PHP Tutorial Laravel 4 Framework (Authentication) - User Account Signin III (Login Authentication)
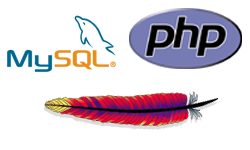
Laravel 4
- Installing on Ubuntu - local
- Installing on a Shared host
- Installing on Windows
- Creating users table
- Home page with controller and blade
- Blade Templating
- Database connection and sending emails
- Creating user account I - GET
- Creating user account II - POST
- Creating user account III - Error checking & redirecting input
- Creating user account IV - User::create()
- Activating user account I - Mail::send()
- Activating user account II - Update user's status
- User account sign-in I - Route and link
- User account sign-in II - Validation
- User account sign-in III - Login Authentication
- Singing out
- Remember the user
- Changing Password I
- Changing Password II
- Recovering forgotten password I
- Recovering forgotten password II
- User Profile
- Database Migration using artisan
This tutorial is the continuation from User account sign-in II - Validation.
Now we have validated user inputs: email and password. In this chapter, we want to authenticate user's login attempt.
In order to get useful debug information, we should add a 'debug' => true to app/config/app.php
In this section, we'll use Auth::attempt() to handle the validated user's login attempt. The Auth::attempt() is to authenticate a user using the given credentials.
AccountController.php file:
class AccountController extends BaseController { ... /* After submitting the sign-in form */ public function postSignIn() { $validator = Validator::make(Input::all(), array( 'email' => 'required|email', 'password' => 'required' ) ); if($validator->fails()) { // Redirect to the sign in page return Redirect::route('account-sign-in') ->withErrors($validator) ->withInput(); // redirect the input } else { $auth = Auth::attempt(array( 'email' => Input::get('email'), 'password' => Input::get('password'), 'active' => 1 )); } if($auth) { // Redirect to the intented page // For example, a user will be redirected to '/ // when the user tried to change password without login' return Redirect::intended('/'); } else { return Redirect::route('account-sign-in') ->with('global', 'Wrong Email/password wrong.'); } return Redirect::route('account-sign-in') ->with('global', 'There is a problem. Have you activated your account?'); } ...
If the user typed in wrong password with correct Email:
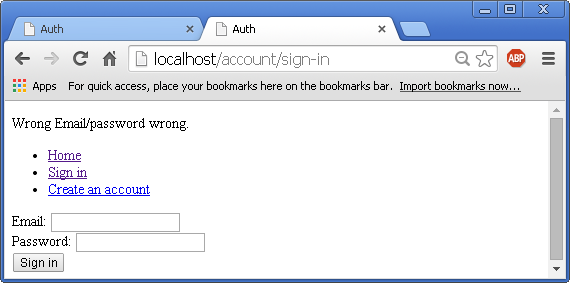
Successful login attempt:
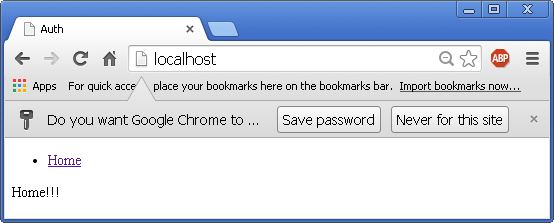
home.blade.php file:
Here we want to say to Hello when a user logged in successfully.
@extends('layout.main') @section('content') @if(Auth::check())Hello, {{ Auth::user()->username }}.
@elseSorry, not signed in.
@endif @stop
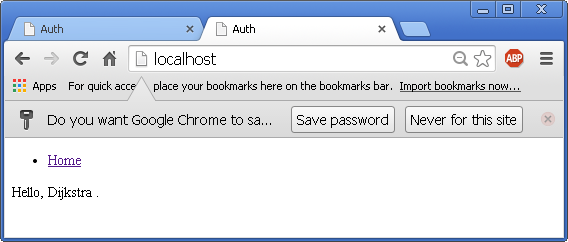
Here is the Laravel authentication project file:
auth.zipLaravel 4
- Installing on Ubuntu - local
- Installing on a Shared host
- Installing on Windows
- Creating users table
- Home page with controller and blade
- Blade Templating
- Database connection and sending emails
- Creating user account I - GET
- Creating user account II - POST
- Creating user account III - Error checking & redirecting input
- Creating user account IV - User::create()
- Activating user account I - Mail::send()
- Activating user account II - Update user's status
- User account sign-in I - Route and link
- User account sign-in II - Validation
- User account sign-in III - Login Authentication
- Singing out
- Remember the user
- Changing Password I
- Changing Password II
- Recovering forgotten password I
- Recovering forgotten password II
- User Profile
- Database Migration using artisan
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization