GoLang Tutorial - JSON
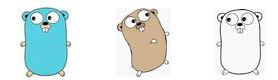
JSON (JavaScript Object Notation) is a simple data interchange format. It is most commonly used for communication between web back-ends and JavaScript programs running in the browser, but it is used in many other places, too.
With the json package it's a snap to read and write JSON data from our Go programs.
func Marshal(v interface{}) ([]byte, error)
The function returns the JSON encoding form of v.
With a given Go data structure, Message:
type Message struct { Name string Body string Time int64 }
and an instance of Message
m := Message{"Interface", "Empty Interface", 1556747623}
We can marshal a JSON-encoded version of m using json.Marshal:
b, err := json.Marshal(m)
If all is well, err will be nil and b will be a []byte containing this JSON data:
b == []byte(`{"Name":"Interface","Body":"Empty Interface","Time":1556747623}`)
Here is the full code:
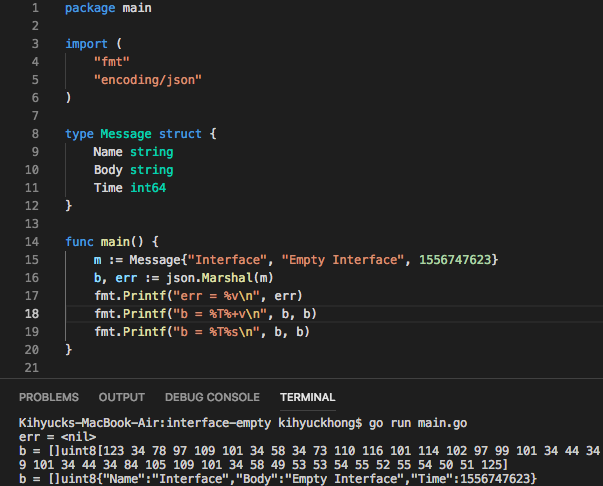
The code: json-marshall.go
To decode JSON data we use the Unmarshal() function:
func Unmarshal(data []byte, v interface{}) error
We must first create a place where the decoded data will be stored:
var m Message
and call json.Unmarshal, passing it a []byte of JSON data and a pointer to m:
err := json.Unmarshal(b, &m)
If b contains valid JSON that fits in m, after the call err will be nil and the data from b will have been stored in the struct m, as if by an assignment like:
m = Message{ Name: "Interface", Body: "Empty Interface", Time: 1556747623, }
Here is the full code:
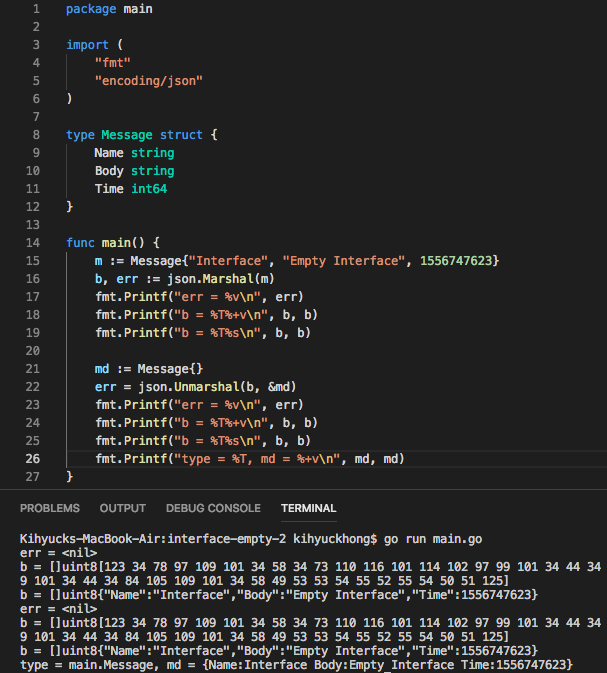
The code: json-unmarshall.go
Go Tutorial
- GoLang Tutorial - HelloWorld
- Calling code in an external package & go.mod / go.sum files
- Workspaces
- Workspaces II
- Visual Studio Code
- Data Types and Variables
- byte and rune
- Packages
- Functions
- Arrays and Slices
- A function taking and returning a slice
- Conditionals
- Loops
- Maps
- Range
- Pointers
- Closures and Anonymous Functions
- Structs and receiver methods
- Value or Pointer Receivers
- Interfaces
- Web Application Part 0 (Introduction)
- Web Application Part 1 (Basic)
- Web Application Part 2 (Using net/http)
- Web Application Part 3 (Adding "edit" capability)
- Web Application Part 4 (Handling non-existent pages and saving pages)
- Web Application Part 5 (Error handling and template caching)
- Web Application Part 6 (Validating the title with a regular expression)
- Web Application Part 7 (Function Literals and Closures)
- Building Docker image and deploying Go application to a Kubernetes cluster (minikube)
- Serverless Framework (Serverless Application Model-SAM)
- Serverless Web API with AWS Lambda
- Arrays vs Slices with an array left rotation sample
- Variadic Functions
- Goroutines
- Channels ("<-")
- Channels ("<-") with Select
- Channels ("<-") with worker pools
- Defer
- GoLang Panic and Recover
- String Formatting
- JSON
- SQLite
- Modules 0: Using External Go Modules from GitHub
- Modules 1 (Creating a new module)
- Modules 2 (Adding Dependencies)
- AWS SDK for Go (S3 listing)
- Linked List
- Binary Search Tree (BST) Part 1 (Tree/Node structs with insert and print functions)
- Go Application Authentication I (BasicAuth, Bearer-Token-Based Authentication)
- Go Application Authentication II (JWT Authentication)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization