user or client. So, to retrieve information about the token owner, such as user details or permissions, the server may need to perform an additional query to a user database or authentication server. This introduces an extra step compared to authentication methods where user details are directly encoded in the token.
main.go:
package main import ( "crypto/rand" "encoding/hex" "net/http" "strings" "github.com/gin-gonic/gin" ) var tokens []string func main() { r := gin.Default() r.POST("/login", gin.BasicAuth(gin.Accounts{ "admin": "secret", }), func(c *gin.Context) { token, _ := randomHex(20) tokens = append(tokens, token) c.JSON(http.StatusOK, gin.H{ "token": token, }) }) r.GET("/resource", func(c *gin.Context) { bearerToken := c.Request.Header.Get("Authorization") reqToken := strings.Split(bearerToken, " ")[1] for _, token := range tokens { if token == reqToken { c.JSON(http.StatusOK, gin.H{ "data": "resource data", }) return } } c.JSON(http.StatusUnauthorized, gin.H{ "message": "unauthorized", }) }) r.Run() // Listen and serve on 0.0.0.0:8080 } func randomHex(n int) (string, error) { bytes := make([]byte, n) if _, err := rand.Read(bytes); err != nil { return "", err } return hex.EncodeToString(bytes), nil }
- Import packages:
import ( "crypto/rand" "encoding/hex" "github.com/gin-gonic/gin" "net/http" "strings" )
The code imports necessary packages, including "crypto/rand" for secure random number generation, "encoding/hex" for hexadecimal encoding, "github.com/gin-gonic/gin" for the Gin web framework, and "net/http" for HTTP-related functionality. - Global Variable:
var tokens []string
The tokens slice is used to store generated tokens. In a production environment, however, we would likely use a more secure and persistent storage mechanism. - Main Function:
func main() { r := gin.Default()
The main function initializes a new instance of the Gin router with default middleware. - Login Endpoint:
r.POST("/login", gin.BasicAuth(gin.Accounts{ "admin": "secret", }), func(c *gin.Context) { token, _ := randomHex(20) tokens = append(tokens, token) c.JSON(http.StatusOK, gin.H{ "token": token, }) })
This code defines a "/login" POST endpoint protected with basic authentication. The provided username and password are "admin" and "secret". Upon successful authentication, a random 20-byte hexadecimal token is generated using the randomHex function. The generated token is added to the tokens slice and returned in the JSON response. - Resource Endpoint:
r.GET("/resource", func(c *gin.Context) { bearerToken := c.Request.Header.Get("Authorization") reqToken := strings.Split(bearerToken, " ")[1] for _, token := range tokens { if token == reqToken { c.JSON(http.StatusOK, gin.H{ "data": "resource data", }) return } } c.JSON(http.StatusUnauthorized, gin.H{ "message": "unauthorized", }) })
The "/resource" GET endpoint checks for a valid token in the request's Authorization header. If a valid token is found in the tokens slice, it responds with a JSON containing "resource data". Otherwise, it returns a 401 Unauthorized status. - Run the Server:
r.Run() // Listen and serve on 0.0.0.0:8080 }
The server is started, listening on port 8080. - Random Hex Function:
func randomHex(n int) (string, error) { bytes := make([]byte, n) if _, err := rand.Read(bytes); err != nil { return "", err } return hex.EncodeToString(bytes), nil }
The server is started, listening on port 8080.
The randomHex function generates a random byte slice of length n and converts it to a hexadecimal string using hex.EncodeToString.
$ go mod init example.com $ go mod tidy $ go run main.go [GIN-debug] [WARNING] Creating an Engine instance with the Logger and Recovery middleware already attached. [GIN-debug] [WARNING] Running in "debug" mode. Switch to "release" mode in production. - using env: export GIN_MODE=release - using code: gin.SetMode(gin.ReleaseMode) [GIN-debug] POST /login --> main.main.func1 (4 handlers) [GIN-debug] GET /resource --> main.main.func2 (3 handlers) [GIN-debug] [WARNING] You trusted all proxies, this is NOT safe. We recommend you to set a value. Please check https://pkg.go.dev/github.com/gin-gonic/gin#readme-don-t-trust-all-proxies for details. [GIN-debug] Environment variable PORT is undefined. Using port :8080 by default [GIN-debug] Listening and serving HTTP on :8080
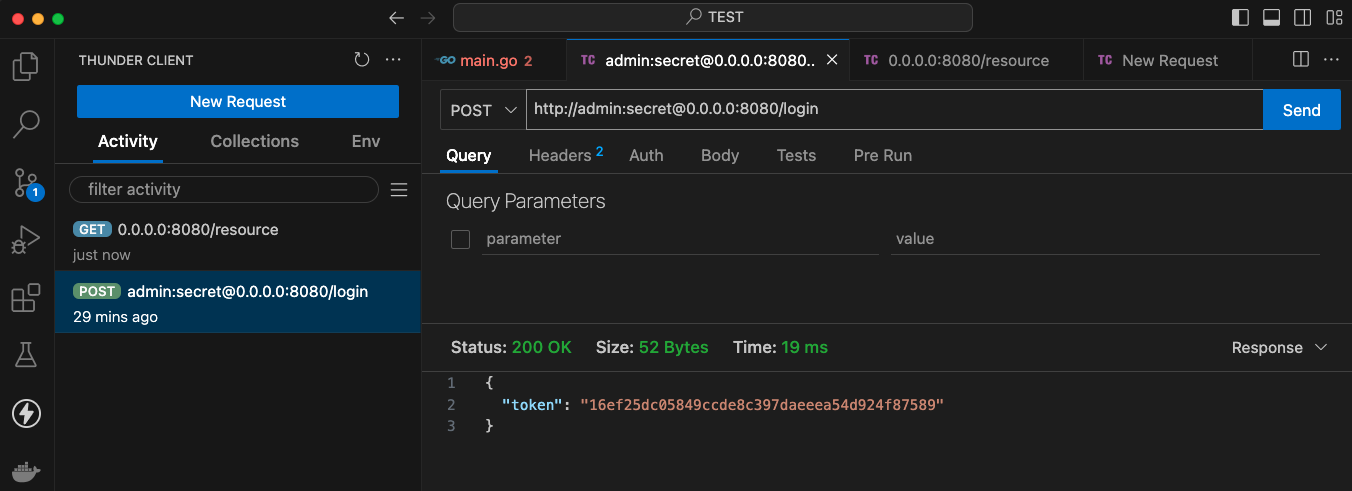
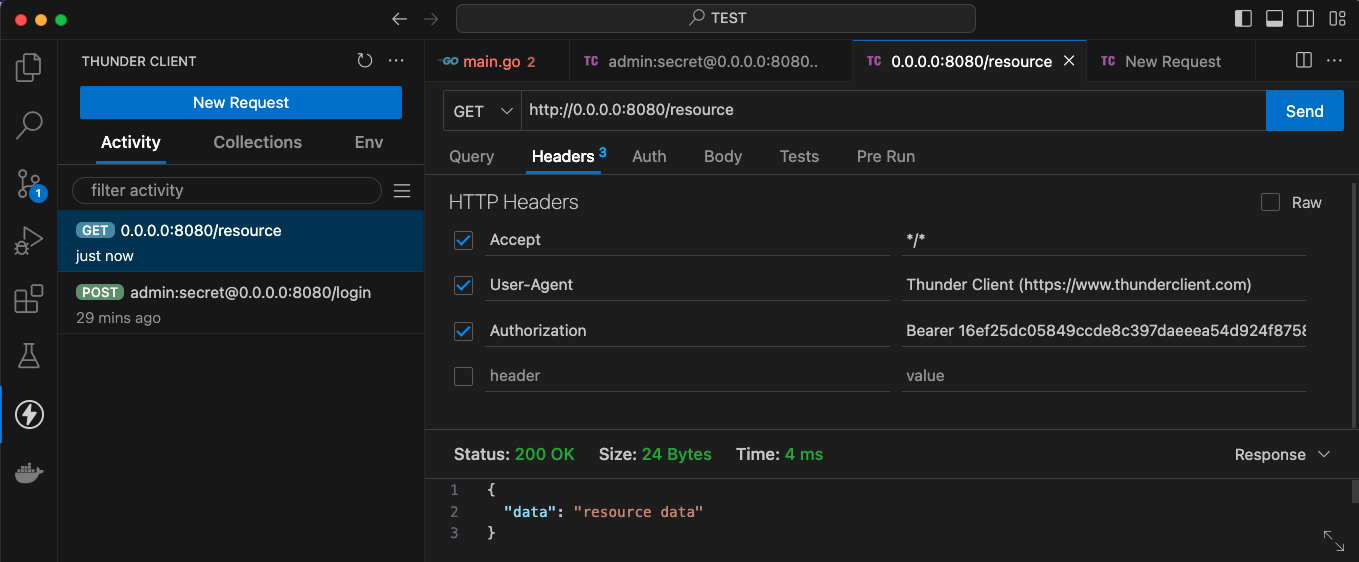
First, we make a POST request to the /login endpoint to retrieve the token, which we save using the response handler script:
POST http://admin:secret@0.0.0.0:8080/login
Then, we use the saved token to request the resource by adding the token to the header:
GET http://0.0.0.0:8080/resource Authorization: Bearer {{auth_token}}
Source: https://github.com/Einsteinish/go_tutorial/tree/main/authentication
Continued to Go Application Authentication I (BasicAuth, Bearer-Token-Based Authentication).
Go Tutorial
- GoLang Tutorial - HelloWorld
- Calling code in an external package & go.mod / go.sum files
- Workspaces
- Workspaces II
- Visual Studio Code
- Data Types and Variables
- byte and rune
- Packages
- Functions
- Arrays and Slices
- A function taking and returning a slice
- Conditionals
- Loops
- Maps
- Range
- Pointers
- Closures and Anonymous Functions
- Structs and receiver methods
- Value or Pointer Receivers
- Interfaces
- Web Application Part 0 (Introduction)
- Web Application Part 1 (Basic)
- Web Application Part 2 (Using net/http)
- Web Application Part 3 (Adding "edit" capability)
- Web Application Part 4 (Handling non-existent pages and saving pages)
- Web Application Part 5 (Error handling and template caching)
- Web Application Part 6 (Validating the title with a regular expression)
- Web Application Part 7 (Function Literals and Closures)
- Building Docker image and deploying Go application to a Kubernetes cluster (minikube)
- Serverless Framework (Serverless Application Model-SAM)
- Serverless Web API with AWS Lambda
- Arrays vs Slices with an array left rotation sample
- Variadic Functions
- Goroutines
- Channels ("<-")
- Channels ("<-") with Select
- Channels ("<-") with worker pools
- Defer
- GoLang Panic and Recover
- String Formatting
- JSON
- SQLite
- Modules 0: Using External Go Modules from GitHub
- Modules 1 (Creating a new module)
- Modules 2 (Adding Dependencies)
- AWS SDK for Go (S3 listing)
- Linked List
- Binary Search Tree (BST) Part 1 (Tree/Node structs with insert and print functions)
- Go Application Authentication I (BasicAuth, Bearer-Token-Based Authentication)
- Go Application Authentication II (JWT Authentication)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization