PHP & MySQL Tutorial
- Arrays
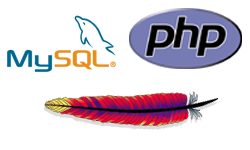
An array is a variable that can contain multiple values.
To work with arrays, we should be familiar with two new terms: elements and indexes.
Elements are the values that are stored in the array.
Each element in the array is referenced by an index that differentiates the element from any other unique element in the array.
Numeric arrays use numbers as their indexed, while associative arrays use strings.
When using associative arrays allow us to add the element,
PHP automatically assigns the first free number, starting at 0.
Both types of arrays allow us to add new elements one at a time.
Associative arrays are useful for storing configuration data because their keys can have a meaningful name.
An ordinary variable is given array status by the array() function.
Then, multiple data values can be assigned to the array elements using array's name.<html> <head> <title>Creating Arrays</title> </head> <body> <?php $script = array(); $script[0] = "ASP"; $script[1] = "ColdFusion Markup Language"; $script[2] = "Java via JavaServer Pages"; $script[3] = "Javascript using Server Side Javascript"; $script[4] = "PHP"; $script[5] = "Perl"; $script[6] = "SMX"; $script[7] = "Python"; $script[8] = "Ruby"; $script[9] = "Lasso"; $script[10] = "WebDNA"; echo "<p><b>Server-side Scripting Languages </b></p><ul>"; foreach ($script as $val ) { echo "<li>$val</li>"; } echo "</ul>"; ?> </body> </html>
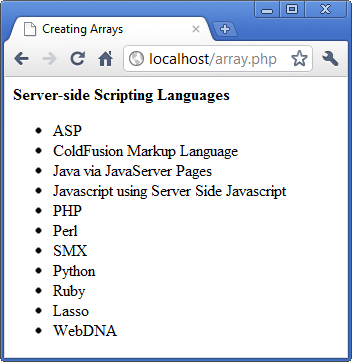
Following example is using the array language construct, a special function.
The array() function allows us to create our array and assign multiple elements all at once.
The array pairs if index keys and values as parameters.
It returns an array that is usually assigned to a variable. The elements to be assigned are separated by commas.
<html> <head><title>Creating Arrays</title></head> <body> <?php $script = array(); $script[0] = "ASP"; $script[1] = "ColdFusion Markup Language"; $script[2] = "Java via JavaServer Pages"; $script[3] = "Javascript using Server Side Javascript"; $script[4] = "PHP"; $script[5] = "Perl"; $script[6] = "SMX"; $script[7] = "Python"; $script[8] = "Ruby"; $script[9] = "Lasso"; $script[10] = "WebDNA"; $extension = array (" ", "cfm", "jsp", "ssjs", "php", "pl", "smx", "py", "rb", "lasso", "dna tpl"); echo "<p><b>Server-side Scripting Languages </b></p><ul>"; for ($i = 0; $i < 11; $i++) { echo "<li>$script[$i] ($extension[$i])</li>"; } echo "</ul>"; ?> </body> </html>
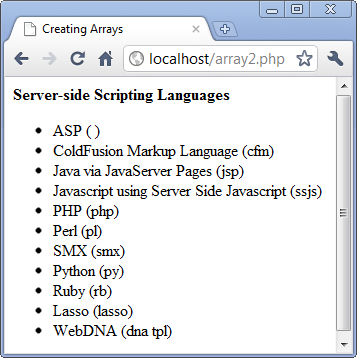
To create associative array, in the following example, we use key and value pairs in two ways.
<html> <head> <title>Associative Arrays</title> </head> <body> <?php $script = array("ASP" => " ", "ColdFusion Markup Language" => "cfm", "Java via JavaServer Pages" =>"jsp", "Javascript using Server Side Javascript" => "ssjs", "PHP" => "php", "Perl" => "pl", "SMX" => "smx"); $script["Python"] = "py"; $script["Ruby"] = "rb"; $script["Lasso"] = "lasso"; $script["WebDNA"] = "dna tpl"; echo "<p><b>Server-side Scripting Languages </b></p><ul>"; foreach ($script as $key => $value) { echo "<li>$key ($value)</li>"; } echo "</ul>"; ?> </body> </html>
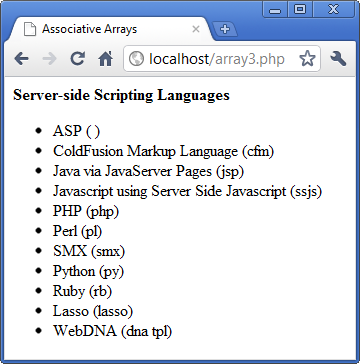
We cab easily create arrays containing sequential numbers of letters by using range() function.
<?php $One_to_Ten = range(1,10); foreach($One_to_Ten as $number) { echo "$number" . " "; } echo "\n"; $A_to_Z = range('A', 'Z'); foreach($A_to_Z as $alphabet) { echo "$alphabet" . " "; } ?>
The output:
1 2 3 4 5 6 7 8 9 10 A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization