PHP Tutorial Laravel 4 Framework (Authentication) - Activating User Account I
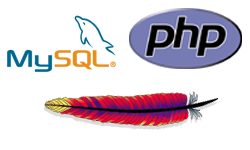
Laravel 4
- Installing on Ubuntu - local
- Installing on a Shared host
- Installing on Windows
- Creating users table
- Home page with controller and blade
- Blade Templating
- Database connection and sending emails
- Creating user account I - GET
- Creating user account II - POST
- Creating user account III - Error checking & redirecting input
- Creating user account IV - User::create()
- Activating user account I - Mail::send()
- Activating user account II - Update user's status
- User account sign-in I - Route and link
- User account sign-in II - Validation
- User account sign-in III - Login Authentication
- Singing out
- Remember the user
- Changing Password I
- Changing Password II
- Recovering forgotten password I
- Recovering forgotten password II
- User Profile
- Database Migration using artisan
This tutorial is the continuation from Creating user account IV - User::create().
Now that the user can create an account, we want to send an email to the user so that the user can activate that account and login.
In this section, we'll see how the code can be passed between uri and activation page:
routes.php:
<?php Route::get('/', array('as' => 'home', 'uses' => 'HomeController@home' )); /* Unauthenticated group */ Route::group(array('before' => 'guest'), function() { /* CSRF protection */ Route::group(array('before' => 'csrf'), function() { /* Create an account (POST) */ Route::post('/account/create', array('as' => 'account-create-post', 'uses' => 'AccountController@postCreate' )); }); /* Create an account (GET) */ Route::get('/account/create', array('as' => 'account-create', 'uses' => 'AccountController@getCreate' )); /* Activate an account */ Route::get('/account/activate/{code}', array('as' => 'account-activate', 'uses' => 'AccountController@getActivate' )); });
The method getActivate() will be defined in AccountController.php file:
<?php class AccountController extends BaseController { /* Viewing the form */ public function getCreate() { return View::make('account.create'); } /* Submitting the form */ public function postCreate() { $validator = Validator::make(Input::all(), array( 'email' => 'required|max:50|email|unique:users', 'username' => 'required|max:20|min:3|unique:users', 'password' => 'required|min:6', 'password_again'=> 'required|same:password' ) ); if($validator->fails()) { return Redirect::route('account-create') ->withErrors($validator) ->withInput(); // fills the field with the old inputs what were correct } else { // create an account $email = Input::get('email'); $username = Input::get('username'); $password = Input::get('password'); // Activation code $code = str_random(60); /* This does the same as User::create() $user = new User; $user->fill(array( 'email' => $email, 'username' => $username, 'password' => Hash::make($password), 'code' => $code, 'active' => 0, )); $userdata = $user->save(); */ // record $userdata = User::create(array( 'email' => $email, 'username' => $username, 'password' => Hash::make($password), 'code' => $code, 'active' => 0 )); if($userdata) { return Redirect::route('home') ->with('global', 'Your account has been created. We have sent you an email to activate your accout'); } } } public function getActivate($code) { return $code; } }
The two codes shows the parameter(
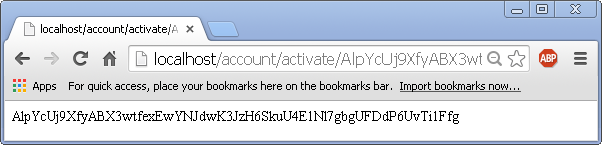
In this section, we'll send an email from K@bogotobogo.com to Dijkstra_Bogo@yahoo.com.
Here is the updated AccountController.php file:
<?php class AccountController extends BaseController { /* Viewing the form */ public function getCreate() { return View::make('account.create'); } /* Submitting the form */ public function postCreate() { $validator = Validator::make(Input::all(), array( 'email' => 'required|max:50|email|unique:users', 'username' => 'required|max:20|min:3|unique:users', 'password' => 'required|min:6', 'password_again'=> 'required|same:password' ) ); if($validator->fails()) { return Redirect::route('account-create') ->withErrors($validator) ->withInput(); // fills the field with the old inputs what were correct } else { // create an account $email = Input::get('email'); $username = Input::get('username'); $password = Input::get('password'); // Activation code $code = str_random(60); /* This does the same as User::create() $user = new User; $user->fill(array( 'email' => $email, 'username' => $username, 'password' => Hash::make($password), 'code' => $code, 'active' => 0, )); $userdata = $user->save(); */ // record $userdata = User::create(array( 'email' => $email, 'username' => $username, 'password' => Hash::make($password), 'code' => $code, 'active' => 0 )); if($userdata) { Mail::send('emails.auth.activate', array('link' => URL::route('account-activate', $code), 'username' => $username), function($message) use ($userdata) { $message->to($userdata->email, $userdata->username)->subject('Activate your account'); }); return Redirect::route('home') ->with('global', 'Your account has been created. We have sent you an email to activate your accout'); } } } public function getActivate($code) { } }
Let's create an account and test if a user can receive the activation mail.
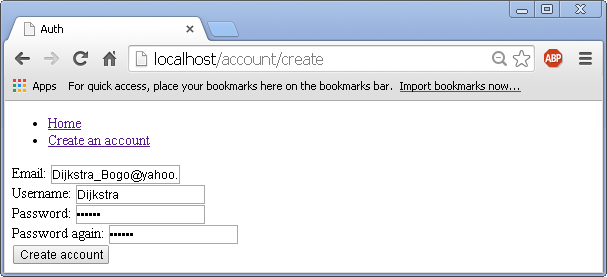
At the "Create account":
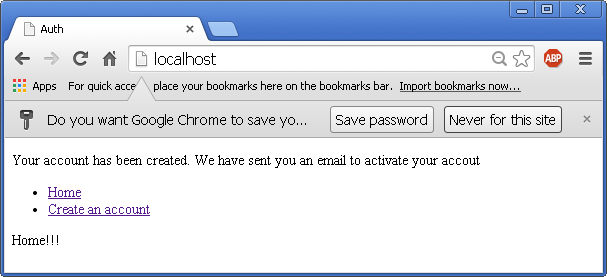
The code (activate.blade.php) for email body looks like this:
Hello {{ $username }}, <br><br> Please activate your account using the following link.<br><br> <br> {{ $link }}
Indeed, I got activation code via my yahoo email:
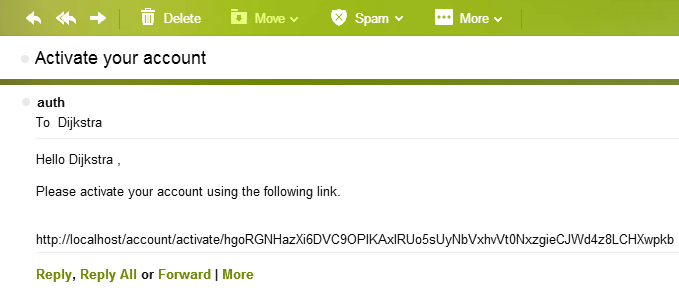
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization