PHP Tutorial Laravel 4 Framework (Authentication) - Remember Me
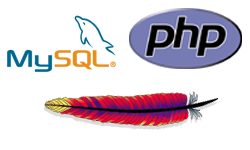
Laravel 4
- Installing on Ubuntu - local
- Installing on a Shared host
- Installing on Windows
- Creating users table
- Home page with controller and blade
- Blade Templating
- Database connection and sending emails
- Creating user account I - GET
- Creating user account II - POST
- Creating user account III - Error checking & redirecting input
- Creating user account IV - User::create()
- Activating user account I - Mail::send()
- Activating user account II - Update user's status
- User account sign-in I - Route and link
- User account sign-in II - Validation
- User account sign-in III - Login Authentication
- Singing out
- Remember the user
- Changing Password I
- Changing Password II
- Recovering forgotten password I
- Recovering forgotten password II
- User Profile
- Database Migration using artisan
This tutorial is the continuation from Singing out.
Now that a user can sign in and sign out, we want to have our app remember the user's information if the user wants.
In this section, we'll use Auth::check() to see if a user is logged in. If so, we want to display a logout link while the user is logged in. We want show a link that the user can logout.
views/layout/navigation.blade.php file:
<nav> <ul> <li> <a href="{{ URL::route('home') }}">Home</a></li> @if(Auth::check()) <li><a href="">Sign out</a></li> @else <li><a href="{{ URL::route('account-sign-in') }}">Sign in</a></li> <li><a href="{{ URL::route('account-create') }}">Create an account</a></li> @endif </ul> </nav>
So, if the user is not logged in, we display the register and login links, otherwise, while the user is logged in, we display a logout link, instead.
Let's see how the link looks like:
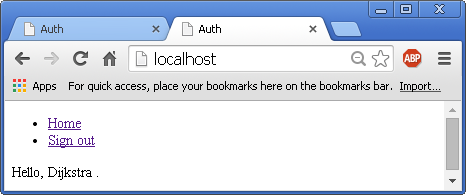
We want to redirect a user not logged in (guest) to a sign in page.
filters.php file:
Route::filter('auth', function() { /* if (Auth::guest()) return Redirect::guest('login'); ==> */ if (Auth::guest()) return Redirect::guest(URL::route('account-sign-in')); });
Now we want to make a group for authenticated users.
routes.php file:
/* Authenticated group */ Route::group(array('before' => 'auth'), function() { /* Sign out (GET) */ Route::get('/account/sign-out', array('as' => 'account-sign-out', 'uses' => 'AccountController@getSignOut' )); }
Note that we wrote only GET method since there is no need for POST for sing out.
AccountController.php file:
/* Authenticated group */ Route::group(array('before' => 'auth'), function() { /* Sign out (GET) */ Route::get('/account/sign-out', array('as' => 'account-sign-out', 'uses' => 'AccountController@getSignOut' )); }
We also need the getSignOut() method. It uses static method Auth::logout() and then redirect back to 'home':
public function getSignOut() { Auth::logout(); return Redirect::route('home'); }
The link to 'home' needs to be make:
navigation.blade.php file:
<nav> <ul> <li> <a href="{{ URL::route('home') }}">Home</a></li> @if(Auth::check()) <li><a href="{{ URL::route('account-sign-out') }}">Sign out</a></li> @else <li><a href="{{ URL::route('account-sign-in') }}">Sign in</a></li> <li><a href="{{ URL::route('account-create') }}">Create an account</a></li> @endif </ul> </nav>
When I try sign out, I got the error, Unknown column 'remember_token':
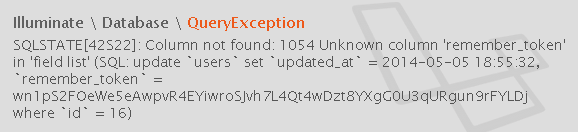
Lalavel doc Upgrading To 4.1.26 From <= 4.1.25 says:
Laravel 4.1.26 introduces security improvements for "remember me" cookies. Before this update, if a remember cookie was hijacked by another malicious user, the cookie would remain valid for a long period of time, even after the true owner of the account reset their password, logged out, etc.
This change requires the addition of a new remember_token column to your users (or equivalent) database table. After this change, a fresh token will be assigned to the user each time they login to your application. The token will also be refreshed when the user logs out of the application. The implications of this change are: if a "remember me" cookie is hijacked, simply logging out of the application will invalidate the cookie.
As discussed in the previous section, it looks like we need another column, remember_token, for our
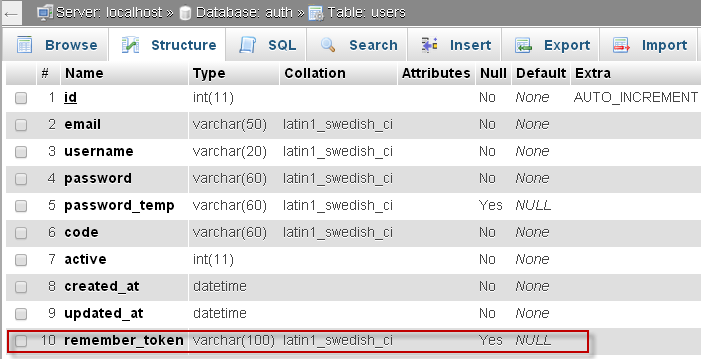
I created a new account:
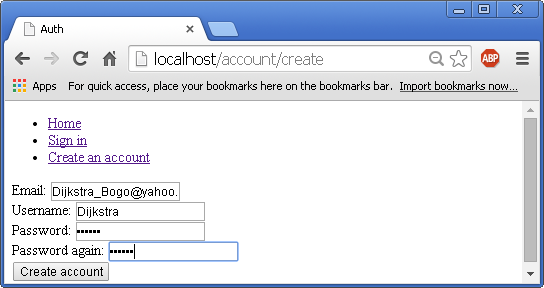
Account activation:
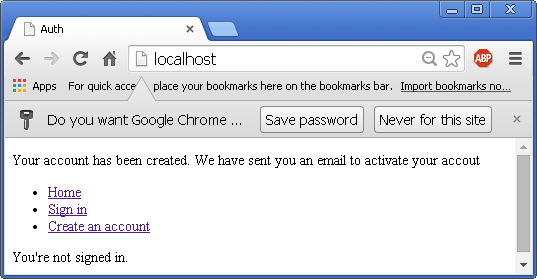
Signing in:
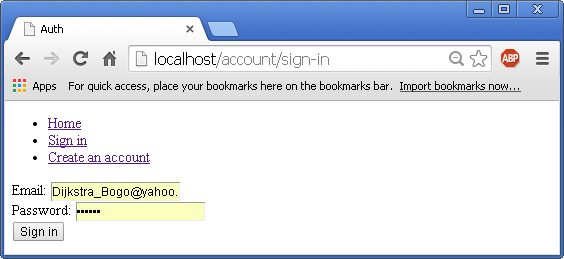
Signed in:
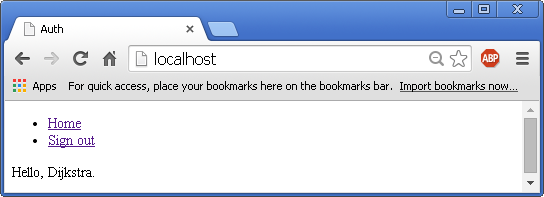
Then, tried to sign out:
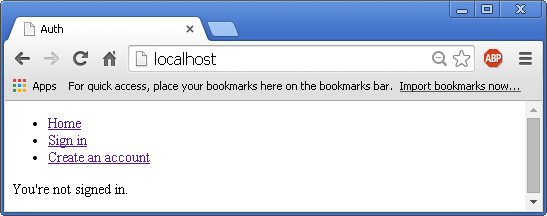
Laravel 4
- Installing on Ubuntu - local
- Installing on a Shared host
- Installing on Windows
- Creating users table
- Home page with controller and blade
- Blade Templating
- Database connection and sending emails
- Creating user account I - GET
- Creating user account II - POST
- Creating user account III - Error checking & redirecting input
- Creating user account IV - User::create()
- Activating user account I - Mail::send()
- Activating user account II - Update user's status
- User account sign-in I - Route and link
- User account sign-in II - Validation
- User account sign-in III - Login Authentication
- Singing out
- Remember the user
- Changing Password I
- Changing Password II
- Recovering forgotten password I
- Recovering forgotten password II
- User Profile
- Database Migration using artisan
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization