GoLang Tutorial - Arrays vs Slices with an array left rotation sample
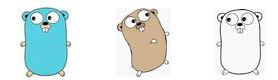
We looked at arrays and slices separately in Arrays and Slices.
So, what are the differences?
When we write a go program, for most the cases, we use slices instead of arrays.
An array is fixed in size. But slices can be dynamic: the number of elements in a slice can grow dynamically. Keep in mind that slice uses array behind the curtain but slice by itself cannot store any data. So, we can think of slice like a reference to an array. All it does is to play with just a portion of the underlying array.
Let's look at the following diagram:
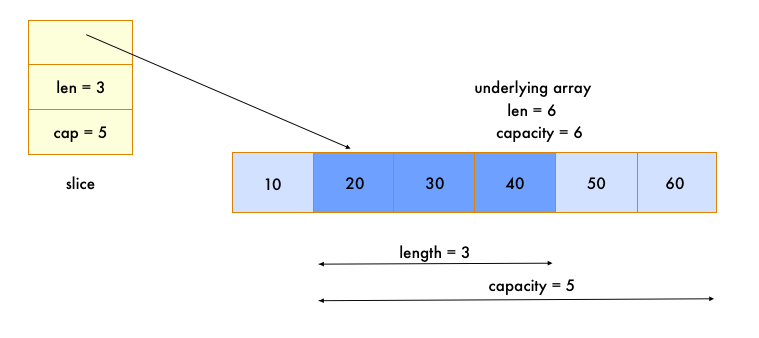
The length of a slice is the length of the segment of the array that the slice contains. The capacity is the maximum size up to which the segment can grow. In our example, the capacity is the number of elements in the underlying array starting from the first element in the slice. So, it is 5.
The code for the diagram looks like this:
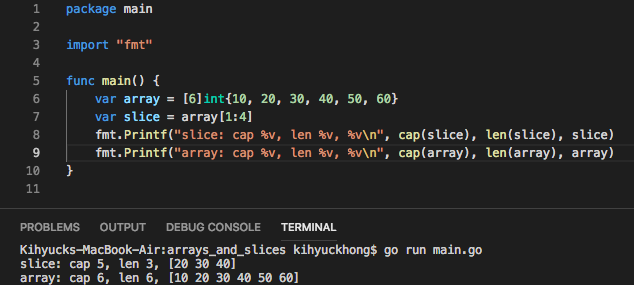
The code: slice-array.go
Golang provides a library function called make() for creating slices. Following is the signature:
func make([]T, len, cap) []T
The make() function takes a type, a length, and an optional capacity. It allocates an underlying array with size equal to the given capacity, and returns a slice that refers to that array:
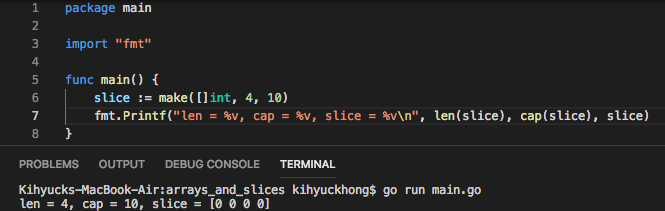
The code creates an array of size 10, slices it till index 4, and returns the slice reference.
The capacity parameter in the make() function is optional. When skipped, it defaults to the specified length:
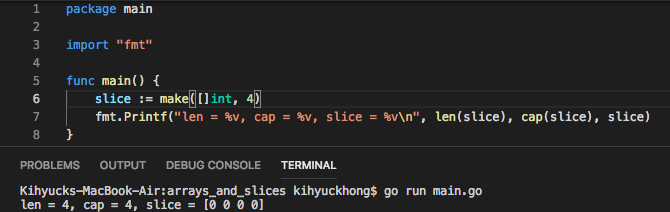
Try to write a code that does rotating an array. We'll find ourselves end up using slices instead of arrays.
Here is the code:
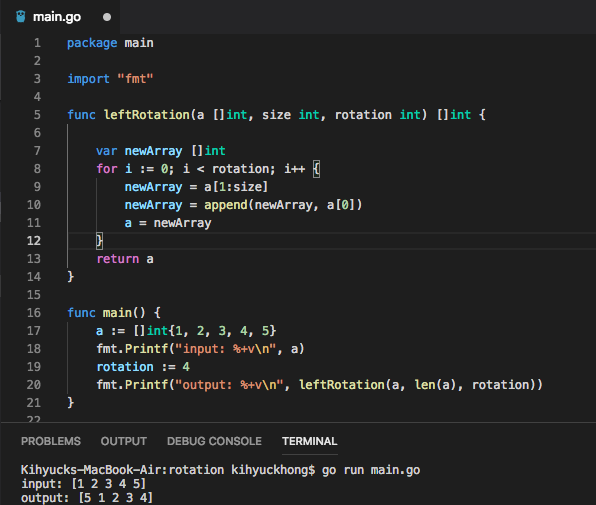
The code: left_rotation.go
Go Tutorial
- GoLang Tutorial - HelloWorld
- Calling code in an external package & go.mod / go.sum files
- Workspaces
- Workspaces II
- Visual Studio Code
- Data Types and Variables
- byte and rune
- Packages
- Functions
- Arrays and Slices
- A function taking and returning a slice
- Conditionals
- Loops
- Maps
- Range
- Pointers
- Closures and Anonymous Functions
- Structs and receiver methods
- Value or Pointer Receivers
- Interfaces
- Web Application Part 0 (Introduction)
- Web Application Part 1 (Basic)
- Web Application Part 2 (Using net/http)
- Web Application Part 3 (Adding "edit" capability)
- Web Application Part 4 (Handling non-existent pages and saving pages)
- Web Application Part 5 (Error handling and template caching)
- Web Application Part 6 (Validating the title with a regular expression)
- Web Application Part 7 (Function Literals and Closures)
- Building Docker image and deploying Go application to a Kubernetes cluster (minikube)
- Serverless Framework (Serverless Application Model-SAM)
- Serverless Web API with AWS Lambda
- Arrays vs Slices with an array left rotation sample
- Variadic Functions
- Goroutines
- Channels ("<-")
- Channels ("<-") with Select
- Channels ("<-") with worker pools
- Defer
- GoLang Panic and Recover
- String Formatting
- JSON
- SQLite
- Modules 0: Using External Go Modules from GitHub
- Modules 1 (Creating a new module)
- Modules 2 (Adding Dependencies)
- AWS SDK for Go (S3 listing)
- Linked List
- Binary Search Tree (BST) Part 1 (Tree/Node structs with insert and print functions)
- Go Application Authentication I (BasicAuth, Bearer-Token-Based Authentication)
- Go Application Authentication II (JWT Authentication)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization