GoLang Tutorial - String formatting
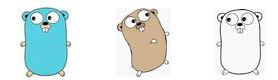
This post will show some examples of GoLang's common string formatting (Printf()). So many options and quite often we wonder which one to use.
The complete guide can be found Package fmt.
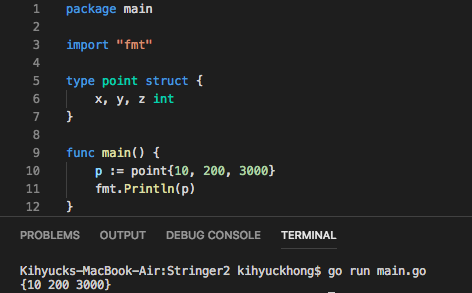
If the value is a struct, the %+v variant will include the struct's field names:
fmt.Printf("%v\n", p) fmt.Printf("%+v\n", p)
{10 200 3000} {x:10 y:200 z:3000}
The %#v variant prints a Go syntax representation of the value, i.e. the source code snippet that would produce that value:
fmt.Printf("%#v\n", p)
main.point{x:10, y:200, z:3000}
To print the type of a value, use %T:
fmt.Printf("%T\n", p)
main.point
Formatting booleans, use %t:
var b bool b = true fmt.Printf("%t\n", b)
true
For integer, use %d for standard, base-10 formatting:
fmt.Printf("%d\n", 12335)
12345
binary representation of an integer, use %b:
fmt.Printf("%b\n", 9)
1001
To prints the character corresponding to the given integer, use %c:
fmt.Printf("%c\n", 89)
Y
%x provides hex encoding., use %x:
fmt.Printf("%x\n", 1501)
5dd
For float, use %f:
fmt.Printf("%f\n", 3.141592)
3.141592
%e and %E format the float in scientific notation:
fmt.Printf("%e\n", math.Pow(-9, 3)) fmt.Printf("%E\n", math.Pow(-9, 3))
-7.290000e+02 -7.290000E+02
For basic string, use %s:
fmt.Printf("%s\n", "string format")
string format
To double-quote a string, use %q:
fmt.Printf("%s\n", "quoted") fmt.Printf("%s\n", "\"quoted\"") fmt.Printf("%q\n", "\"quoted\"")
quoted "quoted" "\"quoted\""
As with integers seen earlier, %x renders the string in base-16, with two output characters per byte of input:
fmt.Printf("%x\n", "hex this") fmt.Printf("%x\n", "h") fmt.Printf("%x\n", "e") fmt.Printf("%x\n", "x") fmt.Printf("%x\n", " ")
6865782074686973 68 65 78 20 ...
To print a representation of a pointer, use %p:
fmt.Printf("%p\n", &p)
0xc000014200
Go Tutorial
- GoLang Tutorial - HelloWorld
- Calling code in an external package & go.mod / go.sum files
- Workspaces
- Workspaces II
- Visual Studio Code
- Data Types and Variables
- byte and rune
- Packages
- Functions
- Arrays and Slices
- A function taking and returning a slice
- Conditionals
- Loops
- Maps
- Range
- Pointers
- Closures and Anonymous Functions
- Structs and receiver methods
- Value or Pointer Receivers
- Interfaces
- Web Application Part 0 (Introduction)
- Web Application Part 1 (Basic)
- Web Application Part 2 (Using net/http)
- Web Application Part 3 (Adding "edit" capability)
- Web Application Part 4 (Handling non-existent pages and saving pages)
- Web Application Part 5 (Error handling and template caching)
- Web Application Part 6 (Validating the title with a regular expression)
- Web Application Part 7 (Function Literals and Closures)
- Building Docker image and deploying Go application to a Kubernetes cluster (minikube)
- Serverless Framework (Serverless Application Model-SAM)
- Serverless Web API with AWS Lambda
- Arrays vs Slices with an array left rotation sample
- Variadic Functions
- Goroutines
- Channels ("<-")
- Channels ("<-") with Select
- Channels ("<-") with worker pools
- Defer
- GoLang Panic and Recover
- String Formatting
- JSON
- SQLite
- Modules 0: Using External Go Modules from GitHub
- Modules 1 (Creating a new module)
- Modules 2 (Adding Dependencies)
- AWS SDK for Go (S3 listing)
- Linked List
- Binary Search Tree (BST) Part 1 (Tree/Node structs with insert and print functions)
- Go Application Authentication I (BasicAuth, Bearer-Token-Based Authentication)
- Go Application Authentication II (JWT Authentication)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization