PHP & MySQL Tutorial
- Functions
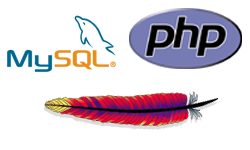
PHP organizes code into functions. Functions allow us to group a block of code together and execute that code by its name. To keep variables in our code separate from variables in functions, PHP provides separate storage of variables within each function. The separate storage space means that the scope is the local storage of the function.
PHP has lots of intrinsic functions such as echo(), but we can create our own using the function keyword.
In the example below, the function has been named draw_line() and it will draw a line when it is called.
<html> <head> <title>Functions</title> </head> <body> <?php function draw_line() { echo "<hr>" ; } ?> <?php draw_line(); ?> <p>PHP produce dynamic web pages.<br /> For this purpose, <br /> PHP code is embedded into the HTML source document <br /> and interpreted by a web server with a PHP processor module, <br /> which generates the web page document.</p> <?php draw_line(); ?> </body> </html>
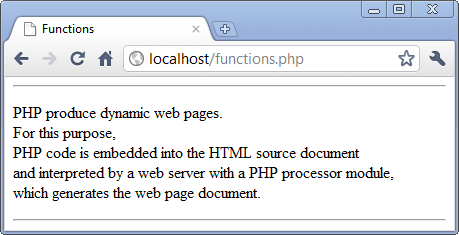
The plain brackets that follow function names can be used to provide data for the code of the function. The data contained within the brackets is the function argument.
In the following example, the function call passes string values to the quotation() functions' $q and $n arguments for quote and name, respectively.
Note that we are using arrays since there are several quotes.
<html> <?php function quotation($q,$n) { echo "<textarea rows=\"3\" cols=\"70\">"; echo "\"$q\""; echo "\n\n \t\t\t\t\t\t-$n"; echo "</textarea>"; } ?> <?php $quote = array(); $name = array(); $quote[0] = "Hardware: The parts of a computer system that can be kicked."; $name[0] = "Jeff Pesis"; $quote[1] = "The question of whether computers can think is like the question of whether submarines can swim."; $name[1] = "Dijkstra"; $quote[2] = "Controlling complexity is the essence of computer programming."; $name[2] = "Kernigan"; $quote[3] = "Java is, in many ways, C++-."; $name[3] = "Feldman"; quotation($quote[0],$name[0]); quotation($quote[1],$name[1]); quotation($quote[2],$name[2]); quotation($quote[3],$name[3]); ?> </html>
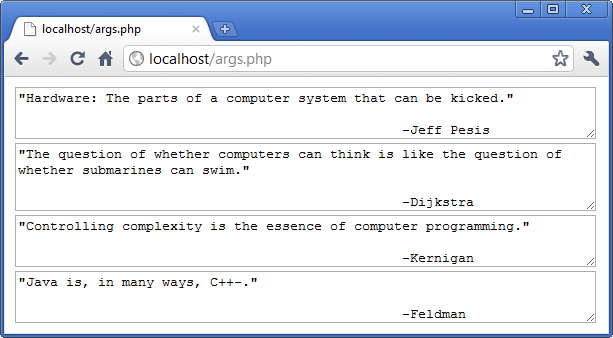
Functions can call other functions. The example below demonstrates the use of multiple functions.
<html> <head> <title>Multiple Functions - Complex</title> </head> <body> <?php function conjugate($r,$i) { echo "conjugate of ($r,$i) is "; write($r,-$i); } function write($r,$i) { echo "($r,$i)"; } conjugate(5,10); ?> </body> </html>
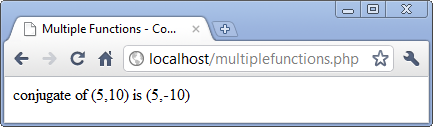
The term scope refers to the places within a code where a certain variable is visible. The scope rules in PHP are:
- Built-in superglobal variables are visible everywhere.
- Constants are always visible globally if they are declared. They can be visible inside and outside functions.
- Global variables declared in a script are visible except inside functions.
- Variable inside functions that are declared as global refer to the global variables of the same name.
- Variables created inside functions and declared as static are invisible from outside the function and they keep their values.
- Variables created inside functions are local to the function and cease to exist when the function ends.
The following example creates a global variable $r and $i. Global variables that are declared outside functions can be accessed by any function in that script.
Unlike other languages that make global variable accessible automatically, functions in PHP is required to declare explicitly that they want to use a global variable. If not declared explicitly, the scope of variable is treated as local variable.
To declare the intention of using the global variable, a function code should use global keyword followed by the variable name. Two functions have access to the global variables.<html> <head> <title>Scope of Variables - Complex Number</title> </head> <body> <?php $r = 20; $i = 10; function conjugate() { global $r,$i; echo "conjugate of ($r,$i) is "; write(); } function write() { global $r,$i; echo "($r,-$i)"; } conjugate(5,10); ?> </body> </html>
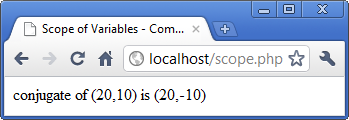
If we comment the global declaration of write() function:
function write() { //global $r,$i; echo "($r,-$i)";
we'll get an error:
conjugate of (20,10) is Notice: Undefined variable: r in C:\Server\www\apache.dev\public_html\test.php on line 17
When a function specifies arguments, all calls to that function should normally pass the correct number of argument values. However, a default value can be assigned to the argument.
In the following example, two arguments are specified, each with a default value to be used if no argument value is passed from the caller:
<?php function sum($i = 11, $j = 12) { $s = $i + $j; echo "$i +$j = $s"; } ?> <html> <head> <title>Defalut Arguments - Multiple Args</title> </head> <body> <?php sum(1,2); ?> <br / > <?php sum(10); ?> </body> </html>
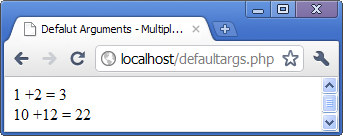
To make our code more reliable, reusable, and readable, we can place our functions in a separate file. PHP provides two statements to load a file into our PHP script: require() and include().
- include()
- require()
- include_once()
- require_once()
The require() and include are pretty similar in their functionality. The only difference between them is the case when they fail, the require() construct gives a fatal error, while the include() gives only a warning. For example, include and include_once() provide a warning if the resource cannot be retrieved and tries to continue execution of the program. The require() and require_once() functions provide stop processing of the particular page if they can't retrieve the resource.
The purpose of require_once() and include_once() constructs is to ensure that an included file can be included only once. Using these constructs protects us from accidentally including the same function library twice. But these are slower than the include() and require().
The include() allows us to include and attach other PHP scripts to our script. Here, we have a function returning the sum of the two arguments:
// sum.php <?php function sum($i, $j) { return $i + $j; } ?>
In another function, we print out the sum by include the sum.php:
// test.php <?php include('sum.php'); echo sum(10, 20); ?>
When executed, this gives:
30
We may have a problem if we include the same scripts because the include statement doesn't check for scripts that have already included:
<?php include('sum.php'); include('sum.php'); echo sum(10, 20); ?>
When executed, we would get the error something like this:
Fatal error: Cannot re-declare sum() (previously declared in C:\Server\www\apache.dev\public_html\sum.php:2) in C:\Server\www\apache.dev\public_html\sum.php on line 4
Replacing the include() with include_once(), we can avoid this type of error:
<?php include_once('sum.php'); include_once('sum.php'); echo sum(10, 20); ?>
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization