PHP & MySQL Tutorial
json - encode and decode
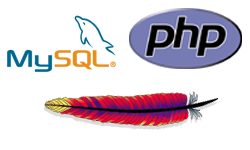
bogotobogo.com site search:
json - encode and decode
JSON (JavaScript Object Notation) is a lightweight data-interchange format.
- It is easy for humans to read and write.
- It is easy for machines to parse and generate.
- It is based on a subset of the JavaScript Programming Language, Standard ECMA-262 3rd Edition - December 1999.
- JSON is a text format that is completely language independent but uses conventions that are familiar to programmers of the C-family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, and many others.
- These properties make JSON an ideal data-interchange language.
- These are universal data structures. Virtually all modern programming languages support them in one form or another.
- It makes sense that a data format that is interchangeable with programming languages also be based on these structures.
JSON is built on two structures:
- A collection of name/value pairs. In various languages, this is realized as an object, record, struct, dictionary, hash table, keyed list, or associative array.
- An ordered list of values. In most languages, this is realized as an array, vector, list, or sequence.
In JSON, they take on these forms:
- An object is an unordered set of name/value pairs. An object begins with { (left brace) and ends with } (right brace). Each name is followed by : (colon) and the name/value pairs are separated by , (comma).
- An array is an ordered collection of values. An array begins with [ (left bracket) and ends with ] (right bracket). Values are separated by , (comma).
Pictures from http://www.json.org/
json - samples
Here are some code samples:
<?php /* object */ echo "\n\nCase 1\n"; $string = '{"audio": "01234", "video":"56789"}'; $obj = json_decode($string); var_dump($obj); echo $obj->{"audio"}; echo "\n"; echo $obj->{"video"}; echo "\n"; /* array */ /* When the second parameter of json_decode is true, returned objects will be converted into associative arrays. */ echo "\n\nCase 2\n"; $stringArr = '{"audio": "01234", "video":"56789"}'; $arr = json_decode($stringArr, true); var_dump($arr); echo $arr{"audio"}; echo "\n"; echo $arr{"video"}; echo "\n"; foreach ($arr as $key => $value) { echo "Key: $key; Value: $value\n"; } echo "\n\nCase 3\n"; $stringArr = '{"audio": {"one": 1, "two": 2, "three": 3, "four": 4}, "video": {"one": 1, "two": 2, "three": 3, "four": 4}}'; var_dump($stringArr); $arr = json_decode($stringArr, true ); echo $arr{"audio"}["one"]; echo "\n"; echo $arr{"video"}["four"]; echo "\n"; foreach ($arr["audio"] as $key => $value) { echo "Key: $key; Value: $value\n"; } foreach ($arr["video"] as $key => $value) { echo "Key: $key; Value: $value\n"; } echo "\n\nCase 4\n"; $stringArr = '{"audio": {"one": [1,2,3], "two": [11,22,33], "three": [111,222,333]}, "video": {"A": [1,2,3], "B": [11,22,33], "C": [111,222,333]}}'; var_dump($stringArr); $arr = json_decode($stringArr, true ); echo $arr{"audio"}["one"][0]; echo "\n"; echo $arr{"video"}["B"][2]; echo "\n"; foreach ($arr["audio"]["one"] as $key => $value) { echo "Key: $key; Value: $value\n"; } foreach ($arr["video"]["B"] as $key => $value) { echo "Key: $key; Value: $value\n"; } $nOne = sizeof($arr['audio']['one']); for($i = 0; $i < $nOne; $i++) { $value = $arr["audio"]["one"][$i]; echo "$value "; } echo "\n"; $nB = sizeof($arr['video']['B']); for($i = 0; $i < $nB; $i++) { $value = $arr["video"]["B"][$i]; echo "$value "; } ?>
Output:
Case 1 object(stdClass)#1 (2) { ["audio"]=> string(5) "01234" ["video"]=> string(5) "56789" } 01234 56789 Case 2 array(2) { ["audio"]=> string(5) "01234" ["video"]=> string(5) "56789" } 01234 56789 Key: audio; Value: 01234 Key: video; Value: 56789 Case 3 string(123) "{"audio": {"one": 1, "two": 2, "three": 3, "four": 4}, "video": {"one": 1, "two": 2, "three": 3, "four": 4}}" 1 4 Key: one; Value: 1 Key: two; Value: 2 Key: three; Value: 3 Key: four; Value: 4 Key: one; Value: 1 Key: two; Value: 2 Key: three; Value: 3 Key: four; Value: 4 Case 4 string(147) "{"audio": {"one": [1,2,3], "two": [11,22,33], "three": [111,222,333]}, "video": {"A": [1,2,3], "B": [11,22,33], "C": [111,222,333]}}" 1 33 Key: 0; Value: 1 Key: 1; Value: 2 Key: 2; Value: 3 Key: 0; Value: 11 Key: 1; Value: 22 Key: 2; Value: 33 1 2 3 11 22 33
If we want to read the data from a file, we can use the file_get_contents() function:
$json = file_get_contents('data.json'); $data = json_decode($json, true );
json - xml
Here is one of the xml sample files (shown below) from a json file: ismvInfo.json.
<smil xmlns="http://www.w3.org/2001/SMIL20/Language"> <head> <meta name="clientManifestRelativePath" content="bunny.ismc" /> </head> <body> <switch> <video src="bunny_400.ismv" systemBitrate="400074"> <param name="trackID" value="1" valueType="data" /> </video> <video src="bunny_894.ismv" systemBitrate="895227"> <param name="trackID" value="1" valueType="data" /> </video> <video src="bunny_2000.ismv" systemBitrate="2001598"> <param name="trackID" value="1" valueType="data" /> </video> <audio src="bunny_2000.ismv" systemBitrate="127984"> <param name="trackID" value="2" valueType="data" /> </audio> </switch> </body> </smil>
The php code sample:
<?php $json = file_get_contents('./ismvInfo.json'); $data = json_decode($json, true ); var_dump($data); $nVideoStream = sizeof($data['video']['src']); $nAudioStream = sizeof($data['audio']['src']); $base = $data['baseName']; $clientManifest = $base . '.ismc';; $serverManifest = $base . '.ism';; /* construct server manifest array */ $mPlaylist = array( "<?xml version=\"1.0\" encoding=\"utf-8\"?>\n", "<smil xmlns=\"http://www.w3.org/2001/SMIL20/Language\">\n", " <head>\n", " <meta name=\"clientManifestRelativePath\" content=\"$clientManifest\" />\n", " </head>\n", " <body>\n", " <switch>\n" ); for ($i=0; $i < $nVideoStream; $i++) { $src = $data['video']['src'][$i]; $bitrate = $data['video']['bitrate'][$i]; $value = $data['video']['TrackIDvalue'][$i]; $mPlaylist[] = " <video src=\"$src\" systemBitrate=\"$bitrate\">\n"; $mPlaylist[] = " <param name=\"trackID\" value=\"$value\" valueType=\"data\" />\n"; $mPlaylist[] = " </video>\n"; } for ($i=0; $i < $nAudioStream; $i++) { $src = $data['audio']['src'][$i]; $bitrate = $data['audio']['bitrate'][$i]; $value = $data['audio']['TrackIDvalue'][$i]; $mPlaylist[] = " <audio src=\"$src\" systemBitrate=\"$bitrate\">\n"; $mPlaylist[] = " <param name=\"trackID\" value=\"$value\" valueType=\"data\" />\n"; $mPlaylist[] = " </audio>\n"; } $mPlaylist[] = " </switch>\n"; $mPlaylist[] = " </body>\n"; $mPlaylist[] = "</smil>\n"; /* server manifest */ $file = fopen($serverManifest,'w'); foreach($mPlaylist as $mp) { fwrite($file, $mp); } fclose($file); /* construct client manifest array */ $duration = $data['video']['Duration']; $nChunks = $data['video']['Chunks']; $qualityLevels = $data['video']['QualityLevels']; $cPlaylist = array( "<?xml version=\"1.0\" encoding=\"utf-8\"?>\n", "<SmoothStreamingMedia MajorVersion=\"2\" MinorVersion=\"0\" Duration=\"$duration\">\n", " <StreamIndex Type=\"video\" QualityLevels=\"$qualityLevels\" Chunks=\"$nChunks\" Url=\"QualityLevels({bitrate})/Fragments(video={start time})\">\n" ); for ($i=0; $i < $nVideoStream; $i++) { $index = $data['video']['index'][$i]; $bitrate = $data['video']['bitrate'][$i]; $fourCC = $data['video']['fourCC'][$i]; $width = $data['video']['width'][$i]; $height = $data['video']['height'][$i]; $codecPrivateData = $data['video']['codecPrivateData'][$i]; $cPlaylist[] = " <QualityLevel Index=\"$index\" Bitrate=\"$bitrate\" FourCC=\"$fourCC\" MaxWidth=\"$width\" MaxHeight=\"$height\" CodecPrivateData=\"$codecPrivateData\" />\n"; } for ($i=0; $i < $nChunks; $i++) { $fDurations = $data['video']['fragDurations'][$i]; $cPlaylist[] = " <c n=\"$i\" d=\"$fDurations\" />\n"; } $cPlaylist[] = " </StreamIndex>\n"; $qualityLevels = $data['audio']['QualityLevels']; $cPlaylist[] = " <StreamIndex Type=\"audio\" QualityLevels=\"$qualityLevels\" Chunks=\"$nChunks\" Url=\"QualityLevels({bitrate})/Fragments(audio={start time})\">\n"; $nAudioStream = sizeof($data['audio']['src']); $bitrate = $data['audio']['bitrate']; for ($i=0; $i < $nAudioStream; $i++) { $index = $data['audio']['index'][$i]; $bitrate = $data['audio']['bitrate'][$i]; $fourCC = $data['audio']['fourCC'][$i]; $samplingRate = $data['audio']['samplingRate'][$i]; $channels = $data['audio']['channels'][$i]; $bitsPerSample = $data['audio']['bitsPerSample'][$i]; $packetSize = $data['audio']['packetSize'][$i]; $audioTag = $data['audio']['audioTag'][$i]; $codecPrivateData = $data['audio']['codecPrivateData'][$i]; $cPlaylist[] = " <QualityLevel Index=\"$index\" Bitrate=\"$bitrate\" FourCC=\"$fourCC\" SamplingRate=\"$samplingRate\" Channels=\"$channels\" BitsPerSample=\"$bitsPerSample\" PacketSize=\"$packetSize\" AudioTag=\"$audioTag\" CodecPrivateData=\"$codecPrivateData\" />\n"; } for ($i=0; $i < $nChunks; $i++) { $fDurations = $data['audio']['fragDurations'][$i]; $cPlaylist[] = " <c n=\"$i\" d=\"$fDurations\" />\n"; } $cPlaylist[] = " </StreamIndex>\n"; $cPlaylist[] = "</SmoothStreamingMedia>\n"; /* client manifest */ $file = fopen($clientManifest,'w'); foreach($cPlaylist as $cp) { fwrite($file, $cp); } fclose($file); ?>
Here are the xml output files: bunny.ism and bunny.ismc:
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization