PHP Tutorial Laravel 4 Framework (Authentication) - routes, controller, and view for Home Page
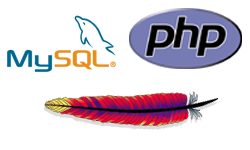
Laravel 4
- Installing on Ubuntu - local
- Installing on a Shared host
- Installing on Windows
- Creating users table
- Home page with controller and blade
- Blade Templating
- Database connection and sending emails
- Creating user account I - GET
- Creating user account II - POST
- Creating user account III - Error checking & redirecting input
- Creating user account IV - User::create()
- Activating user account I - Mail::send()
- Activating user account II - Update user's status
- User account sign-in I - Route and link
- User account sign-in II - Validation
- User account sign-in III - Login Authentication
- Singing out
- Remember the user
- Changing Password I
- Changing Password II
- Recovering forgotten password I
- Recovering forgotten password II
- User Profile
- Database Migration using artisan
The following file has all the updates up to the chapter 22.
Here is the link auth.zip.
This tutorial is the continuation from Creating users table.
In this chapter, we'll see how the Laravel works in terms of the relationships among route, controller, and view. Until this chapter, the path of the displaying our home page has been route -> view , but we're going to use controller in-between them. Also, we'll have a taste of Laravel template which is called blade.
When we does not put anything into the url, just root directory, http://localhost/bogotobogo/Laravel/auth/public/, we get the following Laravel logo:
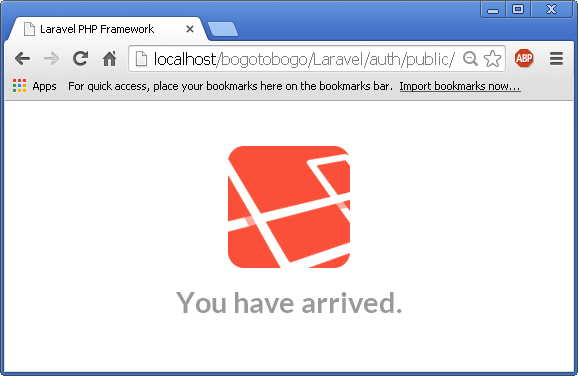
Actually, it hits the get() method in routes.php:
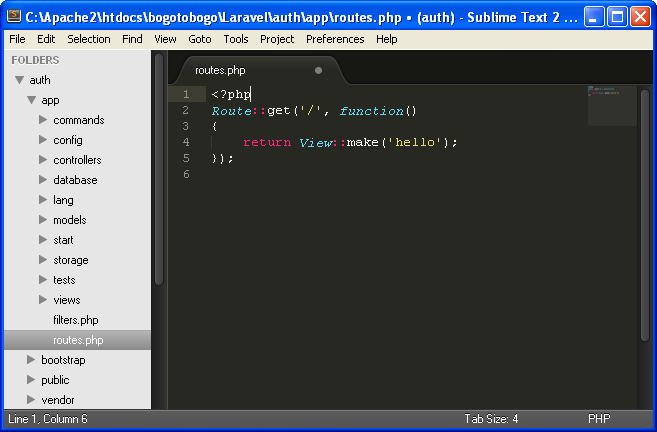
Then, it returns the view from hello.php:
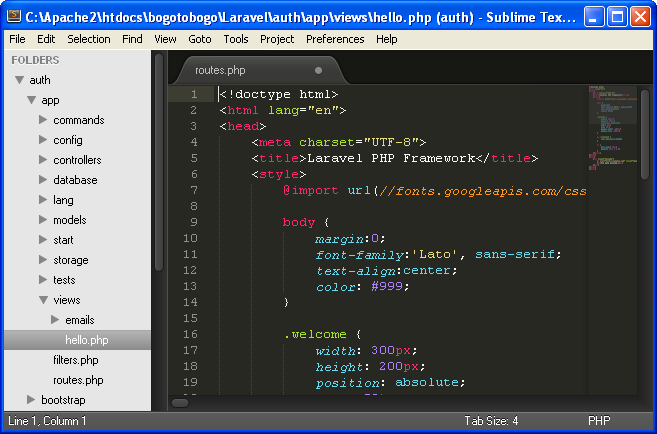
We're going to modify the default path for setting up for view from routes->views to routes->controller->views.
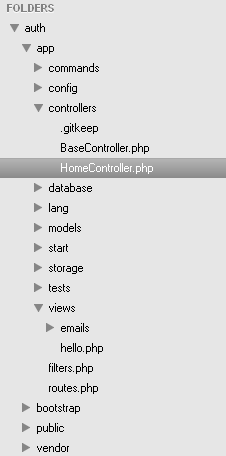
Let's look at the HomeController.php:
<?php class HomeController extends BaseController { public function showWelcome() { return View::make('hello'); } }
We want to remove the routes.php in views (Closure based routes) and use controllers instead.
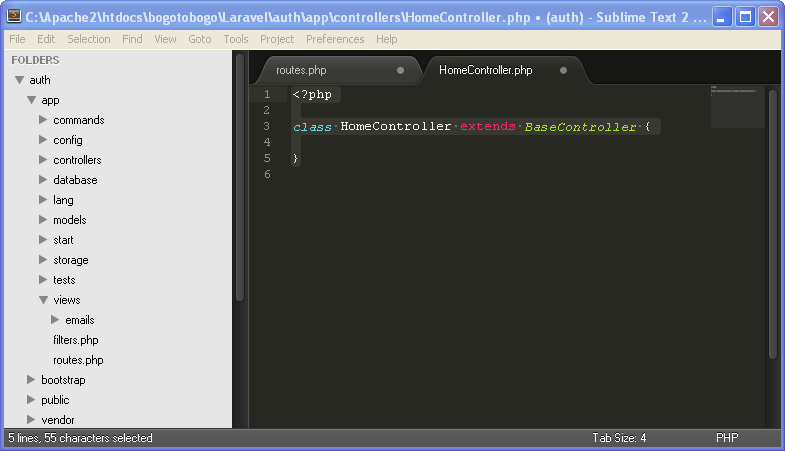
When we refresh the root page, we get this:
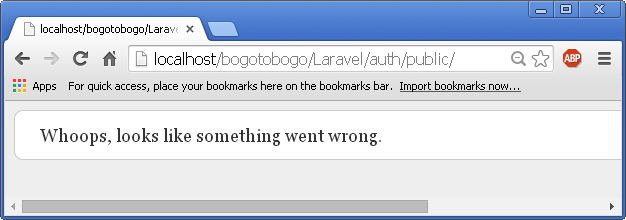
That's because we removed hellp.php from views.
We want to specify the controller in routes.php:
<?php /* Route::get('/', function() { return View::make('hello'); }); */ /* ==> */ Route::get('/', 'HomeController@home');
Here, we're telling Laravel that we'll use 'HomeController' as a controller and the method to use is home.
The home() method looks like this:
/* public function showWelcome() { return View::make('hello'); } */ /* ==> */ public function home() { return 'Home'; }
We're now using controller in-between routes and views. Note that we're not using views yet.
Here is the rendered result:
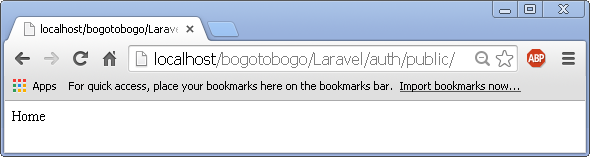
We can use array() like this:
Route::get('/', array('as' => 'home', 'uses' => 'HomeController@home'));
As we know, we haven't made a new view since we removed the view in hello.php in the earlier section. So, now we need to make one: home.blade.php. The 'blade' is a template in Laravel.
Though the blade provides several features, we'll just use the simplest one here:
Home sweet home!
Our new HomeController.php should look like this:
<?php class HomeController extends BaseController { public function home() { return View::make('home'); } }
Our home is now rendered this way:
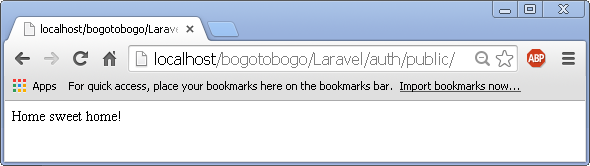
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization