GoLang Tutorial - HelloWorld
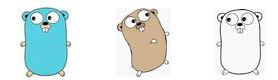
In 2009, Google made Go, often called Golang. It's like C, being statically typed and explicit. Go is known for quick startup and low runtime overhead. People love using it for microservices and concurrent programming.
Download an appropriate package from https://go.dev/doc/install.

The package installs the Go distribution to /usr/local/go. The package should put the /usr/local/go/bin directory in our PATH environment variable.
export PATH=$PATH:/usr/local/go/bin
$ go version go version go1.21.4 darwin/amd64
To try out GoLang, follow these steps to ensure that Go is installed correctly and set up a workspace by building a simple program:
- Check Go Installation: Verify that Go is installed correctly on your system.
- Set Up Workspace:
Create a workspace directory. For example, we can use the following command to create a directory named go in our home directory:
$ mkdir -p $HOME/go
This will be our Go workspace directory. - hello.go:
package main import "fmt" func main() { fmt.Println("Hello, world!") }
Now we're ready to proceed with building a simple program in our newly set up Go workspace.
We should use go mod init
command to create a Go module for our hello world code.
This will make it easier to manage our dependencies and share our code with others:
$ go mod init example/hello go: creating new go.mod: module example/hello go: to add module requirements and sums: go mod tidy
The go mod init
command in Go is used to initialize a new module.
A module is a collection of related Go packages that are versioned together as a single unit.
The go mod init
command is typically run at the root of our project to create or initialize a go.mod file,
which contains information about the module and its dependencies.
The example/hello is the module path. It uniquely identifies our module and its location in our version control system. It is common practice to use a path that resembles the directory structure of our project. I n this case, it suggests that our project is inside a directory named example, and the module is named hello.
Navigate to the directory where we saved the hello.go file and run the following command to build our code:
$ go build
Then, run the following command to run our code:
$ ./hello
We should see the following output:
Hello, world!
We have written and run our first Golang code!
Well, we can do a little bit more, adding time:
package main import ( "fmt" "time" ) func main() { fmt.Println("Hello, world!") fmt.Println("The time is ", time.Now().Format("2006-01-02 15:04:05")) }
Note that we're formating time.Time type func() using the appropriate format placeholders corresponding to the components of the time we want to display, and these placeholders are fixed. Actually, in the time package in Go, the reference time for formatting dates and times is January 2, 2006, at 15:04:05 (3:04:05 PM) MST. This specific date and time were chosen by the creators of Go as a memorable and easy-to-remember reference point for formatting purposes.
Also note that we're importing "time" package.
Modules vs Packages:
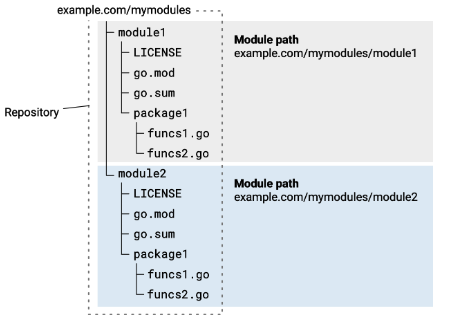
Go Tutorial
- GoLang Tutorial - HelloWorld
- Calling code in an external package & go.mod / go.sum files
- Workspaces
- Workspaces II
- Visual Studio Code
- Data Types and Variables
- byte and rune
- Packages
- Functions
- Arrays and Slices
- A function taking and returning a slice
- Conditionals
- Loops
- Maps
- Range
- Pointers
- Closures and Anonymous Functions
- Structs and receiver methods
- Value or Pointer Receivers
- Interfaces
- Web Application Part 0 (Introduction)
- Web Application Part 1 (Basic)
- Web Application Part 2 (Using net/http)
- Web Application Part 3 (Adding "edit" capability)
- Web Application Part 4 (Handling non-existent pages and saving pages)
- Web Application Part 5 (Error handling and template caching)
- Web Application Part 6 (Validating the title with a regular expression)
- Web Application Part 7 (Function Literals and Closures)
- Building Docker image and deploying Go application to a Kubernetes cluster (minikube)
- Serverless Framework (Serverless Application Model-SAM)
- Serverless Web API with AWS Lambda
- Arrays vs Slices with an array left rotation sample
- Variadic Functions
- Goroutines
- Channels ("<-")
- Channels ("<-") with Select
- Channels ("<-") with worker pools
- Defer
- GoLang Panic and Recover
- String Formatting
- JSON
- SQLite
- Modules 0: Using External Go Modules from GitHub
- Modules 1 (Creating a new module)
- Modules 2 (Adding Dependencies)
- AWS SDK for Go (S3 listing)
- Linked List
- Binary Search Tree (BST) Part 1 (Tree/Node structs with insert and print functions)
- Go Application Authentication I (BasicAuth, Bearer-Token-Based Authentication)
- Go Application Authentication II (JWT Authentication)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization