GoLang Tutorial - Structs and receiver methods
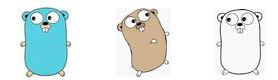
A struct is just a collect of fields.
In the following code, we define a struct called "Person", and then initialize it in two ways.
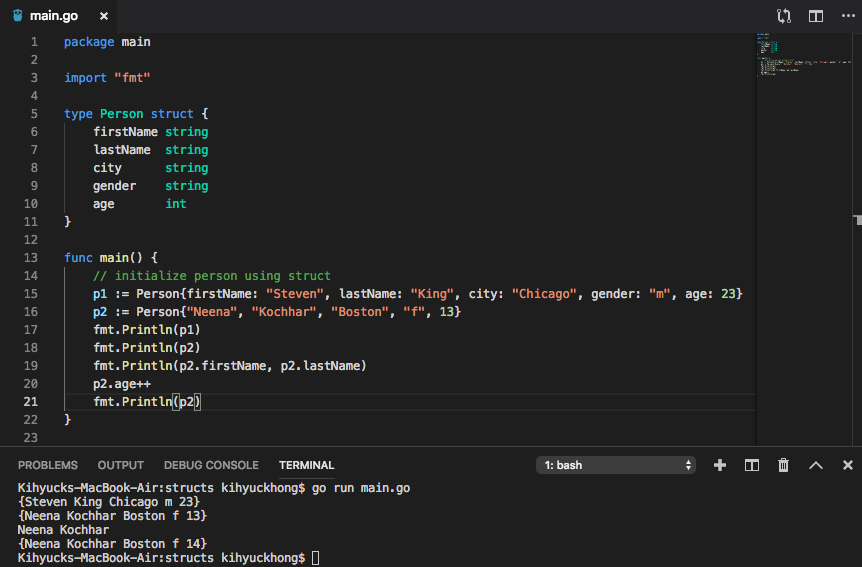
Note that we can not only access a specific field of a struct but also we can modify the value of any field.
Go does not have classes. However, we can define methods on types.
A method is a function with a special receiver argument.
The following example shows how we use (or create) the method of the struct.
In the code, we defined a method called (p Person) hello():
func (p Person) hello() {}
It is a receiver in Go terms and the syntax looks like this:

It's a Go way of creating a method for a struct!
As commented in the code below, it's a value type. We'll soon see a pointer type receiver like this:
func (p *Person) hasBirthday() {}
Here is our full code:
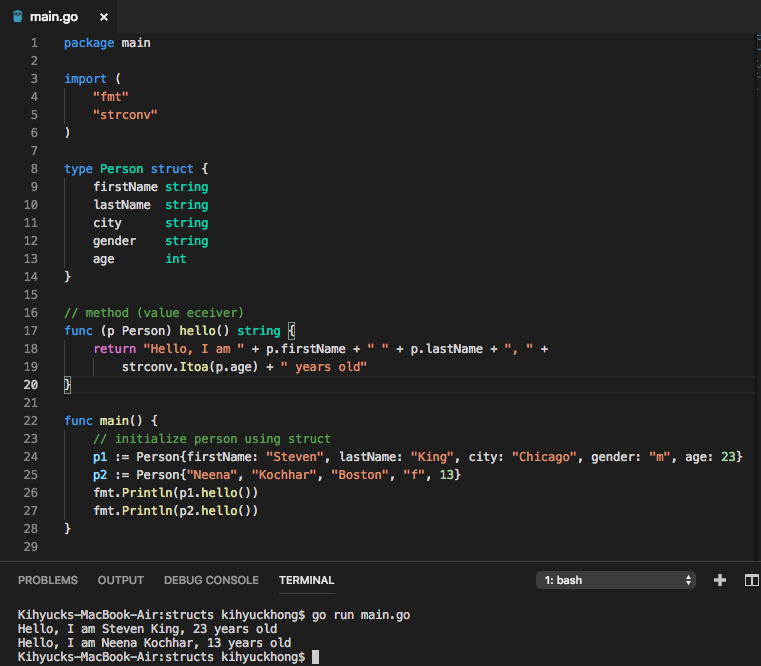
The next example shows how we modified the data of the struct. In the code, we defined a method (receiver) called (p *Person) hasBirthday(). Note that it's taking a pointer:
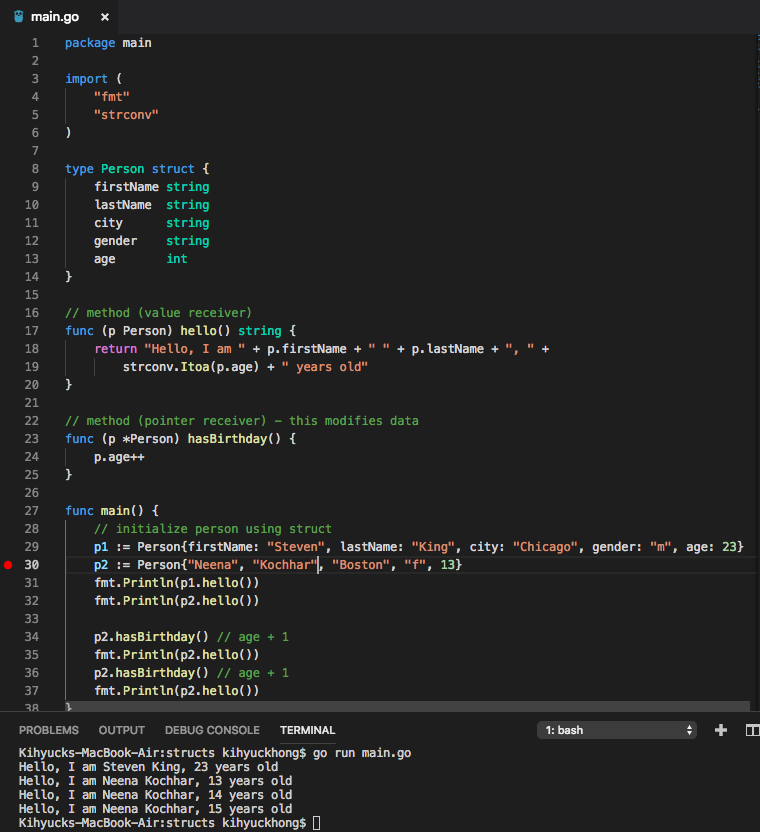
Another example of a receiver:
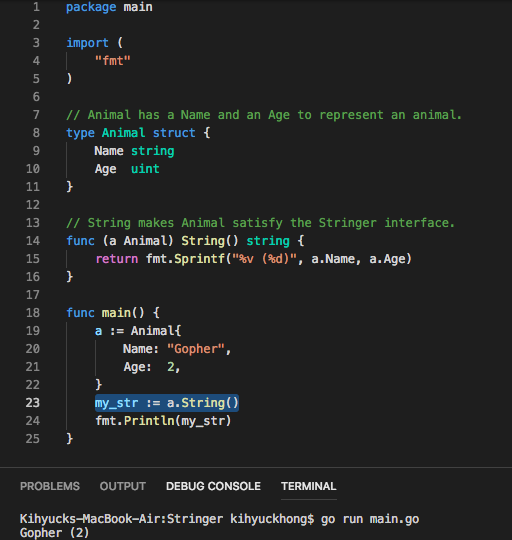
The code: stringer.go
We made a method called String() which enables us to customize print for the Animal struct.
Similar to the previous example. This time, we customize the IP-addresses:
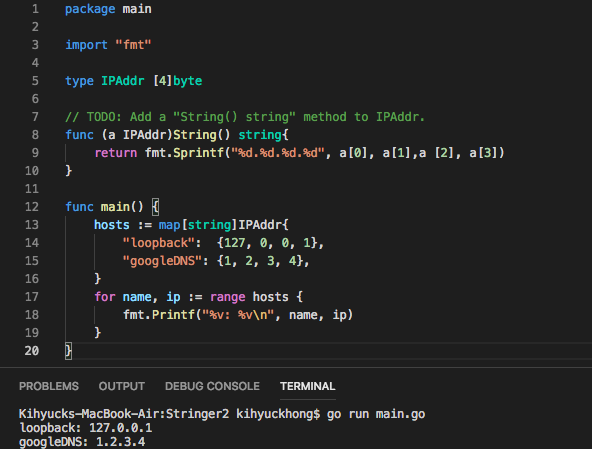
The code: ip_addr.go
We made a method called String() which enables us to customize print for the [4]byte array. For instance, IPAddr{1, 2, 3, 4} will be print as "1.2.3.4".
The following is an example code showing one of the ways of constructing a struct.
First, it creates an empty slice of struct pointers, create a struct struct pointer, filled in the elements of the struct, then finally attach the struct pointer to the list of pointers:
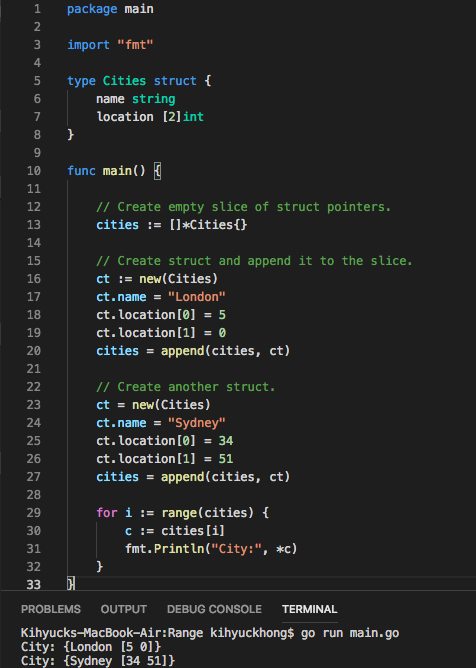
The code: city-struct.go
Go Tutorial
- GoLang Tutorial - HelloWorld
- Calling code in an external package & go.mod / go.sum files
- Workspaces
- Workspaces II
- Visual Studio Code
- Data Types and Variables
- byte and rune
- Packages
- Functions
- Arrays and Slices
- A function taking and returning a slice
- Conditionals
- Loops
- Maps
- Range
- Pointers
- Closures and Anonymous Functions
- Structs and receiver methods
- Value or Pointer Receivers
- Interfaces
- Web Application Part 0 (Introduction)
- Web Application Part 1 (Basic)
- Web Application Part 2 (Using net/http)
- Web Application Part 3 (Adding "edit" capability)
- Web Application Part 4 (Handling non-existent pages and saving pages)
- Web Application Part 5 (Error handling and template caching)
- Web Application Part 6 (Validating the title with a regular expression)
- Web Application Part 7 (Function Literals and Closures)
- Building Docker image and deploying Go application to a Kubernetes cluster (minikube)
- Serverless Framework (Serverless Application Model-SAM)
- Serverless Web API with AWS Lambda
- Arrays vs Slices with an array left rotation sample
- Variadic Functions
- Goroutines
- Channels ("<-")
- Channels ("<-") with Select
- Channels ("<-") with worker pools
- Defer
- GoLang Panic and Recover
- String Formatting
- JSON
- SQLite
- Modules 0: Using External Go Modules from GitHub
- Modules 1 (Creating a new module)
- Modules 2 (Adding Dependencies)
- AWS SDK for Go (S3 listing)
- Linked List
- Binary Search Tree (BST) Part 1 (Tree/Node structs with insert and print functions)
- Go Application Authentication I (BasicAuth, Bearer-Token-Based Authentication)
- Go Application Authentication II (JWT Authentication)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization