PHP Tutorial Laravel 4 Framework (Authentication) - Changing Password II
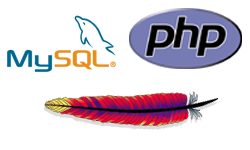
Laravel 4
- Installing on Ubuntu - local
- Installing on a Shared host
- Installing on Windows
- Creating users table
- Home page with controller and blade
- Blade Templating
- Database connection and sending emails
- Creating user account I - GET
- Creating user account II - POST
- Creating user account III - Error checking & redirecting input
- Creating user account IV - User::create()
- Activating user account I - Mail::send()
- Activating user account II - Update user's status
- User account sign-in I - Route and link
- User account sign-in II - Validation
- User account sign-in III - Login Authentication
- Singing out
- Remember the user
- Changing Password I
- Changing Password II
- Recovering forgotten password I
- Recovering forgotten password II
- User Profile
- Database Migration using artisan
This tutorial is the continuation from Changing Password I.
In this chapter, we'll make the forms for the password change and the post actions as well.
In this section, we'll provide forms so that a user can type old password, new password, and confirming password.
views/account/password.blade.php file:
@extends('layout.main') @section('content') <form action="{{ URL::route('account-change-password-post') }}" method="post"> <div class="field"> Old password: <input type="password" name="old_password" > </div> <div class="field"> New password: <input type="password" name="password" > </div> <div class="field"> New password again: <input type="password" name="password_again" > </div> <input type="submit" value="Change password"> {{ Form::token() }} </form> @stop
Let's see how our new forms look like:
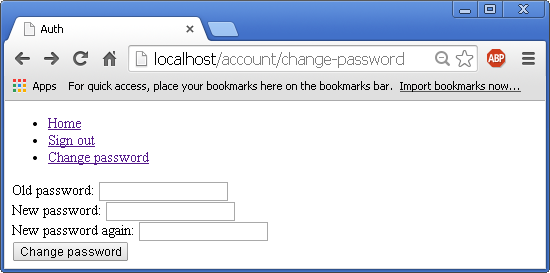
Now we have the forms for the users can fill out. But we want to make sure the inputs are valid ones.
What rules should be applied to the forms?
- Old password should match current password
- New password should be identical to the New password again
- All password should be filled in (all required)
- Password length shouldn't be less than 6 characters
Let's work on AccountController.php file:
public function postChangePassword() { $validator = Validator::make(Input::all(), array( 'password' => 'required', 'old_password' => 'required|min:6', 'password_again'=> 'required|same:password' ) ); if($validator->fails()) { return Redirect::route('account-change-password') ->withErrors($validator); } else { // Change } }
Also we need to implement the error checking in password.blade.php file:
@extends('layout.main') @section('content') <form action="{{ URL::route('account-change-password-post') }}" method="post"> <div class="field"> Old password: <input type="password" name="old_password" > @if($errors->has('old_password')) {{ $errors->first('old_password')}} @endif </div> <div class="field"> New password: <input type="password" name="password" > @if($errors->has('password')) {{ $errors->first('password')}} @endif </div> <div class="field"> New password again: <input type="password" name="password_again" > @if($errors->has('password_again')) {{ $errors->first('password_again')}} @endif </div> <input type="submit" value="Change password"> {{ Form::token() }} </form> @stop
Let's check how the validation works;
With no inputs:
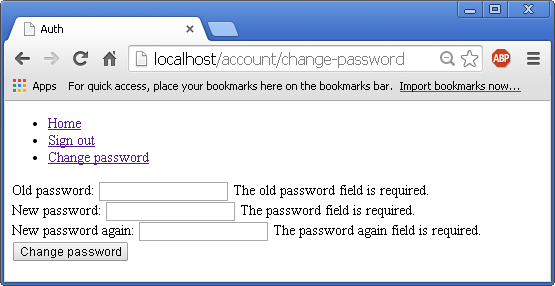
With different new password and new password again:
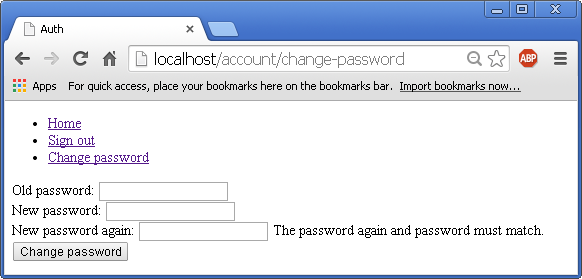
The current password should match the old password that user typed in. We'll put this code into the case after the inputs passed validation in AccountController.php file:
public function postChangePassword() { $validator = Validator::make(Input::all(), array( 'password' => 'required', 'old_password' => 'required|min:6', 'password_again'=> 'required|same:password' ) ); if($validator->fails()) { return Redirect::route('account-change-password') ->withErrors($validator); } else { // passed validation // Grab the current user $user = User::find(Auth::user()->id); // Get passwords from the user's input $old_password = Input::get('old_password'); $password = Input::get('password'); // test input password against the existing one if(Hash::check($old_password, $user->getAuthPassword())){ $user->password = Hash::make($password); // save the new password if($user->save()) { return Redirect::route('home') ->with('global', 'Your password has been changed.'); } } else { return Redirect::route('account-change-password') ->with('global', 'Your old password is incorrect.'); } } /* fall back */ return Redirect::route('account-change-password') ->with('global', 'Your password could not be changed.'); }
Let's test the new code.
With wrong old password:
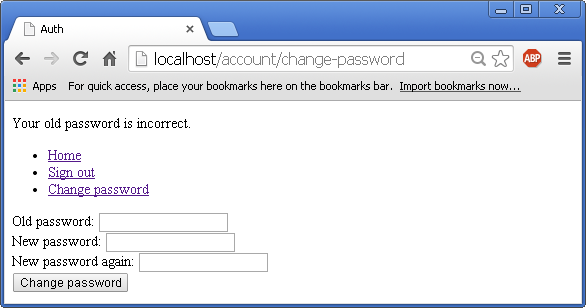
Correct all three input:
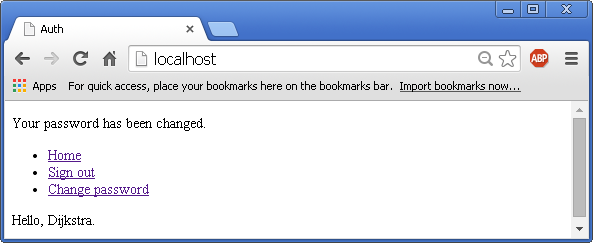
Signed out:
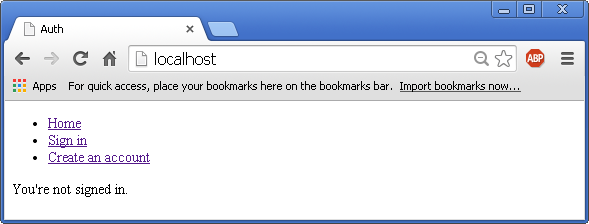
Signing in:
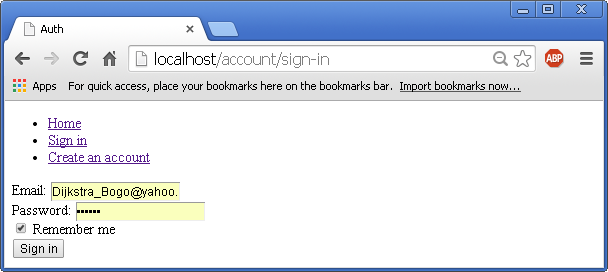
Signed in with the new password:
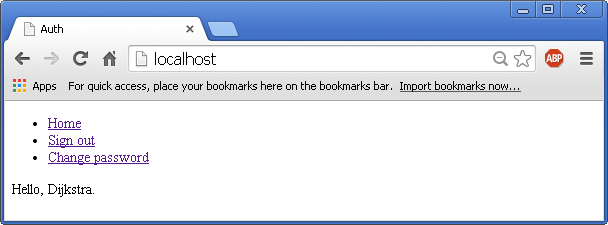
Laravel 4
- Installing on Ubuntu - local
- Installing on a Shared host
- Installing on Windows
- Creating users table
- Home page with controller and blade
- Blade Templating
- Database connection and sending emails
- Creating user account I - GET
- Creating user account II - POST
- Creating user account III - Error checking & redirecting input
- Creating user account IV - User::create()
- Activating user account I - Mail::send()
- Activating user account II - Update user's status
- User account sign-in I - Route and link
- User account sign-in II - Validation
- User account sign-in III - Login Authentication
- Singing out
- Remember the user
- Changing Password I
- Changing Password II
- Recovering forgotten password I
- Recovering forgotten password II
- User Profile
- Database Migration using artisan
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization