PHP Tutorial Laravel 4 Framework (Authentication) - User Profile Controller
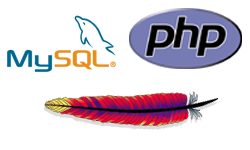
Laravel 4
- Installing on Ubuntu - local
- Installing on a Shared host
- Installing on Windows
- Creating users table
- Home page with controller and blade
- Blade Templating
- Database connection and sending emails
- Creating user account I - GET
- Creating user account II - POST
- Creating user account III - Error checking & redirecting input
- Creating user account IV - User::create()
- Activating user account I - Mail::send()
- Activating user account II - Update user's status
- User account sign-in I - Route and link
- User account sign-in II - Validation
- User account sign-in III - Login Authentication
- Singing out
- Remember the user
- Changing Password I
- Changing Password II
- Recovering forgotten password I
- Recovering forgotten password II
- User Profile
- Database Migration using artisan
We've been using a controller for an account. In this chapter, we'll make another controller for the user profile. We could use the account controller for this, but having another controller and working with it could be a good Laravel practice. .
The URI for user profile is given as /user/username as in the picture below:
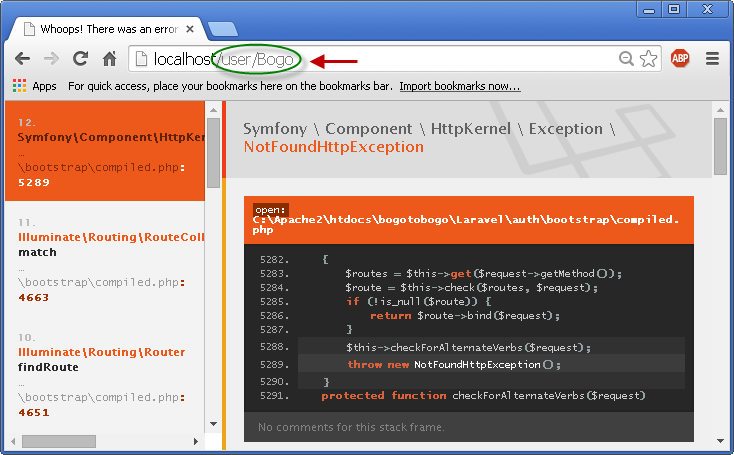
We get NotFoundHttpException since the page is not there yet.
The new route for user profile is defined in views/routes.php file like this
<?php Route::get('/', array('as' => 'home', 'uses' => 'HomeController@home' )); Route::get('/user/{username}', array('as' => 'profile-user', 'uses' => 'ProfileController@user' ));
Let's make a new controller called ProfileController.php under controller folder:
<?php class ProfileController extends BaseController { public function user($username) { return View::make('profile.user'); } }
The view profile.user hasn't been defined yet and that's the next thing we're going to do.
Let's create a new folder called profile under views. Then, user that folder, we'll have a new view file called user.blade.php:
@extends('layout.main') @section('content') User profile. @stop
So, when we type URI /user/username/, it will display the plane text, "User profile."
Here is the data in our database:

The out put looks like this:
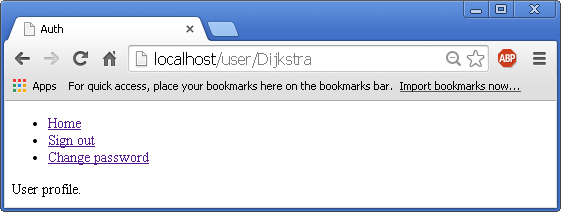
Let's access the database, extract info and display it.
ProfileController.php:
<?php class ProfileController extends BaseController { public function user($username) { $user = User::where('username', '=', $username); if($user->count()) { return View::make('profile.user'); } return App::abort(404); } }
If we refresh the browser, nothing happens because it does not have an error, and the username "Dijkstra" is in the database.

However, if we type a username that's not exists, we get an error:
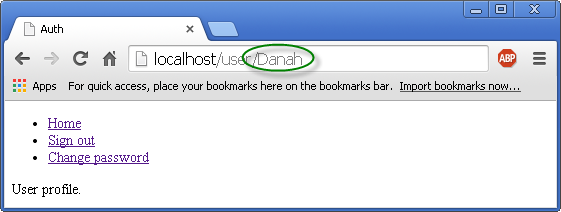
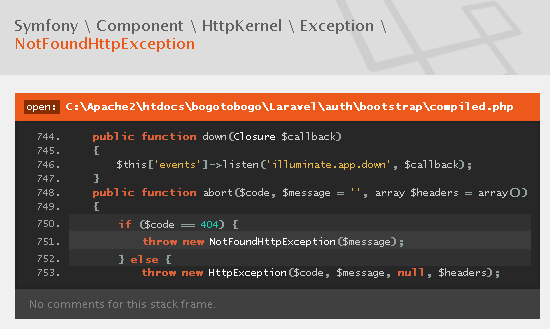
Now, we want to pass user information to the view:
<?php class ProfileController extends BaseController { public function user($username) { $user = User::where('username', '=', $username); if($user->count()) { $user = $user->first(); return View::make('profile.user') ->with('user', $user); } return App::abort(404); } }
We got the $user object passed down to view from ProfileController.php. So, it's time to display it:
@extends('layout.main') @section('content') <p>{{ $user->username }} ( {{ $user->email }} ) </p> @stop
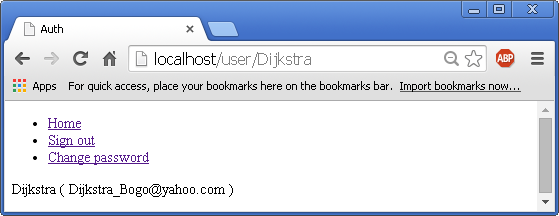
Laravel 4
- Installing on Ubuntu - local
- Installing on a Shared host
- Installing on Windows
- Creating users table
- Home page with controller and blade
- Blade Templating
- Database connection and sending emails
- Creating user account I - GET
- Creating user account II - POST
- Creating user account III - Error checking & redirecting input
- Creating user account IV - User::create()
- Activating user account I - Mail::send()
- Activating user account II - Update user's status
- User account sign-in I - Route and link
- User account sign-in II - Validation
- User account sign-in III - Login Authentication
- Singing out
- Remember the user
- Changing Password I
- Changing Password II
- Recovering forgotten password I
- Recovering forgotten password II
- User Profile
- Database Migration using artisan
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization